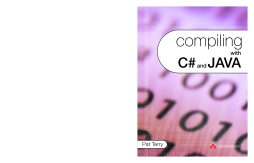
Additional Information
Book Details
Abstract
A compiler is a special program that processes statements in a particular programming language and turns them into machine code that the computer can understand.
Compiling with C# and Java is an introduction to compiler construction using the Java Virtual Machine (JVM) and .NET Common Language Routine (CLR), both of which provide the interface between compiler, C# or Java code, and hardware.
Loaded with exercises, examples and case studies, the text balances theory and practice to provide the reader with a solid working knowledge of the subject.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Compiling with C | i | ||
Contents | v | ||
Preface | xiv | ||
Acknowledgements | xix | ||
Translators and Languages | 1 | ||
Objectives | 1 | ||
Systems programs and translators | 2 | ||
The benefits of using high-level languages | 4 | ||
The curse of complexity | 7 | ||
Successful language design | 8 | ||
The benefits of formal description | 10 | ||
Translator Classification and Structure | 12 | ||
T-diagrams | 13 | ||
Classes of translator | 14 | ||
Linkers and loaders | 16 | ||
Phases in translation | 17 | ||
Multi-stage translators | 25 | ||
Interpreters, interpretive compilers and emulators | 27 | ||
The P-system and the JVM | 30 | ||
JIT compilers and the .NET Framework | 32 | ||
Compiler Development and Bootstrapping | 35 | ||
Using a high-level translator | 35 | ||
Using a high-level host language | 37 | ||
Bootstrapping | 37 | ||
Self-compiling compilers | 38 | ||
The half bootstrap | 39 | ||
Bootstrapping from a portable interpretive compiler | 40 | ||
A P-code assembler | 42 | ||
The use of compiler generating tools | 43 | ||
Stack Machines | 44 | ||
Simple machine architecture | 44 | ||
Assembler languages | 47 | ||
Addressing modes | 48 | ||
The PVM – a simple stack machine | 51 | ||
Programming the PVM | 56 | ||
An emulator for the PVM | 61 | ||
A minimal assembler for PVM code | 66 | ||
Enhancing the efficiency of the emulator | 69 | ||
Error handling in the PVM | 70 | ||
Enhancing the instruction set of the PVM | 75 | ||
Another stack machine – the JVM | 80 | ||
The CLR – a conceptual stack machine | 82 | ||
Language Specification | 84 | ||
Syntax, semantics and pragmatics | 84 | ||
Languages, symbols, alphabets and strings | 86 | ||
Regular expressions | 87 | ||
Grammars and productions | 91 | ||
Classic BNF notation for productions | 95 | ||
Simple examples | 96 | ||
Phrase structure and lexical structure | 101 | ||
e-productions | 101 | ||
Extensions to BNF | 102 | ||
Syntax diagrams | 116 | ||
Formal treatment of semantics | 117 | ||
Development and Classification of Grammars | 123 | ||
Equivalent grammars | 123 | ||
Case study – equivalent grammars for describing expressions | 124 | ||
Some simple restrictions on grammars | 129 | ||
Ambiguous grammars | 131 | ||
The Chomsky hierarchy | 135 | ||
Parser and Scanner Construction | 172 | ||
Construction of simple recursivev descent parsers | 172 | ||
Case study – a parser for assignment sequences | 175 | ||
Other aspects of recursive descent parsing | 179 | ||
Syntax error detection and recovery | 185 | ||
Construction of simple scanners | 194 | ||
Case study – a scanner for Boolean assignments | 195 | ||
Keywords and literals | 204 | ||
Comment handling | 208 | ||
LR parsing | 211 | ||
Automated construction of scanners and parsers | 216 | ||
Syntax-directed Translation | 219 | ||
Embedding semantic actions into syntax rules | 219 | ||
Attribute grammars | 223 | ||
Synthesized and inherited attributes | 227 | ||
Classes of attribute grammars | 232 | ||
Case study – a small database for a group of students | 233 | ||
Using Coco/R: Overview | 238 | ||
Coco/R – a brief history | 238 | ||
Installing and running Coco/R | 239 | ||
Case study – a simple adding machine | 242 | ||
Overall form of a Cocol description | 243 | ||
Scanner specification | 244 | ||
Parser specification | 251 | ||
The driver program | 267 | ||
Using Coco/R: Case Studies | 270 | ||
Case study – understanding C declarations | 270 | ||
Case study – generating simple two-address code from expressions | 273 | ||
Case study – generating two-address code from an AST | 277 | ||
Case study – manipulating EBNF productions | 284 | ||
Case study – a simple assembler for the PVM | 287 | ||
Further projects | 295 | ||
A Parva Compiler: the Front End | 307 | ||
Overall compiler structure | 308 | ||
File handling | 309 | ||
Error reporting | 310 | ||
Scanning and parsing | 310 | ||
Syntax error recovery | 311 | ||
Constraint analysis | 311 | ||
Further projects | 326 | ||
A Parva Compiler: the Back End | 343 | ||
Extending the symbol table entries | 344 | ||
The code generation interface | 347 | ||
Using the code generation interface | 349 | ||
Code generation for the PVM | 359 | ||
Towards greater efficiency | 363 | ||
Other aspects of code generation | 372 | ||
Further projects | 380 | ||
A Parva Compiler: Functions and Parameters | 391 | ||
Source language extensions | 391 | ||
Constraint analysis | 395 | ||
Run-time storage management | 398 | ||
Putting it all together | 407 | ||
Mutually recursive functions | 419 | ||
Nested functions | 420 | ||
Heap management | 433 | ||
Further projects | 438 | ||
A C | 445 | ||
The source language – C | 446 | ||
The target language | 446 | ||
The symbol table | 457 | ||
Abstract syntax design | 467 | ||
Abstract syntax tree construction | 475 | ||
Parsing declarations | 489 | ||
Further projects | 500 | ||
A C | 502 | ||
Single-pass compiler strategy | 503 | ||
Generating code for statements from an abstract syntax tree | 504 | ||
Generating code for expressionsf rom an abstract syntax tree | 515 | ||
Low-level code generation – the CodeGen class | 533 | ||
The OpCode class | 536 | ||
Tracking the depth of stack | 537 | ||
Generating the directives | 541 | ||
Further projects | 544 | ||
Appendix A: Assembler programmer’s guide to the PVM, JVM and CLR virtual machines | 549 | ||
Fundamental types | 549 | ||
Instruction sets | 551 | ||
Assembler directives | 568 | ||
Appendix B: Library routines | 572 | ||
Appendix C: Context-free grammars and I/O facilities for Parva and C | 578 | ||
Appendix D: Software resources for this book | 584 | ||
The Resource Kit | 584 | ||
Coco/R distributions | 585 | ||
Other compiler tools, free compilers and similar resources | 586 | ||
Disclaimer | 588 | ||
Bibliography | 590 | ||
Index | 596 |