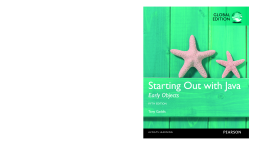
Additional Information
Book Details
Abstract
This text is intended for use in the Java programming course
Tony Gaddis’s accessible, step-by-step presentation helps beginning students understand the important details necessary to become skilled programmers at an introductory level. Gaddis motivates the study of both programming skills and the Java programming language by presenting all the details needed to understand the “how” and the “why”—but never losing sight of the fact that most beginners struggle with this material. His approach is both gradual and highly accessible, ensuring that students understand the logic behind developing high-quality programs.
In Starting Out with Java: Early Objects, Gaddis looks at objects—the fundamentals of classes and methods—before covering procedural programming. As with all Gaddis texts, clear and easy-to-read code listings, concise and practical real-world examples, and an abundance of exercises appear in every chapter.
Teaching and Learning Experience
This program presents a better teaching and learning experience—for you and your students.
-
Enhance Learning with the Gaddis Approach: Gaddis’s accessible approach features clear and easy-to-read code listings, concise real-world examples, and exercises in every chapter.
-
Keep Your Course Current: Content is refreshed to provide the most up-to-date information on new technologies for your course.
-
Support Instructors and Students: Student and instructor resources are available to expand on the topics presented in the text.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Title | Title | ||
Copyright | Copyright | ||
Contents | 9 | ||
Preface | 15 | ||
Chapter 1 Introduction to Computers and Java | 23 | ||
1.1 Introduction | 23 | ||
1.2 Why Program? | 23 | ||
1.3 Computer Systems: Hardware and Software | 24 | ||
1.4 Programming Languages | 28 | ||
1.5 What Is a Program Made of? | 31 | ||
1.6 The Programming Process | 38 | ||
1.7 Object-Oriented Programming | 41 | ||
Review Questions and Exercises | 46 | ||
Programming Challenge | 50 | ||
Chapter 2 Java Fundamentals | 53 | ||
2.1 The Parts of a Java Program | 53 | ||
2.2 The System.out.print and System.out.print ln Methods, and the Java API | 59 | ||
2.3 Variables and Literals | 65 | ||
2.4 Primitive Data Types | 71 | ||
2.5 Arithmetic Operators | 82 | ||
2.6 Combined Assignment Operators | 91 | ||
2.7 Conversion between Primitive Data Types | 92 | ||
2.8 Creating Named Constants with final | 96 | ||
2.9 The String Class | 98 | ||
2.10 Scope | 103 | ||
2.11 Comments | 105 | ||
2.12 Programming Style | 109 | ||
2.13 Reading Keyboard Input | 111 | ||
2.14 Dialog Boxes | 119 | ||
2.15 The System.out.printf Method | 126 | ||
2.16 Common Errors to Avoid | 138 | ||
Review Questions and Exercises | 140 | ||
Programming Challenges | 145 | ||
Chapter 3 A First Look at Classes and Objects | 151 | ||
3.1 Classes | 151 | ||
3.2 More about Passing Arguments | 171 | ||
3.3 Instance Fields and Methods | 174 | ||
3.4 Constructors | 177 | ||
3.5 A Bank Account Class | 183 | ||
3.6 Classes, Variables, and Scope | 194 | ||
3.7 Packages and import Statements | 195 | ||
3.8 Focus on Object-Oriented Design: Finding the Classes and Their Responsibilities | 196 | ||
3.9 Common Errors to Avoid | 205 | ||
Review Questions and Exercises | 205 | ||
Programming Challenges | 209 | ||
Chapter 4 Decision Structures | 215 | ||
4.1 The if Statement | 215 | ||
4.2 The if-else Statement | 224 | ||
4.3 The Payroll Class | 227 | ||
4.4 Nested if Statements | 231 | ||
4.5 The if-else-if Statement | 239 | ||
4.6 Logical Operators | 244 | ||
4.7 Comparing String Objects | 252 | ||
4.8 More about Variable Declaration and Scope | 257 | ||
4.9 The Conditional Operator (Optional) | 259 | ||
4.10 The switch Statement | 260 | ||
4.11 Formatting Numbers with the Decimal Format Class | 270 | ||
4.12 Focus on Problem Solving: The Sales Commission Class | 276 | ||
4.13 Generating Random Numbers with the Random Class | 283 | ||
4.14 Common Errors to Avoid | 289 | ||
Review Questions and Exercises | 290 | ||
Programming Challenges | 295 | ||
Chapter 5 Loops and Files | 301 | ||
5.1 The Increment and Decrement Operators | 301 | ||
5.2 The while Loop | 305 | ||
5.3 Using the while Loop for Input Validation | 312 | ||
5.4 The do-while Loop | 315 | ||
5.5 The for Loop | 318 | ||
5.6 Running Totals and Sentinel Values | 329 | ||
5.7 Nested Loops | 334 | ||
5.8 The break and continue Statements | 342 | ||
5.9 Deciding Which Loop to Use | 342 | ||
5.10 Introduction to File Input and Output | 343 | ||
5.11 Common Errors to Avoid | 363 | ||
Review Questions and Exercises | 364 | ||
Programming Challenges | 370 | ||
Chapter 6 A Second Look at Classes and Objects | 379 | ||
6.1 Static Class Members | 379 | ||
6.2 Overloaded Methods | 386 | ||
6.3 Overloaded Constructors | 391 | ||
6.4 Passing Objects as Arguments to Methods | 398 | ||
6.5 Returning Objects from Methods | 411 | ||
6.6 The toString Method | 414 | ||
6.7 Writing an equals Method | 418 | ||
6.8 Methods That Copy Objects | 420 | ||
6.9 Aggregation | 423 | ||
6.10 The this Reference Variable | 436 | ||
6.11 Inner Classes | 439 | ||
6.12 Enumerated Types | 442 | ||
6.13 Garbage Collection | 451 | ||
6.14 Focus on Object-Oriented Design: Class Collaboration | 453 | ||
6.15 Common Errors to Avoid | 457 | ||
Review Questions and Exercises | 458 | ||
Programming Challenges | 463 | ||
Chapter 7 Arrays and the ArrayList Class | 471 | ||
7.1 Introduction to Arrays | 471 | ||
7.2 Processing Array Contents | 482 | ||
7.3 Passing Arrays as Arguments to Methods | 494 | ||
7.4 Some Useful Array Algorithms and Operations | 498 | ||
7.5 Returning Arrays from Methods | 510 | ||
7.6 String Arrays | 512 | ||
7.7 Arrays of Objects | 516 | ||
7.8 The Sequential Search Algorithm | 520 | ||
7.9 The Selection Sort and the Binary Search Algorithms | 523 | ||
7.10 Two-Dimensional Arrays | 531 | ||
7.11 Arrays with Three or More Dimensions | 543 | ||
7.12 Command-Line Arguments and Variable-Length Argument Lists | 544 | ||
7.13 The ArrayList Class | 548 | ||
7.14 Common Errors to Avoid | 556 | ||
Review Questions and Exercises | 557 | ||
Programming Challenges | 561 | ||
Chapter 8 Text Processing and Wrapper Classes | 569 | ||
8.1 Introduction to Wrapper Classes | 569 | ||
8.2 Character Testing and Conversion with the Character Class | 570 | ||
8.3 More about String Objects | 577 | ||
8.4 The StringBuilder Class | 591 | ||
8.5 Tokenizing Strings | 600 | ||
8.6 Wrapper Classes for the Numeric Data Types | 609 | ||
8.7 Focus on Problem Solving: The TestScoreReader Class | 613 | ||
8.8 Common Errors to Avoid | 617 | ||
Review Questions and Exercises | 617 | ||
Programming Challenges | 621 | ||
Chapter 9 Inheritance | 627 | ||
9.1 What Is Inheritance? | 627 | ||
9.2 Calling the Superclass Constructor | 638 | ||
9.3 Overriding Superclass Methods | 646 | ||
9.4 Protected Members | 654 | ||
9.5 Classes That Inherit from Subclasses | 661 | ||
9.6 The Object Class | 666 | ||
9.7 Polymorphism | 668 | ||
9.8 Abstract Classes and Abstract Methods | 673 | ||
9.9 Interfaces | 679 | ||
9.10 Common Errors to Avoid | 690 | ||
Review Questions and Exercises | 691 | ||
Programming Challenges | 697 | ||
Chapter 10 Exceptions and Advanced File I/O | 703 | ||
10.1 Handling Exceptions | 703 | ||
10.2 Throwing Exceptions | 726 | ||
10.3 Advanced Topics: Binary Files, Random Access Files, and Object Serialization | 734 | ||
10.4 Common Errors to Avoid | 750 | ||
Review Questions and Exercises | 751 | ||
Programming Challenges | 757 | ||
Chapter 11 GUI Applications—Part 1 | 761 | ||
11.1 Introduction | 761 | ||
11.2 Dialog Boxes | 764 | ||
11.3 Creating Windows | 775 | ||
11.4 Layout Managers | 802 | ||
11.5 Radio Buttons and Check Boxes | 819 | ||
11.6 Borders | 832 | ||
11.7 Focus on Problem Solving: Extending the JPanel Class | 834 | ||
11.8 Splash Screens | 848 | ||
11.9 Using Console Output to Debug a GUI Application | 849 | ||
11.10 Common Errors to Avoid | 853 | ||
Review Questions and Exercises | 853 | ||
Programming Challenges | 858 | ||
Chapter 12 GUI Applications—Part 2 | 863 | ||
12.1 Read-Only Text Fields | 863 | ||
12.2 Lists | 864 | ||
12.3 Combo Boxes | 881 | ||
12.4 Displaying Images in Labels and Buttons | 887 | ||
12.5 Mnemonics and Tool Tips | 893 | ||
12.6 File Choosers and Color Choosers | 895 | ||
12.7 Menus | 899 | ||
12.8 More about Text Components: Text Areas and Fonts | 908 | ||
12.9 Sliders | 913 | ||
12.10 Look and Feel | 917 | ||
12.11 Common Errors to Avoid | 919 | ||
Review Questions and Exercises | 920 | ||
Programming Challenges | 925 | ||
Chapter 13 Applets and More | 931 | ||
13.1 Introduction to Applets | 931 | ||
13.2 A Brief Introduction to HTML | 933 | ||
13.3 Creating Applets with Swing | 943 | ||
13.4 Using AWT for Portability | 951 | ||
13.5 Drawing Shapes | 956 | ||
13.6 Handling Mouse Events | 976 | ||
13.7 Timer Objects | 987 | ||
13.8 Playing Audio | 991 | ||
13.9 Common Errors to Avoid | 996 | ||
Review Questions and Exercises | 996 | ||
Programming Challenges | 1002 | ||
Chapter 14 Creating GUI Applications with JavaFX | 1005 | ||
14.1 Introduction | 1005 | ||
14.2 Scene Graphs | 1007 | ||
14.3 Using Scene Builder to Create JavaFX Applications | 1009 | ||
14.4 Writing the Application Code | 1023 | ||
14.5 RadioButtons and CheckBoxes | 1033 | ||
14.6 Displaying Images | 1047 | ||
14.7 Common Errors to Avoid | 1052 | ||
Review Questions and Exercises | 1052 | ||
Programming Challenges | 1056 | ||
Chapter 15 Recursion | 1061 | ||
15.1 Introduction to Recursion | 1061 | ||
15.2 Solving Problems with Recursion | 1064 | ||
15.3 Examples of Recursive Methods | 1068 | ||
15.4 A Recursive Binary Search Method | 1075 | ||
15.5 The Towers of Hanoi | 1078 | ||
15.6 Common Errors to Avoid | 1082 | ||
Review Questions and Exercises | 1083 | ||
Programming Challenges | 1086 | ||
Chapter 16 Databases | 1089 | ||
16.1 Introduction to Database Management Systems | 1089 | ||
16.2 Tables, Rows, and Columns | 1095 | ||
16.3 Introduction to the SQL SELECT Statement | 1099 | ||
16.4 Inserting Rows | 1120 | ||
16.5 Updating and Deleting Existing Rows | 1123 | ||
16.6 Creating and Deleting Tables | 1132 | ||
16.7 Creating a New Database with JDBC | 1136 | ||
16.8 Scrollable Result Sets | 1137 | ||
16.9 Result Set Meta Data | 1139 | ||
16.10 Displaying Query Results in a JTable | 1142 | ||
16.11 Relational Data | 1153 | ||
16.12 Advanced Topics | 1174 | ||
16.13 Common Errors to Avoid | 1176 | ||
Review Questions and Exercises | 1177 | ||
Programming Challenges | 1182 | ||
Appendix A Getting Started with Alice 2 | 1185 | ||
Index | 1211 | ||
Symbols | 1211 | ||
A | 1211 | ||
B | 1212 | ||
C | 1213 | ||
D | 1214 | ||
E | 1215 | ||
F | 1215 | ||
G | 1216 | ||
H | 1216 | ||
I | 1217 | ||
J | 1217 | ||
K | 1219 | ||
L | 1219 | ||
M | 1219 | ||
N | 1220 | ||
O | 1220 | ||
P | 1221 | ||
Q | 1221 | ||
R | 1221 | ||
S | 1222 | ||
T | 1224 | ||
U | 1224 | ||
V | 1225 | ||
W | 1225 | ||
X | 1225 | ||
Credits | 1227 |