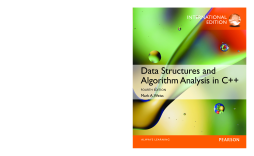
Additional Information
Book Details
Abstract
Data Structures and Algorithm Analysis in C++ is an advanced algorithms book that bridges the gap between traditional CS2 and Algorithms Analysis courses.
As the speed and power of computers increases, so does the need for effective programming and algorithm analysis. By approaching these skills in tandem, Mark Allen Weiss teaches readers to develop well-constructed, maximally efficient programs using the C++ programming language.
This book explains topics from binary heaps to sorting to NP-completeness, and dedicates a full chapter to amortized analysis and advanced data structures and their implementation. Figures and examples illustrating successive stages of algorithms contribute to Weiss’ careful, rigorous and in-depth analysis of each type of algorithm.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Title | Title | ||
Contents\r | 7 | ||
Preface | 15 | ||
Chapter 1 Programming: A General Overview | 19 | ||
1.1 What’s This Book About? | 19 | ||
1.2 Mathematics Review | 20 | ||
1.2.1 Exponents | 21 | ||
1.2.2 Logarithms | 21 | ||
1.2.3 Series | 22 | ||
1.2.4 Modular Arithmetic | 23 | ||
1.2.5 The P Word | 24 | ||
1.3 A Brief Introduction to Recursion | 26 | ||
1.4 C++ Classes | 30 | ||
1.4.1 Basic class Syntax | 30 | ||
1.4.2 Extra Constructor Syntax and Accessors | 31 | ||
1.4.3 Separation of Interface and Implementation | 34 | ||
1.4.4 vector and string | 37 | ||
1.5 C++ Details | 39 | ||
1.5.1 Pointers | 39 | ||
1.5.2 Lvalues, Rvalues, and References | 41 | ||
1.5.3 Parameter Passing | 43 | ||
1.5.4 Return Passing | 45 | ||
1.5.5 std::swap and std::move | 47 | ||
1.5.6 The Big-Five: Destructor, Copy Constructor, Move Constructor, CopyAssignment operator=, Move Assignment operator= | 48 | ||
1.5.7 C-style Arrays and Strings | 53 | ||
1.6 Templates | 54 | ||
1.6.1 Function Templates | 55 | ||
1.6.2 Class Templates | 56 | ||
1.6.3 Object, Comparable, and an Example | 57 | ||
1.6.4 Function Objects | 59 | ||
1.6.5 Separate Compilation of Class Templates | 62 | ||
1.7 Using Matrices | 62 | ||
1.7.1 The Data Members, Constructor, and Basic Accessors | 62 | ||
1.7.2 operator[] | 63 | ||
1.7.3 Big-Five | 64 | ||
Summary | 64 | ||
Exercises | 64 | ||
References | 66 | ||
Chapter 2 Algorithm Analysis | 69 | ||
2.1 Mathematical Background | 69 | ||
2.2 Model | 72 | ||
2.3 What to Analyze | 72 | ||
2.4 Running-Time Calculations | 75 | ||
2.4.1 A Simple Example | 76 | ||
2.4.2 General Rules | 76 | ||
2.4.3 Solutions for the Maximum SubsequenceSum Problem | 78 | ||
2.4.4 Logarithms in the Running Time | 84 | ||
2.4.5 Limitations of Worst-Case Analysis | 88 | ||
Summary | 88 | ||
Exercises | 89 | ||
References | 94 | ||
Chapter 3 Lists, Stacks, and Queues | 95 | ||
3.1 Abstract Data Types (ADTs) | 95 | ||
3.2 The List ADT | 96 | ||
3.2.1 Simple Array Implementation of Lists | 96 | ||
3.2.2 Simple Linked Lists | 97 | ||
3.3 vector and list in the STL | 98 | ||
3.3.1 Iterators | 100 | ||
3.3.2 Example: Using erase on a List | 101 | ||
3.3.3 const_iterators | 102 | ||
3.4 Implementation of vector | 104 | ||
3.5 Implementation of list | 109 | ||
3.6 The Stack ADT | 121 | ||
3.6.1 Stack Model | 121 | ||
3.6.2 Implementation of Stacks | 122 | ||
3.6.3 Applications | 122 | ||
3.7 The Queue ADT | 130 | ||
3.7.1 Queue Model | 131 | ||
3.7.2 Array Implementation of Queues | 131 | ||
3.7.3 Applications of Queues | 133 | ||
Summary | 134 | ||
Exercises | 134 | ||
Chapter 4 Trees | 139 | ||
4.1 Preliminaries | 139 | ||
4.1.1 Implementation of Trees | 140 | ||
4.1.2 Tree Traversals with an Application | 141 | ||
4.2 Binary Trees | 144 | ||
4.2.1 Implementation | 146 | ||
4.2.2 An Example: Expression Trees | 146 | ||
4.3 The Search Tree ADT—Binary Search Trees | 150 | ||
4.3.1 contains | 152 | ||
4.3.2 findMin and findMax | 153 | ||
4.3.3 insert | 154 | ||
4.3.4 remove | 157 | ||
4.3.5 Destructor and Copy Constructor | 159 | ||
4.3.6 Average-Case Analysis | 159 | ||
4.4 AVL Trees | 162 | ||
4.4.1 Single Rotation | 165 | ||
4.4.2 Double Rotation | 167 | ||
4.5 Splay Trees | 176 | ||
4.5.1 A Simple Idea (That Does Not Work) | 176 | ||
4.5.2 Splaying | 178 | ||
4.6 Tree Traversals (Revisited) | 184 | ||
4.7 B-Trees | 186 | ||
4.8 Sets and Maps in the Standard Library | 191 | ||
4.8.1 Sets | 191 | ||
4.8.2 Maps | 192 | ||
4.8.3 Implementation of set and map | 193 | ||
4.8.4 An Example That Uses Several Maps | 194 | ||
Summary | 199 | ||
Exercises | 200 | ||
References | 207 | ||
Chapter 5 Hashing | 211 | ||
5.1 General Idea | 211 | ||
5.2 Hash Function | 212 | ||
5.3 Separate Chaining | 214 | ||
5.4 Hash Tables without Linked Lists | 219 | ||
5.4.1 Linear Probing | 219 | ||
5.4.2 Quadratic Probing | 220 | ||
5.4.3 Double Hashing | 225 | ||
5.5 Rehashing | 226 | ||
5.6 Hash Tables in the Standard Library | 228 | ||
5.7 Hash Tables with Worst-Case O(1) Access | 230 | ||
5.7.1 Perfect Hashing | 231 | ||
5.7.2 Cuckoo Hashing | 233 | ||
5.7.3 Hopscotch Hashing | 245 | ||
5.8 Universal Hashing | 248 | ||
5.9 Extendible Hashing | 251 | ||
Summary | 254 | ||
Exercises | 255 | ||
References | 259 | ||
Chapter 6 Priority Queues (Heaps) | 263 | ||
6.1 Model | 263 | ||
6.2 Simple Implementations | 264 | ||
6.3 Binary Heap | 265 | ||
6.3.1 Structure Property | 265 | ||
6.3.2 Heap-Order Property | 266 | ||
6.3.3 Basic Heap Operations | 267 | ||
6.3.4 Other Heap Operations | 270 | ||
6.4 Applications of Priority Queues | 275 | ||
6.4.1 The Selection Problem | 276 | ||
6.4.2 Event Simulation | 277 | ||
6.5 d-Heaps | 278 | ||
6.6 Leftist Heaps | 279 | ||
6.6.1 Leftist Heap Property | 279 | ||
6.6.2 Leftist Heap Operations | 280 | ||
6.7 Skew Heaps | 287 | ||
6.8 Binomial Queues | 289 | ||
6.8.1 Binomial Queue Structure | 289 | ||
6.8.2 Binomial Queue Operations | 289 | ||
6.8.3 Implementation of Binomial Queues | 294 | ||
6.9 Priority Queues in the Standard Library | 300 | ||
Summary | 301 | ||
Exercises | 301 | ||
References | 306 | ||
Chapter 7 Sorting | 309 | ||
7.1 Preliminaries | 309 | ||
7.2 Insertion Sort | 310 | ||
7.2.1 The Algorithm | 310 | ||
7.2.2 STL Implementation of Insertion Sort | 311 | ||
7.2.3 Analysis of Insertion Sort | 312 | ||
7.3 A Lower Bound for Simple Sorting Algorithms | 313 | ||
7.4 Shellsort | 314 | ||
7.4.1 Worst-Case Analysis of Shellsort | 315 | ||
7.5 Heapsort | 318 | ||
7.5.1 Analysis of Heapsort | 319 | ||
7.6 Mergesort | 322 | ||
7.6.1 Analysis of Mergesort | 324 | ||
7.7 Quicksort | 327 | ||
7.7.1 Picking the Pivot | 329 | ||
7.7.2 Partitioning Strategy | 331 | ||
7.7.3 Small Arrays | 333 | ||
7.7.4 Actual Quicksort Routines | 333 | ||
7.7.5 Analysis of Quicksort | 336 | ||
7.7.6 A Linear-Expected-Time Algorithm for Selection | 339 | ||
7.8 A General Lower Bound for Sorting | 341 | ||
7.8.1 Decision Trees | 341 | ||
7.9 Decision-Tree Lower Bounds for Selection Problems | 343 | ||
7.10 Adversary Lower Bounds | 346 | ||
7.11 Linear-Time Sorts: Bucket Sort and Radix Sort | 349 | ||
7.12 External Sorting | 354 | ||
7.12.1 Why We Need New Algorithms | 354 | ||
7.12.2 Model for External Sorting | 354 | ||
7.12.3 The Simple Algorithm | 355 | ||
7.12.4 Multiway Merge | 356 | ||
7.12.5 Polyphase Merge | 357 | ||
7.12.6 Replacement Selection | 358 | ||
Summary | 359 | ||
Exercises | 359 | ||
References | 365 | ||
Chapter 8 The Disjoint Sets Class | 369 | ||
8.1 Equivalence Relations | 369 | ||
8.2 The Dynamic Equivalence Problem | 370 | ||
8.3 Basic Data Structure | 371 | ||
8.4 Smart Union Algorithms | 375 | ||
8.5 Path Compression | 378 | ||
8.6 Worst Case for Union-by-Rank and Path Compression | 379 | ||
8.6.1 Slowly Growing Functions | 380 | ||
8.6.2 An Analysis by Recursive Decomposition | 380 | ||
8.6.3 An O( M log *N ) Bound | 387 | ||
8.6.4 An O( M α(M, N) ) Bound | 388 | ||
8.7 An Application | 390 | ||
Summary | 392 | ||
Exercises | 393 | ||
References | 394 | ||
Chapter 9 Graph Algorithms | 397 | ||
9.1 Definitions | 397 | ||
9.1.1 Representation of Graphs | 398 | ||
9.2 Topological Sort | 400 | ||
9.3 Shortest-Path Algorithms | 404 | ||
9.3.1 Unweighted Shortest Paths | 405 | ||
9.3.2 Dijkstra’s Algorithm | 409 | ||
9.3.3 Graphs with Negative Edge Costs | 418 | ||
9.3.4 Acyclic Graphs | 418 | ||
9.3.5 All-Pairs Shortest Path | 422 | ||
9.3.6 Shortest Path Example | 422 | ||
9.4 Network Flow Problems | 424 | ||
9.4.1 A Simple Maximum-Flow Algorithm | 426 | ||
9.5 Minimum Spanning Tree | 431 | ||
9.5.1 Prim’s Algorithm | 432 | ||
9.5.2 Kruskal’s Algorithm | 435 | ||
9.6 Applications of Depth-First Search | 437 | ||
9.6.1 Undirected Graphs | 438 | ||
9.6.2 Biconnectivity | 439 | ||
9.6.3 Euler Circuits | 443 | ||
9.6.4 Directed Graphs | 447 | ||
9.6.5 Finding Strong Components | 449 | ||
9.7 Introduction to NP-Completeness | 450 | ||
9.7.1 Easy vs. Hard | 451 | ||
9.7.2 The Class NP | 452 | ||
9.7.3 NP-Complete Problems | 452 | ||
Summary | 455 | ||
Exercises | 455 | ||
References | 463 | ||
Chapter 10 Algorithm Design Techniques | 467 | ||
10.1 Greedy Algorithms | 467 | ||
10.1.1 A Simple Scheduling Problem | 468 | ||
10.1.2 Huffman Codes | 471 | ||
10.1.3 Approximate Bin Packing | 477 | ||
10.2 Divide and Conquer | 485 | ||
10.2.1 Running Time of Divide-and-Conquer Algorithms | 486 | ||
10.2.2 Closest-Points Problem | 488 | ||
10.2.3 The Selection Problem | 493 | ||
10.2.4 Theoretical Improvements for Arithmetic Problems | 496 | ||
10.3 Dynamic Programming | 500 | ||
10.3.1 Using a Table Instead of Recursion | 501 | ||
10.3.2 Ordering Matrix Multiplications | 503 | ||
10.3.3 Optimal Binary Search Tree | 505 | ||
10.3.4 All-Pairs Shortest Path | 509 | ||
10.4 Randomized Algorithms | 512 | ||
10.4.1 Random-Number Generators | 513 | ||
10.4.2 Skip Lists | 518 | ||
10.4.3 Primality Testing | 521 | ||
10.5 Backtracking Algorithms | 524 | ||
10.5.1 The Turnpike Reconstruction Problem | 524 | ||
10.5.2 Games | 529 | ||
Summary | 536 | ||
Exercises | 536 | ||
References | 545 | ||
Chapter 11 Amortized Analysis | 551 | ||
11.1 An Unrelated Puzzle | 552 | ||
11.2 Binomial Queues | 552 | ||
11.3 Skew Heaps | 557 | ||
11.4 Fibonacci Heaps | 559 | ||
11.4.1 Cutting Nodes in Leftist Heaps | 560 | ||
11.4.2 Lazy Merging for Binomial Queues | 562 | ||
11.4.3 The Fibonacci Heap Operations | 566 | ||
11.4.4 Proof of the Time Bound | 567 | ||
11.5 Splay Trees | 569 | ||
Summary | 573 | ||
Exercises | 574 | ||
References | 575 | ||
Chapter 12 Advanced Data Structuresand Implementation | 577 | ||
12.1 Top-Down Splay Trees | 577 | ||
12.2 Red-Black Trees | 584 | ||
12.2.1 Bottom-Up Insertion | 585 | ||
12.2.2 Top-Down Red-Black Trees | 586 | ||
12.2.3 Top-Down Deletion | 588 | ||
12.3 Treaps | 594 | ||
12.4 Suffix Arrays and Suffix Trees | 597 | ||
12.4.1 Suffix Arrays | 598 | ||
12.4.2 Suffix Trees | 601 | ||
12.4.3 Linear-Time Construction of Suffix Arrays and Suffix Trees | 604 | ||
12.5 k-d Trees | 614 | ||
12.6 Pairing Heaps | 620 | ||
Summary | 624 | ||
Exercises | 626 | ||
References | 630 | ||
Appendix A Separate Compilationof Class Templates | 633 | ||
A.1 Everything in the Header | 634 | ||
A.2 Explicit Instantiation | 634 | ||
Index | 637 | ||
A | 637 | ||
B | 638 | ||
C | 639 | ||
D | 640 | ||
E | 641 | ||
F | 642 | ||
G | 643 | ||
H | 643 | ||
I | 644 | ||
J | 644 | ||
K | 644 | ||
L | 645 | ||
M | 645 | ||
N | 646 | ||
O | 647 | ||
P | 647 | ||
Q | 649 | ||
R | 649 | ||
S | 650 | ||
T | 652 | ||
U | 652 | ||
V | 653 | ||
W | 653 | ||
X | 653 | ||
Y | 653 | ||
Z | 653 |