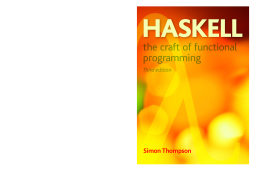
Additional Information
Book Details
Abstract
Introducing functional programming in the Haskell language, this book is written for students and programmers with little or no experience. It emphasises the process of crafting programmes, problem solving and avoiding common programming pitfalls.
Covering basic functional programming, through abstraction to larger scale programming, students are lead step by step through the basics, before being introduced to more advanced topics.
This edition includes new material on testing and domain-specific languages and a variety of new examples and case studies, including simple games. Existing material has been expanded and re-ordered, so that some concepts such as simple data types and input/output are presented at an earlier stage.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover\r | Cover | ||
Contents | vii | ||
Preface | xiii | ||
Chapter 1: Introducing functional programming\r | 1 | ||
1.1 Computers and modelling\r | 2 | ||
1.2 What is a function?\r | 3 | ||
1.3 Pictures and functions\r | 4 | ||
1.4 Types\r | 5 | ||
1.5 The Haskell programming language\r | 7 | ||
1.6 Expressions and evaluation\r | 8 | ||
1.7 Definitions\r | 9 | ||
1.8 Function definitions\r | 11 | ||
1.9 Types and functional programming\r | 14 | ||
1.10 Calculation and evaluation\r | 15 | ||
1.11 The essence of Haskell programming\r | 16 | ||
1.12 Domain-specific languages\r | 17 | ||
1.13 Two models of Pictures\r | 18 | ||
1.14 Tests, properties and proofs\r | 22 | ||
Summary | 25 | ||
Chapter 2: Getting started with Haskell and GHCi\r | 27 | ||
2.1 A first Haskell program\r | 27 | ||
2.2 Using Haskell in practice\r | 28 | ||
2.3 Using GHCi\r | 29 | ||
2.4 The standard prelude and the Haskell libraries\r | 33 | ||
2.5 Modules\r | 34 | ||
2.6 A second example: pictures\r | 35 | ||
2.7 Errors and error messages\r | 38 | ||
Summary | 40 | ||
Chapter 3: Basic types and definitions\r | 41 | ||
3.1 The Booleans: Bool\r | 42 | ||
3.2 The integers: Integer and Int\r | 45 | ||
3.3 Overloading\r | 48 | ||
3.4 Guards\r | 48 | ||
3.5 Characters and strings\r | 52 | ||
3.6 Floating-point numbers: Float\r | 56 | ||
3.7 Syntax\r | 60 | ||
Summary | 66 | ||
Chapter 4: Designing and writing programs\r | 67 | ||
4.1 Where do I start? Designing a program in Haskell\r | 67 | ||
4.2 Solving a problem in steps: local definitions\r | 72 | ||
4.3 Defining types for ourselves: enumerated types\r | 78 | ||
4.4 Recursion\r | 81 | ||
4.5 Primitive recursion in practice\r | 84 | ||
4.6 Extended exercise: pictures\r | 87 | ||
4.7 General forms of recursion\r | 89 | ||
4.8 Program testing\r | 91 | ||
Summary | 95 | ||
Chapter 5: Data types, tuples and lists\r | 97 | ||
5.1 Introducing tuples and lists\r | 97 | ||
5.2 Tuple types\r | 100 | ||
5.3 Introducing algebraic types\r | 103 | ||
5.4 Our approach to lists\r | 109 | ||
5.5 Lists in Haskell\r | 109 | ||
5.6 List comprehensions\r | 111 | ||
5.7 A library database\r | 116 | ||
Summary | 121 | ||
Chapter 6: Programming with lists\r | 123 | ||
6.1 Generic functions: polymorphism\r | 123 | ||
6.2 Haskell list functions in the Prelude\r | 126 | ||
6.3 Finding your way around the Haskell libraries\r | 129 | ||
6.4 The Picture example: implementation\r | 135 | ||
6.5 Extended exercise: alternative implementations of pictures\r | 140 | ||
6.6 Extended exercise: positioned pictures\r | 144 | ||
6.7 Extended exercise: supermarket billing\r | 147 | ||
Summary | 154 | ||
Chapter 7: Defining functions over lists\r | 155 | ||
7.1 Pattern matching revisited\r | 155 | ||
7.2 Lists and list patterns\r | 157 | ||
7.3 Primitive recursion over lists\r | 160 | ||
7.4 Finding primitive recursive definitions\r | 161 | ||
7.5 General recursions over lists\r | 167 | ||
7.6 Example: text processing\r | 170 | ||
Summary | 175 | ||
Chapter 8: Playing the game: I/O in Haskell\r | 177 | ||
8.1 Rock – Paper – Scissors: strategies\r | 177 | ||
8.2 Why is I/O an issue?\r | 181 | ||
8.3 The basics of input/output\r | 182 | ||
8.4 The do notation\r | 185 | ||
8.5 Loops and recursion\r | 189 | ||
8.6 Rock – Paper – Scissors: playing the game\r | 191 | ||
Summary | 194 | ||
Chapter 9: Reasoning about programs\r | 195 | ||
9.1 Understanding definitions\r | 196 | ||
9.2 Testing and proof\r | 197 | ||
9.3 Definedness, termination and finiteness\r | 199 | ||
9.4 A little logic\r | 200 | ||
9.5 Induction\r | 201 | ||
9.6 Further examples of proofs by induction\r | 205 | ||
9.7 Generalizing the proof goal\r | 209 | ||
Summary | 212 | ||
Chapter 10: Generalization: patterns of computation\r | 213 | ||
10.1 Patterns of computation over lists\r | 214 | ||
10.2 Higher-order functions: functions as arguments\r | 216 | ||
10.3 Folding and primitive recursion\r | 221 | ||
10.4 Generalizing: splitting up lists\r | 226 | ||
10.5 Case studies revisited\r | 227 | ||
Summary | 229 | ||
Chapter 11: Higher-order functions\r | 231 | ||
11.1 Operators: function composition and application\r | 232 | ||
11.2 Expressions for functions: lambda abstractions\r | 235 | ||
11.3 Partial application\r | 239 | ||
11.4 Under the hood: curried functions\r | 242 | ||
11.5 Defining higher-order functions\r | 247 | ||
11.6 Verification and general functions\r | 253 | ||
Summary | 262 | ||
Chapter 12: Developing higher-order programs\r | 263 | ||
12.1 Revisiting the Picture example\r | 263 | ||
12.2 Functions as data: strategy combinators\r | 266 | ||
12.3 Functions as data: recognizing regular expressions\r | 269 | ||
12.4 Case studies: functions as data\r | 272 | ||
12.5 Example: creating an index\r | 275 | ||
12.6 Development in practice\r | 281 | ||
12.7 Understanding programs\r | 284 | ||
Summary | 286 | ||
Chapter 13: Overloading, type classes and type checking\r | 287 | ||
13.1 Why overloading?\r | 287 | ||
13.2 Introducing classes\r | 288 | ||
13.3 Signatures and instances\r | 292 | ||
13.4 A tour of the built-in Haskell classes\r | 299 | ||
13.5 Type checking and type inference: an overview\r | 308 | ||
13.6 Monomorphic type checking\r | 309 | ||
13.7 Polymorphic type checking\r | 312 | ||
13.8 Type checking and classes\r | 320 | ||
Summary | 322 | ||
Chapter 14: Algebraic types\r | 323 | ||
14.1 Algebraic type definitions revisited\r | 324 | ||
14.2 Recursive algebraic types\r | 326 | ||
14.3 Polymorphic algebraic types\r | 333 | ||
14.4 Modelling program errors\r | 337 | ||
14.5 Design with algebraic data types\r | 341 | ||
14.6 Algebraic types and type classes\r | 345 | ||
14.7 Reasoning about algebraic types\r | 350 | ||
Summary | 354 | ||
Chapter 15: Case study: Huffman codes\r | 357 | ||
15.1 Modules in Haskell\r | 357 | ||
15.2 Modular design\r | 361 | ||
15.3 Coding and decoding\r | 362 | ||
15.4 Implementation – I\r | 364 | ||
15.5 Building Huffman trees\r | 367 | ||
15.6 Design\r | 368 | ||
15.7 Implementation – II\r | 369 | ||
Summary | 376 | ||
Chapter 16: Abstract data types\r | 377 | ||
16.1 Type representations\r | 377 | ||
16.2 The Haskell abstract data type mechanism\r | 379 | ||
16.3 Queues\r | 383 | ||
16.4 Design\r | 386 | ||
16.5 Simulation\r | 388 | ||
16.6 Implementing the simulation\r | 390 | ||
16.7 Search trees\r | 394 | ||
16.8 Sets\r | 400 | ||
16.9 Relations and graphs\r | 405 | ||
16.10 Commentary\r | 413 | ||
Summary | 414 | ||
Chapter 17: Lazy programming\r | 415 | ||
17.1 Lazy evaluation\r | 416 | ||
17.2 Calculation rules and lazy evaluation\r | 418 | ||
17.3 List comprehensions revisited\r | 421 | ||
17.4 Data-directed programming\r | 428 | ||
17.5 Case study: parsing expressions\r | 432 | ||
17.6 Infinite lists\r | 442 | ||
17.7 Why infinite lists?\r | 447 | ||
17.8 Case study: simulation\r | 450 | ||
17.9 Proof revisited\r | 452 | ||
Summary | 457 | ||
Chapter 18: Programming with monads\r | 459 | ||
18.1 I/O programming\r | 459 | ||
18.2 Further I/O\r | 462 | ||
18.3 The calculator\r | 465 | ||
18.4 The do notation revisited\r | 468 | ||
18.5 Monads: languages for functional programming\r | 469 | ||
18.6 Example: monadic computation over trees\r | 476 | ||
Summary | 482 | ||
Chapter 19: Domain-specific languages\r | 483 | ||
19.1 Programming languages everywhere\r | 483 | ||
19.2 Why DSLs in Haskell?\r | 486 | ||
19.3 Shallow and deep embeddings\r | 487 | ||
19.4 A DSL for regular expressions\r | 490 | ||
19.5 Monadic DSLs\r | 495 | ||
19.6 DSLs for computation: generating data in QuickCheck\r | 498 | ||
19.7 Taking it further\r | 503 | ||
Summary | 504 | ||
Chapter 20: Time and space behaviour\r | 505 | ||
20.1 Complexity of functions\r | 505 | ||
20.2 The complexity of calculations\r | 509 | ||
20.3 Implementations of sets\r | 513 | ||
20.4 Space behaviour\r | 514 | ||
20.5 Folding revisited\r | 517 | ||
20.6 Avoiding recomputation: memoization\r | 523 | ||
Summary | 528 | ||
Chapter 21: Conclusion\r | 529 | ||
Appendix A: Functional, imperative and OO programming\r | 535 | ||
Appendix B: Glossary\r | 543 | ||
Appendix C: Haskell operators\r | 551 | ||
Appendix D: Haskell practicalities\r | 553 | ||
Appendix E: GHCi errors\r | 555 | ||
Appendix F: Project ideas\r | 561 | ||
Bibliography\r | 567 | ||
Index | 571 |