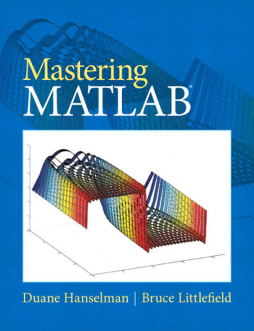
Additional Information
Book Details
Abstract
For undergraduate and graduate courses in MATLAB or as a reference in courses where MATLAB is used.
This text covers all essential aspects of MATLAB presented within an easy- to-follow "learn while doing" tutorial format.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Contents | 5 | ||
Preface | 13 | ||
1 Getting Started | 17 | ||
1.1 Introduction | 17 | ||
1.2 Typographical Conventions | 17 | ||
1.3 What’s New in MATLAB | 18 | ||
1.4 What’s in Mastering MATLAB | 18 | ||
2 Basic Features | 20 | ||
2.1 Simple Math | 20 | ||
2.2 The MATLAB Workspace | 22 | ||
2.3 About Variables | 23 | ||
2.4 Comments, Punctuation, and Aborting Execution | 26 | ||
2.5 Complex Numbers | 28 | ||
2.6 Floating-Point Arithmetic | 30 | ||
2.7 Mathematical Functions | 32 | ||
3 The MATLAB Desktop | 38 | ||
3.1 MATLAB Windows | 38 | ||
3.2 Managing the MATLAB Workspace | 39 | ||
3.3 Memory Management | 43 | ||
3.4 Number Display Formats | 43 | ||
3.5 System Information | 44 | ||
3.6 The MATLAB Search Path | 46 | ||
4 Script M-files | 47 | ||
4.1 Script M-file Use | 47 | ||
4.2 Block Comments and Code Cells | 51 | ||
4.3 Setting Execution Time | 53 | ||
4.4 Startup and Finish | 54 | ||
5 Arrays and Array Operations | 56 | ||
5.1 Simple Arrays | 56 | ||
5.2 Array Addressing or Indexing | 57 | ||
5.3 Array Construction | 59 | ||
5.4 Array Orientation | 62 | ||
5.5 Scalar–Array Mathematics | 66 | ||
5.6 Array–Array Mathematics | 66 | ||
5.7 Standard Arrays | 73 | ||
5.8 Array Manipulation | 77 | ||
5.9 Array Sorting | 92 | ||
5.10 Subarray Searching | 95 | ||
5.11 Array-Manipulation Functions | 102 | ||
5.12 Array Size | 108 | ||
5.13 Arrays and Memory Utilization | 111 | ||
6 Multidimensional Arrays | 117 | ||
6.1 Array Construction | 117 | ||
6.2 Array Mathematics and Manipulation | 121 | ||
6.3 Array Size | 132 | ||
7 Numeric Data Types | 135 | ||
7.1 Integer Data Types | 135 | ||
7.2 Floating-Point Data Types | 141 | ||
7.3 Summary | 144 | ||
8 Cell Arrays and Structures | 145 | ||
8.1 Cell Array Creation | 146 | ||
8.2 Cell Array Manipulation | 149 | ||
8.3 Retrieving Cell Array Content | 151 | ||
8.4 Comma-Separated Lists | 155 | ||
8.5 Cell Functions | 159 | ||
8.6 Cell Arrays of Strings | 162 | ||
8.7 Structure Creation | 164 | ||
8.8 Structure Manipulation | 169 | ||
8.9 Retrieving Structure Content | 171 | ||
8.10 Comma-Separated Lists (Again) | 173 | ||
8.11 Structure Functions | 176 | ||
8.12 Summary | 180 | ||
9 Character Strings | 181 | ||
9.1 String Construction | 181 | ||
9.2 Numbers to Strings to Numbers | 187 | ||
9.3 String Evaluation | 194 | ||
9.4 String Functions | 195 | ||
9.5 Cell Arrays of Strings | 199 | ||
9.6 Searching Using Regular Expressions | 203 | ||
10 Relational and Logical Operations | 211 | ||
10.1 Relational Operators | 211 | ||
10.2 Logical Operators | 215 | ||
10.3 Operator Precedence | 216 | ||
10.4 Relational and Logical Functions | 217 | ||
10.5 NaNs and Empty Arrays | 220 | ||
11 Control Flow | 224 | ||
11.1 For Loops | 224 | ||
11.2 While Loops | 230 | ||
11.3 If-Else-End Constructions | 231 | ||
11.4 Switch-Case Constructions | 234 | ||
11.5 Try-Catch Blocks | 236 | ||
12 Functions | 239 | ||
12.1 M-file Function Construction Rules | 240 | ||
12.2 Input and Output Arguments | 245 | ||
12.3 Function Workspaces | 248 | ||
12.4 Functions and the MATLAB Search Path | 252 | ||
12.5 Creating Your Own Toolbox | 255 | ||
12.6 Command–Function Duality | 256 | ||
12.7 Function Handles and Anonymous Functions | 257 | ||
12.8 Nested Functions | 263 | ||
12.9 Debugging M-files | 267 | ||
12.10 Syntax Checking and File Dependencies | 269 | ||
12.11 Profiling M-files | 270 | ||
13 File and Directory Management | 272 | ||
13.1 Native Data Files | 272 | ||
13.2 Data Import and Export | 275 | ||
13.3 Low-Level File I/O | 279 | ||
13.4 Directory Management | 281 | ||
13.5 File Archives and Compression | 285 | ||
13.6 Internet File Operations | 286 | ||
14 Set, Bit, and Base Functions | 289 | ||
14.1 Set Functions | 289 | ||
14.2 Bit Functions | 294 | ||
14.3 Base Conversions | 295 | ||
15 Time Computations | 297 | ||
15.1 Current Date and Time | 297 | ||
15.2 Date Format Conversions | 298 | ||
15.3 Date Functions | 304 | ||
15.4 Timing Functions | 306 | ||
15.5 Plot Labels | 307 | ||
16 Matrix Algebra | 310 | ||
16.1 Sets of Linear Equations | 310 | ||
16.2 Matrix Functions | 315 | ||
16.3 Special Matrices | 317 | ||
16.4 Sparse Matrices | 318 | ||
16.5 Sparse Matrix Functions | 320 | ||
17 Data Analysis | 323 | ||
17.1 Basic Statistical Analysis | 323 | ||
17.2 Basic Data Analysis | 337 | ||
17.3 Data Analysis and Statistical Functions | 343 | ||
17.4 Time Series Analysis | 344 | ||
18 Data Interpolation | 348 | ||
18.1 One-Dimensional Interpolation | 348 | ||
18.2 Two-Dimensional Interpolation | 353 | ||
18.3 Triangulation and Scattered Data | 357 | ||
18.4 Summary | 365 | ||
19 Polynomials | 367 | ||
19.1 Roots | 367 | ||
19.2 Multiplication | 368 | ||
19.3 Addition | 368 | ||
19.4 Division | 370 | ||
19.5 Derivatives and Integrals | 371 | ||
19.6 Evaluation | 372 | ||
19.7 Rational Polynomials | 372 | ||
19.8 Curve Fitting | 374 | ||
19.9 Polynomial Functions | 377 | ||
20 Cubic Splines | 379 | ||
20.1 Basic Features | 379 | ||
20.2 Piecewise Polynomials | 380 | ||
20.3 Cubic Hermite Polynomials | 383 | ||
20.4 Integration | 385 | ||
20.5 Differentiation | 388 | ||
20.6 Spline Interpolation on a Plane | 389 | ||
21 Fourier Analysis | 393 | ||
21.1 Discrete Fourier Transform | 393 | ||
21.2 Fourier Series | 397 | ||
22 Optimization | 402 | ||
22.1 Zero Finding | 402 | ||
22.2 Minimization in One Dimension | 407 | ||
22.3 Minimization in Higher Dimensions | 409 | ||
22.4 Practical Issues | 412 | ||
23 Integration and Differentiation | 414 | ||
23.1 Integration | 414 | ||
23.2 Differentiation | 420 | ||
24 Differential Equations | 427 | ||
24.1 IVP Format | 427 | ||
24.2 ODE Suite Solvers | 428 | ||
24.3 Basic Use | 429 | ||
24.4 Setting Options | 433 | ||
24.5 BVPs, PDEs, and DDEs | 441 | ||
25 Two-Dimensional Graphics | 442 | ||
25.1 The plot Function | 442 | ||
25.2 Linestyles, Markers, and Colors | 444 | ||
25.3 Plot Grids, Axes Box, and Labels | 446 | ||
25.4 Customizing Plot Axes | 448 | ||
25.5 Multiple Plots | 451 | ||
25.6 Multiple Figures | 451 | ||
25.7 Subplots | 453 | ||
25.8 Interactive Plotting Tools | 454 | ||
25.9 Screen Updates | 456 | ||
25.10 Specialized 2-D Plots | 457 | ||
25.11 Easy Plotting | 465 | ||
25.12 Text Formatting | 467 | ||
25.13 Summary | 469 | ||
26 Three-Dimensional Graphics | 472 | ||
26.1 Line Plots | 472 | ||
26.2 Scalar Functions of Two Variables | 474 | ||
26.3 Mesh Plots | 478 | ||
26.4 Surface Plots | 481 | ||
26.5 Mesh and Surface Plots of Irregular Data | 487 | ||
26.6 Changing Viewpoints | 489 | ||
26.7 Camera Control | 491 | ||
26.8 Contour Plots | 492 | ||
26.9 Specialized 3-D Plots | 494 | ||
26.10 Volume Visualization | 498 | ||
26.11 Easy Plotting | 505 | ||
26.12 Summary | 507 | ||
27 Using Color and Light | 511 | ||
27.1 Understanding Colormaps | 511 | ||
27.2 Using Colormaps | 513 | ||
27.3 Displaying Colormaps | 514 | ||
27.4 Creating and Altering Colormaps | 516 | ||
27.5 Using Color to Describe a Fourth Dimension | 518 | ||
27.6 Transparency | 521 | ||
27.7 Lighting Models | 523 | ||
27.8 Summary | 527 | ||
28 Images, Movies, and Sound | 529 | ||
28.1 Images | 529 | ||
28.2 Image Formats | 531 | ||
28.3 Image Files | 532 | ||
28.4 Movies | 534 | ||
28.5 Movie Files | 536 | ||
28.6 Sound | 540 | ||
28.7 Summary | 541 | ||
29 Printing and Exporting Graphics | 543 | ||
29.1 Printing and Exporting Using Menus | 544 | ||
29.2 Command Line Printing and Exporting | 545 | ||
29.3 Printers and Export File Formats | 546 | ||
29.4 PostScript Support | 548 | ||
29.5 Choosing a Renderer | 549 | ||
29.6 Handle Graphics Properties | 550 | ||
29.7 Setting Defaults | 552 | ||
29.8 Publishing | 553 | ||
29.9 Summary | 554 | ||
30 Handle Graphics | 555 | ||
30.1 Objects | 555 | ||
30.2 Object Handles | 557 | ||
30.3 Object Properties | 557 | ||
30.4 Get and Set | 558 | ||
30.5 Finding Objects | 566 | ||
30.6 Selecting Objects with the Mouse | 568 | ||
30.7 Position and Units | 569 | ||
30.8 Default Properties | 572 | ||
30.9 Common Properties | 575 | ||
30.10 Plot Objects | 577 | ||
30.11 Group Objects | 578 | ||
30.12 Annotation Axes | 580 | ||
30.13 Linking Objects | 581 | ||
30.14 New Plots | 582 | ||
30.15 Callbacks | 583 | ||
30.16 M-file Examples | 584 | ||
30.17 Summary | 591 | ||
31 MATLAB Classes and Object-Oriented Programming | 595 | ||
31.1 Overloading | 597 | ||
31.2 Class Creation | 603 | ||
31.3 Subscripts | 618 | ||
31.4 Converter Functions | 629 | ||
31.5 Precedence, Inheritance, and Aggregation | 630 | ||
31.6 Handle Classes | 632 | ||
32 Examples, Examples, Examples | 633 | ||
32.1 Vectorization | 633 | ||
32.2 JIT-Acceleration | 636 | ||
32.3 The Birthday Problem | 636 | ||
32.4 Up–Down Sequence | 641 | ||
32.5 Alternating Sequence Matrix | 647 | ||
32.6 Vandermonde Matrix | 652 | ||
32.7 Repeated Value Creation and Counting | 655 | ||
32.8 Differential Sums | 665 | ||
32.9 Structure Manipulation | 673 | ||
32.10 Inverse Interpolation | 676 | ||
32.11 Polynomial Curve Fitting | 684 | ||
32.12 Nonlinear Curve Fitting | 692 | ||
32.13 Circle Fitting | 701 | ||
32.14 Laminar Fluid Flow in a Circular Pipe | 706 | ||
32.15 Projectile Motion | 712 | ||
32.16 Bode Plots | 723 | ||
32.17 Inverse Laplace Transform | 734 | ||
32.18 Picture-in-a-Picture Zoom | 740 | ||
Appendix A: MATLAB Release Information | 747 | ||
Appendix B: MATLAB Function Information | 805 | ||
Index | 851 | ||
A | 851 | ||
B | 852 | ||
C | 852 | ||
D | 852 | ||
E | 853 | ||
F | 853 | ||
G | 854 | ||
H | 854 | ||
I | 854 | ||
J | 855 | ||
L | 855 | ||
M | 855 | ||
N | 856 | ||
O | 856 | ||
P | 856 | ||
Q | 857 | ||
R | 857 | ||
S | 857 | ||
T | 858 | ||
U | 859 | ||
V | 859 | ||
W | 859 | ||
X | 859 | ||
Y | 859 | ||
Z | 859 |