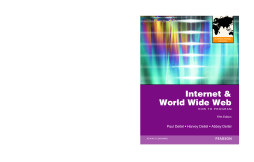
BOOK
Internet and World Wide Web How to Program
Harvey M. Deitel | Paul J. Deitel | Abbey Deitel
(2014)
Additional Information
Book Details
Abstract
For a wide variety of Web Programming, XHTML, and JavaScript courses found in Computer Science, CIS, MIS, IT, Business, Engineering, and Continuing Education departments.
Internet and World Wide Web How to Program, 5e introduces students with little or no programming experience to the exciting world of Web-Based applications. The book has been substantially revised to reflect today's Web 2.0 rich Internet application-development methodologies. A comprehensive book that teaches the fundamentals needed to program on the Internet, this text provides in-depth coverage of introductory programming principles, various markup languages (XHTML, Dynamic HTML and XML), several scripting languages (JavaScript, PHP, Ruby/Ruby on Rails and Perl); AJAX, web services, Web Servers (IIS and Apache) and relational databases (MySQL/Apache Derby/Java DB)all the skills and tools needed to create dynamic Web-based applications. The text contains comprehensive introductions to ASP.NET and JavaServer Faces (JSF). Hundreds of live-code examples of real applications throughout the book available for download allow readers to run the applications and see and hear the outputs. The book provides instruction on building Ajax-enabled rich Internet applications that enhance the presentation of online content and give web applications the look and feel of desktop applications. The chapter on Web 2.0 and Internet business exposes readers to a wide range of other topics associated with Web 2.0 applications and businesses. After mastering the material in this book, students will be well prepared to build real-world, industrial strength, Web-based applications.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover\r | Cover | ||
Title | Title | ||
Contents | 7 | ||
Preface | 19 | ||
Before You Begin | 31 | ||
1 Introduction to Computers and the Internet | 33 | ||
1.1 Introduction | 34 | ||
1.2 The Internet in Industry and Research | 35 | ||
1.3 HTML5, CSS3, JavaScript, Canvas and jQuery | 38 | ||
1.4 Demos | 41 | ||
1.5 Evolution of the Internet and World Wide Web | 42 | ||
1.6 Web Basics | 44 | ||
1.7 Multitier Application Architecture | 48 | ||
1.8 Client-Side Scripting versus Server-Side Scripting | 49 | ||
1.9 World Wide Web Consortium (W3C) | 50 | ||
1.10 Web 2.0: Going Social | 50 | ||
1.11 Data Hierarchy | 55 | ||
1.12 Operating Systems | 57 | ||
1.12.1 Desktop and Notebook Operating Systems | 57 | ||
1.12.2 Mobile Operating Systems | 58 | ||
1.13 Types of Programming Languages | 59 | ||
1.14 Object Technology | 61 | ||
1.15 Keeping Up-to-Date with Information Technologies | 63 | ||
2 Introduction to HTML5: Part 1 | 69 | ||
2.1 Introduction | 70 | ||
2.2 Editing HTML5 | 70 | ||
2.3 First HTML5 Example | 70 | ||
2.4 W3C HTML5 Validation Service | 73 | ||
2.5 Headings | 73 | ||
2.6 Linking | 74 | ||
2.7 Images | 77 | ||
2.7.1 alt Attribute | 79 | ||
2.7.2 Void Elements | 79 | ||
2.7.3 Using Images as Hyperlinks | 79 | ||
2.8 Special Characters and Horizontal Rules | 81 | ||
2.9 Lists | 83 | ||
2.10 Tables | 86 | ||
2.11 Forms | 90 | ||
2.12 Internal Linking | 97 | ||
2.13 meta Elements | 99 | ||
2.14 Web Resources | 101 | ||
3 Introduction to HTML5: Part 2 | 108 | ||
3.1 Introduction | 109 | ||
3.2 New HTML5 Form input Types | 109 | ||
3.2.1 input Type color | 112 | ||
3.2.2 input Type date | 114 | ||
3.2.3 input Type datetime | 114 | ||
3.2.4 input Type datetime-local | 114 | ||
3.2.5 input Type email | 115 | ||
3.2.6 input Type month | 116 | ||
3.2.7 input Type number | 116 | ||
3.2.8 input Type range | 117 | ||
3.2.9 input Type search | 117 | ||
3.2.10 input Type tel | 118 | ||
3.2.11 input Type time | 118 | ||
3.2.12 input Type url | 119 | ||
3.2.13 input Type week | 119 | ||
3.3 input and datalist Elements and autocomplete Attribute | 119 | ||
3.3.1 input Element autocomplete Attribute | 119 | ||
3.3.2 datalist Element | 122 | ||
3.4 Page-Structure Elements | 122 | ||
3.4.1 header Element | 128 | ||
3.4.2 nav Element | 128 | ||
3.4.3 figure Element and figcaption Element | 128 | ||
3.4.4 article Element | 128 | ||
3.4.5 summary Element and details Element | 128 | ||
3.4.6 section Element | 128 | ||
3.4.7 aside Element | 128 | ||
3.4.8 meter Element | 129 | ||
3.4.9 footer Element | 130 | ||
3.4.10 Text-Level Semantics: mark Element and wbr Element | 130 | ||
4 Introduction to Cascading Style Sheets™(CSS): Part 1 | 137 | ||
4.1 Introduction | 138 | ||
4.2 Inline Styles | 138 | ||
4.3 Embedded Style Sheets | 140 | ||
4.4 Conflicting Styles | 143 | ||
4.5 Linking External Style Sheets | 146 | ||
4.6 Positioning Elements: Absolute Positioning, z-index | 148 | ||
4.7 Positioning Elements: Relative Positioning, span | 150 | ||
4.8 Backgrounds | 152 | ||
4.9 Element Dimensions | 154 | ||
4.10 Box Model and Text Flow | 155 | ||
4.11 Media Types and Media Queries | 159 | ||
4.12 Drop-Down Menus | 162 | ||
4.13 (Optional) User Style Sheets | 164 | ||
4.14 Web Resources | 168 | ||
5 Introduction to Cascading Style Sheets™(CSS): Part 2 | 174 | ||
5.1 Introduction | 175 | ||
5.2 Text Shadows | 175 | ||
5.3 Rounded Corners | 176 | ||
5.4 Color | 177 | ||
5.5 Box Shadows | 178 | ||
5.6 Linear Gradients; Introducing Vendor Prefixes | 180 | ||
5.7 Radial Gradients | 183 | ||
5.8 (Optional: WebKit Only) Text Stroke | 185 | ||
5.9 Multiple Background Images | 185 | ||
5.10 (Optional: WebKit Only) Reflections | 187 | ||
5.11 Image Borders | 188 | ||
5.12 Animation; Selectors | 191 | ||
5.13 Transitions and Transformations | 194 | ||
5.13.1 transition and transform Properties | 194 | ||
5.13.2 Skew | 196 | ||
5.13.3 Transitioning Between Images | 197 | ||
5.14 Downloading Web Fonts and the @font-face Rule | 198 | ||
5.15 Flexible Box Layout Module and :nth-child Selectors | 200 | ||
5.16 Multicolumn Layout | 203 | ||
5.17 Media Queries | 205 | ||
5.18 Web Resources | 209 | ||
6 JavaScript: Introduction to Scripting | 217 | ||
6.1 Introduction | 218 | ||
6.2 Your First Script: Displaying a Line of Text with JavaScript in a Web Page | 218 | ||
6.3 Modifying Your First Script | 221 | ||
6.4 Obtaining User Input with prompt Dialogs | 224 | ||
6.4.1 Dynamic Welcome Page | 224 | ||
6.4.2 Adding Integers | 228 | ||
6.5 Memory Concepts | 231 | ||
6.6 Arithmetic | 232 | ||
6.7 Decision Making: Equality and Relational Operators | 234 | ||
6.8 Web Resources | 239 | ||
7 JavaScript: Control Statements I | 246 | ||
7.1 Introduction | 247 | ||
7.2 Algorithms | 247 | ||
7.3 Pseudocode | 247 | ||
7.4 Control Statements | 247 | ||
7.5 if Selection Statement | 250 | ||
7.6 if…else Selection Statement | 251 | ||
7.7 while Repetition Statement | 255 | ||
7.8 Formulating Algorithms: Counter-Controlled Repetition | 257 | ||
7.9 Formulating Algorithms: Sentinel-Controlled Repetition | 260 | ||
7.10 Formulating Algorithms: Nested Control Statements | 266 | ||
7.11 Assignment Operators | 270 | ||
7.12 Increment and Decrement Operators | 271 | ||
7.13 Web Resources | 274 | ||
8 JavaScript: Control Statements II | 283 | ||
8.1 Introduction | 284 | ||
8.2 Essentials of Counter-Controlled Repetition | 284 | ||
8.3 for Repetition Statement | 285 | ||
8.4 Examples Using the for Statement | 288 | ||
8.5 switch Multiple-Selection Statement | 293 | ||
8.6 do…while Repetition Statement | 296 | ||
8.7 break and continue Statements | 298 | ||
8.8 Logical Operators | 300 | ||
8.9 Web Resources | 303 | ||
9 JavaScript: Functions | 310 | ||
9.1 Introduction | 311 | ||
9.2 Program Modules in JavaScript | 311 | ||
9.3 Function Definitions | 312 | ||
9.3.1 Programmer-Defined Function square | 313 | ||
9.3.2 Programmer-Defined Function maximum | 315 | ||
9.4 Notes on Programmer-Defined Functions | 317 | ||
9.5 Random Number Generation | 318 | ||
9.5.1 Scaling and Shifting Random Numbers | 318 | ||
9.5.2 Displaying Random Images | 319 | ||
9.5.3 Rolling Dice Repeatedly and Displaying Statistics | 323 | ||
9.6 Example: Game of Chance; Introducing the HTML5audio and video Elements | 328 | ||
9.7 Scope Rules | 338 | ||
9.8 JavaScript Global Functions | 340 | ||
9.9 Recursion | 341 | ||
9.10 Recursion vs. Iteration | 345 | ||
10 JavaScript: Arrays | 356 | ||
10.1 Introduction | 357 | ||
10.2 Arrays | 357 | ||
10.3 Declaring and Allocating Arrays | 359 | ||
10.4 Examples Using Arrays | 359 | ||
10.4.1 Creating, Initializing and Growing Arrays | 359 | ||
10.4.2 Initializing Arrays with Initializer Lists | 363 | ||
10.4.3 Summing the Elements of an Array with for and for…in | 364 | ||
10.4.4 Using the Elements of an Array as Counters | 366 | ||
10.5 Random Image Generator Using Arrays | 369 | ||
10.6 References and Reference Parameters | 371 | ||
10.7 Passing Arrays to Functions | 372 | ||
10.8 Sorting Arrays with Array Method sort | 375 | ||
10.9 Searching Arrays with Array Method indexOf | 376 | ||
10.10 Multidimensional Arrays | 379 | ||
11 JavaScript: Objects | 392 | ||
11.1 Introduction | 393 | ||
11.2 Math Object | 393 | ||
11.3 String Object | 395 | ||
11.3.1 Fundamentals of Characters and Strings | 395 | ||
11.3.2 Methods of the String Object | 395 | ||
11.3.3 Character-Processing Methods | 397 | ||
11.3.4 Searching Methods | 398 | ||
11.3.5 Splitting Strings and Obtaining Substrings | 401 | ||
11.4 Date Object | 403 | ||
11.5 Boolean and Number Objects | 408 | ||
11.6 document Object | 409 | ||
11.7 Favorite Twitter Searches: HTML5 Web Storage | 410 | ||
11.8 Using JSON to Represent Objects | 417 | ||
12 Document Object Model (DOM):Objects and Collections | 427 | ||
12.1 Introduction | 428 | ||
12.2 Modeling a Document: DOM Nodes and Trees | 428 | ||
12.3 Traversing and Modifying a DOM Tree | 431 | ||
12.4 DOM Collections | 441 | ||
12.5 Dynamic Styles | 443 | ||
12.6 Using a Timer and Dynamic Styles to Create Animated Effects | 445 | ||
13 JavaScript Event Handling: A Deeper Look | 454 | ||
13.1 Introduction\r | 455 | ||
13.2 Reviewing the load Event | 455 | ||
13.3 Event mousemove and the event Object | 457 | ||
13.4 Rollovers with mouseover and mouseout | 461 | ||
13.5 Form Processing with focus and blur | 465 | ||
13.6 More Form Processing with submit and reset | 468 | ||
13.7 Event Bubbling | 470 | ||
13.8 More Events | 472 | ||
13.9 Web Resource | 472 | ||
14 HTML5: Introduction to canvas | 476 | ||
14.1 Introduction | 477 | ||
14.2 canvas Coordinate System | 477 | ||
14.3 Rectangles | 478 | ||
14.4 Using Paths to Draw Lines | 480 | ||
14.5 Drawing Arcs and Circles | 482 | ||
14.6 Shadows | 484 | ||
14.7 Quadratic Curves | 486 | ||
14.8 Bezier Curves | 488 | ||
14.9 Linear Gradients | 489 | ||
14.10 Radial Gradients | 491 | ||
14.11 Images | 493 | ||
14.12 Image Manipulation: Processing the Individual Pixels of a canvas | 495 | ||
14.13 Patterns | 499 | ||
14.14 Transformations | 500 | ||
14.14.1 scale and translate Methods: Drawing Ellipses | 500 | ||
14.14.2 rotate Method: Creating an Animation | 502 | ||
14.14.3 transform Method: Drawing Skewed Rectangles | 504 | ||
14.15 Text | 506 | ||
14.16 Resizing the canvas to Fill the Browser Window | 508 | ||
14.17 Alpha Transparency | 509 | ||
14.18 Compositing | 511 | ||
14.19 Cannon Game | 514 | ||
14.19.1 HTML5 Document | 516 | ||
14.19.2 Instance Variables and Constants | 516 | ||
14.19.3 Function setupGame | 518 | ||
14.19.4 Functions startTimer and stopTimer | 519 | ||
14.19.5 Function resetElements | 519 | ||
14.19.6 Function newGame | 520 | ||
14.19.7 Function updatePositions: Manual Frame-by-FrameAnimation and Simple Collision Detection | 521 | ||
14.19.8 Function fireCannonball | 524 | ||
14.19.9 Function alignCannon | 525 | ||
14.19.10Function draw | 526 | ||
14.19.11Function showGameOverDialog | 528 | ||
14.20 save and restore Methods | 528 | ||
14.21 A Note on SVG | 530 | ||
14.22 A Note on canvas35D | 531 | ||
15 \rXML | 543 | ||
15.1 Introduction | 544 | ||
15.2 XML Basics | 544 | ||
15.3 Structuring Data | 547 | ||
15.4 XML Namespaces | 553 | ||
15.5 Document Type Definitions (DTDs) | 555 | ||
15.6 W3C XML Schema Documents | 558 | ||
15.7 XML Vocabularies | 566 | ||
15.7.1 MathML™ | 566 | ||
15.7.2 Other Markup Languages | 569 | ||
15.8 Extensible Stylesheet Language and XSL Transformations | 570 | ||
15.9 Document Object Model (DOM) | 579 | ||
15.10 Web Resources | 597 | ||
16 Ajax-Enabled Rich Internet Applicationswith XML and JSON | 603 | ||
16.1 Introduction | 604 | ||
16.1.1 Traditional Web Applications vs. Ajax Applications | 605 | ||
16.1.2 Traditional Web Applications | 605 | ||
16.1.3 Ajax Web Applications | 606 | ||
16.2 Rich Internet Applications (RIAs) with Ajax | 606 | ||
16.3 History of Ajax | 609 | ||
16.4 “Raw” Ajax Example Using the XMLHttpRequest Object | 609 | ||
16.4.1 Asynchronous Requests | 610 | ||
16.4.2 Exception Handling | 613 | ||
16.4.3 Callback Functions | 614 | ||
16.4.4 XMLHttpRequest Object Event, Properties and Methods | 614 | ||
16.5 Using XML and the DOM | 615 | ||
16.6 Creating a Full-Scale Ajax-Enabled Application | 619 | ||
16.6.1 Using JSON | 619 | ||
16.6.2 Rich Functionality | 620 | ||
16.6.3 Interacting with a Web Service on the Server | 629 | ||
16.6.4 Parsing JSON Data | 629 | ||
16.6.5 Creating HTML5 Elements and Setting Event Handlers on the Fly | 630 | ||
16.6.6 Implementing Type-Ahead | 630 | ||
16.6.7 Implementing a Form with Asynchronous Validation | 631 | ||
17 Web Servers (Apache and IIS) | 637 | ||
17.1 Introduction | 638 | ||
17.2 HTTP Transactions | 638 | ||
17.3 Multitier Application Architecture | 642 | ||
17.4 Client-Side Scripting versus Server-Side Scripting | 643 | ||
17.5 Accessing Web Servers | 643 | ||
17.6 Apache, MySQL and PHP Installation | 643 | ||
17.6.1 XAMPP Installation | 644 | ||
17.6.2 Running XAMPP | 644 | ||
17.6.3 Testing Your Setup | 645 | ||
17.6.4 Running the Examples Using Apache HTTP Server | 645 | ||
17.7 Microsoft IIS Express and WebMatrix | 646 | ||
17.7.1 Installing and Running IIS Express | 646 | ||
17.7.2 Installing and Running WebMatrix | 646 | ||
17.7.3 Running the Client-Side Examples Using IIS Express | 646 | ||
17.7.4 Running the PHP Examples Using IIS Express | 647 | ||
18 Database: SQL, MySQL, LINQ and Java DB | 649 | ||
18.1 Introduction | 650 | ||
18.2 Relational Databases | 650 | ||
18.3 Relational Database Overview: A books Database | 652 | ||
18.4 \rSQL | 655 | ||
18.4.1 Basic SELECT Query | 656 | ||
18.4.2 WHERE Clause | 656 | ||
18.4.3 ORDER BY Clause | 658 | ||
18.4.4 Merging Data from Multiple Tables: INNER JOIN | 660 | ||
18.4.5 INSERT Statement | 661 | ||
18.4.6 UPDATE Statement | 663 | ||
18.4.7 DELETE Statement | 663 | ||
18.5 MySQL | 664 | ||
18.5.1 Instructions for Setting Up a MySQL User Account | 665 | ||
18.5.2 Creating Databases in MySQL | 666 | ||
18.6 (Optional) Microsoft Language Integrate Query (LINQ) | 666 | ||
18.6.1 Querying an Array of int Values Using LINQ | 667 | ||
18.6.2 Querying an Array of Employee Objects Using LINQ | 669 | ||
18.6.3 Querying a Generic Collection Using LINQ | 674 | ||
18.7 (Optional) LINQ to SQL | 676 | ||
18.8 (Optional) Querying a Database with LINQ | 677 | ||
18.8.1 Creating LINQ to SQL Classes | 677 | ||
18.8.2 Data Bindings Between Controls and the LINQ to SQL Classes | 680 | ||
18.9 (Optional) Dynamically Binding LINQ to SQL Query Results | 684 | ||
18.9.1 Creating the Display Query Results GUI | 684 | ||
18.9.2 Coding the Display Query Results Application | 686 | ||
18.10 Java DB/Apache Derby | 688 | ||
19 \rPHP | 696 | ||
19.1 Introduction | 697 | ||
19.2 Simple PHP Program 698 | 698 | ||
19.3 Converting Between Data Types | 699 | ||
19.4 Arithmetic Operators | 702 | ||
19.5 Initializing and Manipulating Arrays | 706 | ||
19.6 String Comparisons | 709 | ||
19.7 String Processing with Regular Expressions | 710 | ||
19.7.1 Searching for Expressions | 712 | ||
19.7.2 Representing Patterns | 712 | ||
19.7.3 Finding Matches | 713 | ||
19.7.4 Character Classes | 713 | ||
19.7.5 Finding Multiple Instances of a Pattern | 714 | ||
19.8 Form Processing and Business Logic | 714 | ||
19.8.1 Superglobal Arrays | 714 | ||
19.8.2 Using PHP to Process HTML5 Forms | 715 | ||
19.9 Reading from a Database | 719 | ||
19.10 Using Cookies | 723 | ||
19.11 Dynamic Content | 726 | ||
19.12 Web Resources | 734 | ||
20 Web App Development with ASP.NET in C | 740 | ||
20.1 Introduction | 741 | ||
20.2 Web Basics | 742 | ||
20.3 Multitier Application Architecture | 743 | ||
20.4 Your First ASP.NET Application | 745 | ||
20.4.1 Building the WebTime Application | 747 | ||
20.4.2 Examining WebTime.aspx’s Code-Behind File | 756 | ||
20.5 Standard Web Controls: Designing a Form | 756 | ||
20.6 Validation Controls | 761 | ||
20.7 Session Tracking | 767 | ||
20.7.1 Cookies | 768 | ||
20.7.2 Session Tracking with HttpSessionState | 769 | ||
20.7.3 Options.aspx: Selecting a Programming Language | 772 | ||
20.7.4 Recommendations.aspx: Displaying Recommendations Basedon Session Values | 775 | ||
20.8 Case Study: Database-Driven ASP.NET Guestbook | 777 | ||
20.8.1 Building a Web Form that Displays Data from a Database | 779 | ||
20.8.2 Modifying the Code-Behind File for the Guestbook Application | 782 | ||
20.9 Case Study Introduction: ASP.NET AJAX | 784 | ||
20.10 Case Study Introduction: Password-Protected Books Database Application | 784 | ||
21 Web App Development with ASP.NET in C | 790 | ||
21.1 Introduction | 791 | ||
21.2 Case Study: Password-Protected Books Database Application | 791 | ||
21.2.1 Examining the ASP.NET Web Site Template | 792 | ||
21.2.2 Test-Driving the Completed Application | 794 | ||
21.2.3 Configuring the Website | 796 | ||
21.2.4 Modifying the Default.aspx and About.aspx Pages | 799 | ||
21.2.5 Creating a Content Page That Only Authenticated Users Can Access | 800 | ||
21.2.6 Linking from the Default.aspx Page to the Books.aspx Page | 801 | ||
21.2.7 Modifying the Master Page (Site.master) | 802 | ||
21.2.8 Customizing the Password-Protected Books.aspx Page | 804 | ||
21.3 ASP.NET Ajax | 809 | ||
21.3.1 Traditional Web Applications | 809 | ||
21.3.2 Ajax Web Applications | 810 | ||
21.3.3 Testing an ASP.NET Ajax Application | 811 | ||
21.3.4 The ASP.NET Ajax Control Toolkit | 812 | ||
21.3.5 Using Controls from the Ajax Control Toolkit | 813 | ||
22 Web Services in C | 821 | ||
22.1 Introduction | 822 | ||
22.2 WCF Services Basics | 823 | ||
22.3 Simple Object Access Protocol (SOAP) | 823 | ||
22.4 Representational State Transfer (REST) | 824 | ||
22.5 JavaScript Object Notation (JSON) | 824 | ||
22.6 Publishing and Consuming SOAP-Based WCF Web Services | 825 | ||
22.6.1 Creating a WCF Web Service | 825 | ||
22.6.2 Code for the WelcomeSOAPXMLService | 825 | ||
22.6.3 Building a SOAP WCF Web Service | 826 | ||
22.6.4 Deploying the WelcomeSOAPXMLService | 828 | ||
22.6.5 Creating a Client to Consume the WelcomeSOAPXMLService | 829 | ||
22.6.6 Consuming the WelcomeSOAPXMLService | 831 | ||
22.7 Publishing and Consuming REST-Based XML Web Services | 833 | ||
22.7.1 HTTP get and post Requests | 833 | ||
22.7.2 Creating a REST-Based XML WCF Web Service | 833 | ||
22.7.3 Consuming a REST-Based XML WCF Web Service | 836 | ||
22.8 Publishing and Consuming REST-Based JSON Web Services 837 | 837 | ||
22.8.1 Creating a REST-Based JSON WCF Web Service | 837 | ||
22.8.2 Consuming a REST-Based JSON WCF Web Service | 839 | ||
22.9 Blackjack Web Service: Using Session Tracking in a SOAP-BasedWCF Web Service | 841 | ||
22.9.1 Creating a Blackjack Web Service | 841 | ||
22.9.2 Consuming the Blackjack Web Service | 846 | ||
22.10 Airline Reservation Web Service: Database Access and Invoking aService from ASP.NET | 855 | ||
22.11 Equation Generator: Returning User-Defined Types | 859 | ||
22.11.1 Creating the REST-Based XML EquationGenerator Web Service | 862 | ||
22.11.2 Consuming the REST-Based XML EquationGeneratorWeb Service | 863 | ||
22.11.3 Creating the REST-Based JSON WCF EquationGeneratorWeb Service | 867 | ||
22.11.4 Consuming the REST-Based JSON WCF EquationGeneratorWeb Service | 867 | ||
22.12 Web Resources | 871 | ||
23 Web App Development with ASP.NET inVisual Basic | 879 | ||
23.1 Introduction | 880 | ||
23.2 Web Basics | 881 | ||
23.3 Multitier Application Architecture | 882 | ||
23.4 Your First ASP.NET Application | 884 | ||
23.4.1 Building the WebTime Application | 886 | ||
23.4.2 Examining WebTime.aspx’s Code-Behind File | 895 | ||
23.5 Standard Web Controls: Designing a Form | 896 | ||
23.6 Validation Controls | 901 | ||
23.7 Session Tracking | 907 | ||
23.7.1 Cookies | 908 | ||
23.7.2 Session Tracking with HttpSessionState | 909 | ||
23.7.3 Options.aspx: Selecting a Programming Language | 911 | ||
23.7.4 Recommendations.aspx: Displaying Recommendations Basedon Session Values | 915 | ||
23.8 Case Study: Database-Driven ASP.NET Guestbook | 917 | ||
23.8.1 Building a Web Form that Displays Data from a Database | 919 | ||
23.8.2 Modifying the Code-Behind File for the Guestbook Application | 923 | ||
23.9 Online Case Study: ASP.NET AJAX | 924 | ||
23.10 Online Case Study: Password-Protected Books Database Application\r | 924 | ||
A HTML Special Characters | 930 | ||
B HTML Colors | 931 | ||
C JavaScript Operator Precedence Chart | 934 | ||
D ASCII Character Set | 936 | ||
Index | 937 | ||
Symbols | 937 | ||
Numerics | 937 | ||
A | 937 | ||
B | 938 | ||
C | 939 | ||
D | 941 | ||
E | 942 | ||
F | 943 | ||
G | 943 | ||
H | 944 | ||
I | 945 | ||
J | 946 | ||
K | 946 | ||
L | 946 | ||
M | 947 | ||
N | 947 | ||
O | 948 | ||
P | 948 | ||
Q | 949 | ||
R | 949 | ||
S | 950 | ||
T | 952 | ||
U | 953 | ||
V | 954 | ||
W | 954 | ||
X | 955 | ||
Y | 955 | ||
Z | 955 |