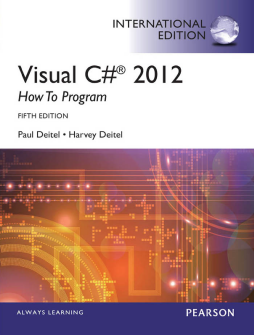
Additional Information
Book Details
Abstract
Appropriate for all basic-to-intermediate level courses in Visual C# 2012 programming.
Created by world-renowned programming instructors Paul and Harvey Deitel, Visual C# 2012 How to Program, Fifth Edition introduces all facets of the C# 2012 language hands-on, through hundreds of working programs. This book has been thoroughly updated to reflect the major innovations Microsoft has incorporated in Visual C# 2012; all discussions and sample code have been carefully audited against the newest Visual C# language specification.
Students begin by getting comfortable with the C# Express 2012 IDE and basic Visual C# syntax. Next, they build their skills one step at a time, mastering control structures, classes, objects, methods, variables, arrays, and the core techniques of object-oriented programming. With this strong foundation in place, the Deitels introduce more sophisticated techniques, including searching, sorting, data structures, generics, and collections. Throughout, the authors show students how to make the most of Microsoft’s Visual Studio tools. A series of appendices provide essential programming reference material.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Contents | 7 | ||
Preface | 19 | ||
Before You Begin | 35 | ||
1 Introduction to Computers, the Internet and Visual C | 39 | ||
1.1 Introduction | 40 | ||
1.2 Hardware and Moore’s Law | 40 | ||
1.3 Data Hierarchy | 41 | ||
1.4 Computer Organization | 44 | ||
1.5 Machine Languages, Assembly Languages and High-Level Languages | 45 | ||
1.6 Object Technology | 46 | ||
1.7 Internet and World Wide Web | 48 | ||
1.8 C | 50 | ||
1.8.1 Object-Oriented Programming | 50 | ||
1.8.2 Event-Driven Programming | 50 | ||
1.8.3 Visual Programming | 50 | ||
1.8.4 An International Standard; Other C | 50 | ||
1.8.5 Internet and Web Programming | 51 | ||
1.8.6 Introducing async/await | 51 | ||
1.8.7 Other Key Contemporary Programming Languages | 51 | ||
1.9 Microsoft’s .NET | 52 | ||
1.9.1 .NET Framework | 52 | ||
1.9.2 Common Language Runtime | 53 | ||
1.9.3 Platform Independence | 53 | ||
1.9.4 Language Interoperability | 53 | ||
1.10 Microsoft’s Windows® Operating System | 54 | ||
1.11 Windows Phone 8 for Smartphones | 55 | ||
1.11.1 Selling Your Apps in the Windows Phone Marketplace | 56 | ||
1.11.2 Free vs. Paid Apps | 56 | ||
1.11.3 Testing Your Windows Phone Apps | 56 | ||
1.12 Windows Azure™ and Cloud Computing | 57 | ||
1.13 Visual Studio Express 2012 Integrated Development Environment | 57 | ||
1.14 Painter Test-Drive in Visual Studio Express 2012 for Windows Desktop | 57 | ||
1.15 Painter Test-Drive in Visual Studio Express 2012 for Windows 8 | 61 | ||
2 Dive Into® Visual Studio Express 2012 for Windows Desktop | 71 | ||
2.1 Introduction | 72 | ||
2.2 Overview of the Visual Studio Express 2012 IDE | 72 | ||
2.3 Menu Bar and Toolbar | 77 | ||
2.4 Navigating the Visual Studio IDE | 79 | ||
2.4.1 Solution Explorer | 81 | ||
2.4.2 Toolbox | 82 | ||
2.4.3 Properties Window | 82 | ||
2.5 Using Help | 84 | ||
2.6 Using Visual App Development to Create a Simple App that Displays Text and an Image | 85 | ||
2.7 Wrap-Up | 95 | ||
2.8 Web Resources | 96 | ||
3 Introduction to C | 103 | ||
3.1 Introduction | 104 | ||
3.2 A Simple C | 104 | ||
3.3 Creating a Simple App in Visual Studio | 110 | ||
3.4 Modifying Your Simple C | 115 | ||
3.5 Formatting Text with Console.Write and Console.WriteLine | 118 | ||
3.6 Another C | 119 | ||
3.7 Memory Concepts | 123 | ||
3.8 Arithmetic | 124 | ||
3.9 Decision Making: Equality and Relational Operators | 128 | ||
3.10 Wrap-Up | 132 | ||
4 Introduction to Classes, Objects, Methods and strings | 144 | ||
4.1 Introduction | 145 | ||
4.2 Classes, Objects, Methods, Properties and Instance Variables | 145 | ||
4.3 Declaring a Class with a Method and Instantiating an Object of a Class | 146 | ||
4.4 Declaring a Method with a Parameter | 151 | ||
4.5 Instance Variables and Properties | 154 | ||
4.6 UML Class Diagram with a Property | 159 | ||
4.7 Software Engineering with Properties and set and get Accessors | 159 | ||
4.8 Auto-Implemented Properties | 161 | ||
4.9 Value Types vs. Reference Types | 161 | ||
4.10 Initializing Objects with Constructors | 163 | ||
4.11 Floating-Point Numbers and Type decimal | 166 | ||
4.12 Wrap-Up | 172 | ||
5 Control Statements: Part 1 | 180 | ||
5.1 Introduction | 181 | ||
5.2 Algorithms | 181 | ||
5.3 Pseudocode | 182 | ||
5.4 Control Structures | 182 | ||
5.5 if Single-Selection Statement | 184 | ||
5.6 if…else Double-Selection Statement | 186 | ||
5.7 while Repetition Statement | 190 | ||
5.8 Formulating Algorithms: Counter-Controlled Repetition | 192 | ||
5.9 Formulating Algorithms: Sentinel-Controlled Repetition | 196 | ||
5.10 Formulating Algorithms: Nested Control Statements | 204 | ||
5.11 Compound Assignment Operators | 209 | ||
5.12 Increment and Decrement Operators | 209 | ||
5.13 Simple Types | 212 | ||
5.14 Wrap-Up | 213 | ||
6 Control Statements: Part 2 | 227 | ||
6.1 Introduction | 228 | ||
6.2 Essentials of Counter-Controlled Repetition | 228 | ||
6.3 for Repetition Statement | 229 | ||
6.4 Examples Using the for Statement | 233 | ||
6.5 do…while Repetition Statement | 237 | ||
6.6 switch Multiple-Selection Statement | 239 | ||
6.7 break and continue Statements | 247 | ||
6.8 Logical Operators | 249 | ||
6.9 Structured-Programming Summary | 254 | ||
6.10 Wrap-Up | 259 | ||
7 Methods: A Deeper Look | 269 | ||
7.1 Introduction | 270 | ||
7.2 Packaging Code in C | 270 | ||
7.3 static Methods, static Variables and Class Math | 272 | ||
7.4 Declaring Methods with Multiple Parameters | 274 | ||
7.5 Notes on Declaring and Using Methods | 278 | ||
7.6 Method-Call Stack and Activation Records | 279 | ||
7.7 Argument Promotion and Casting | 280 | ||
7.8 The .NET Framework Class Library | 281 | ||
7.9 Case Study: Random-Number Generation | 283 | ||
7.9.1 Scaling and Shifting Random Numbers | 287 | ||
7.9.2 Random-Number Repeatability for Testing and Debugging | 288 | ||
7.10 Case Study: A Game of Chance; Introducing Enumerations | 288 | ||
7.11 Scope of Declarations | 293 | ||
7.12 Method Overloading | 296 | ||
7.13 Optional Parameters | 298 | ||
7.14 Named Parameters | 300 | ||
7.15 Recursion | 301 | ||
7.16 Passing Arguments: Pass-by-Value vs. Pass-by-Reference | 304 | ||
7.17 Wrap-Up | 307 | ||
8 Arrays; Introduction to Exception Handling | 323 | ||
8.1 Introduction | 324 | ||
8.2 Arrays | 324 | ||
8.3 Declaring and Creating Arrays | 326 | ||
8.4 Examples Using Arrays | 327 | ||
8.4.1 Creating and Initializing an Array | 327 | ||
8.4.2 Using an Array Initializer | 328 | ||
8.4.3 Calculating a Value to Store in Each Array Element | 329 | ||
8.4.4 Summing the Elements of an Array | 330 | ||
8.4.5 Using Bar Charts to Display Array Data Graphically | 331 | ||
8.4.6 Using the Elements of an Array as Counters | 333 | ||
8.4.7 Using Arrays to Analyze Survey Results; Introduction to Exception Handling | 334 | ||
8.5 Case Study: Card Shuffling and Dealing Simulation | 337 | ||
8.6 foreach Statement | 341 | ||
8.7 Passing Arrays and Array Elements to Methods | 343 | ||
8.8 Passing Arrays by Value and by Reference | 345 | ||
8.9 Case Study: GradeBook Using an Array to Store Grades | 349 | ||
8.10 Multidimensional Arrays | 354 | ||
8.11 Case Study: GradeBook Using a Rectangular Array | 359 | ||
8.12 Variable-Length Argument Lists | 365 | ||
8.13 Using Command-Line Arguments | 367 | ||
8.14 Wrap-Up | 369 | ||
9 Introduction to LINQ and the List Collection | 389 | ||
9.1 Introduction | 390 | ||
9.2 Querying an Array of int Values Using LINQ | 391 | ||
9.3 Querying an Array of Employee Objects Using LINQ | 395 | ||
9.4 Introduction to Collections | 400 | ||
9.5 Querying a Generic Collection Using LINQ | 403 | ||
9.6 Wrap-Up | 405 | ||
9.7 Deitel LINQ Resource Center | 405 | ||
10 Classes and Objects: A Deeper Look | 409 | ||
10.1 Introduction | 410 | ||
10.2 Time Class Case Study | 410 | ||
10.3 Controlling Access to Members | 414 | ||
10.4 Referring to the Current Object’s Members with the this Reference | 415 | ||
10.5 Time Class Case Study: Overloaded Constructors | 417 | ||
10.6 Default and Parameterless Constructors | 423 | ||
10.7 Composition | 424 | ||
10.8 Garbage Collection and Destructors | 427 | ||
10.9 static Class Members | 428 | ||
10.10 readonly Instance Variables | 431 | ||
10.11 Data Abstraction and Encapsulation | 432 | ||
10.12 Class View and Object Browser | 434 | ||
10.13 Object Initializers | 436 | ||
10.14 Wrap-Up | 436 | ||
11 Object-Oriented Programming: Inheritance | 443 | ||
11.1 Introduction | 444 | ||
11.2 Base Classes and Derived Classes | 445 | ||
11.3 protected Members | 447 | ||
11.4 Relationship between Base Classes and Derived Classes | 448 | ||
11.4.1 Creating and Using a CommissionEmployee Class | 448 | ||
11.4.2 Creating a BasePlusCommissionEmployee Class without Using Inheritance | 453 | ||
11.4.3 Creating a CommissionEmployee–BasePlusCommissionEmployee Inheritance Hierarchy | 458 | ||
11.4.4 CommissionEmployee–BasePlusCommissionEmployee Inheritance Hierarchy Using protected Instance Variables | 461 | ||
11.4.5 CommissionEmployee–BasePlusCommissionEmployee Inheritance Hierarchy Using private Instance Variables | 466 | ||
11.5 Constructors in Derived Classes | 471 | ||
11.6 Software Engineering with Inheritance | 472 | ||
11.7 Class object | 472 | ||
11.8 Wrap-Up | 473 | ||
12 OOP: Polymorphism, Interfaces and Operator Overloading | 479 | ||
12.1 Introduction | 480 | ||
12.2 Polymorphism Examples | 482 | ||
12.3 Demonstrating Polymorphic Behavior | 483 | ||
12.4 Abstract Classes and Methods | 486 | ||
12.5 Case Study: Payroll System Using Polymorphism | 488 | ||
12.5.1 Creating Abstract Base Class Employee | 489 | ||
12.5.2 Creating Concrete Derived Class SalariedEmployee | 491 | ||
12.5.3 Creating Concrete Derived Class HourlyEmployee | 493 | ||
12.5.4 Creating Concrete Derived Class CommissionEmployee | 495 | ||
12.5.5 Creating Indirect Concrete Derived Class BasePlusCommissionEmployee | 496 | ||
12.5.6 Polymorphic Processing, Operator is and Downcasting | 498 | ||
12.5.7 Summary of the Allowed Assignments Between Base-Class and Derived-Class Variables | 503 | ||
12.6 sealed Methods and Classes | 504 | ||
12.7 Case Study: Creating and Using Interfaces | 504 | ||
12.7.1 Developing an IPayable Hierarchy | 506 | ||
12.7.2 Declaring Interface IPayable | 507 | ||
12.7.3 Creating Class Invoice | 507 | ||
12.7.4 Modifying Class Employee to Implement Interface IPayable | 509 | ||
12.7.5 Modifying Class SalariedEmployee for Use with IPayable | 511 | ||
12.7.6 Using Interface IPayable to Process Invoices and Employees Polymorphically | 512 | ||
12.7.7 Common Interfaces of the .NET Framework Class Library | 514 | ||
12.8 Operator Overloading | 515 | ||
12.9 Wrap-Up | 518 | ||
13 Exception Handling: A Deeper Look | 524 | ||
13.1 Introduction | 525 | ||
13.2 Example: Divide by Zero without Exception Handling | 526 | ||
13.3 Example: Handling DivideByZeroExceptions and FormatExceptions | 529 | ||
13.3.1 Enclosing Code in a try Block | 531 | ||
13.3.2 Catching Exceptions | 531 | ||
13.3.3 Uncaught Exceptions | 532 | ||
13.3.4 Termination Model of Exception Handling | 533 | ||
13.3.5 Flow of Control When Exceptions Occur | 533 | ||
13.4 .NET Exception Hierarchy | 534 | ||
13.4.1 Class SystemException | 534 | ||
13.4.2 Determining Which Exceptions a Method Throws | 535 | ||
13.5 finally Block | 535 | ||
13.6 The using Statement | 542 | ||
13.7 Exception Properties | 543 | ||
13.8 User-Defined Exception Classes | 547 | ||
13.9 Wrap-Up | 551 | ||
14 Graphical User Interfaces with Windows Forms: Part 1 | 556 | ||
14.1 Introduction | 557 | ||
14.2 Windows Forms | 558 | ||
14.3 Event Handling | 560 | ||
14.3.1 A Simple Event-Driven GUI | 560 | ||
14.3.2 Auto-Generated GUI Code | 562 | ||
14.3.3 Delegates and the Event-Handling Mechanism | 564 | ||
14.3.4 Another Way to Create Event Handlers | 565 | ||
14.3.5 Locating Event Information | 566 | ||
14.4 Control Properties and Layout | 567 | ||
14.5 Labels, TextBoxes and Buttons | 571 | ||
14.6 GroupBoxes and Panels | 574 | ||
14.7 CheckBoxes and RadioButtons | 577 | ||
14.8 PictureBoxes | 585 | ||
14.9 ToolTips | 587 | ||
14.10 NumericUpDown Control | 589 | ||
14.11 Mouse-Event Handling | 591 | ||
14.12 Keyboard-Event Handling | 594 | ||
14.13 Wrap-Up | 597 | ||
15 Graphical User Interfaces with Windows Forms: Part 2 | 607 | ||
15.1 Introduction | 608 | ||
15.2 Menus | 608 | ||
15.3 MonthCalendar Control | 617 | ||
15.4 DateTimePicker Control | 618 | ||
15.5 LinkLabel Control | 621 | ||
15.6 ListBox Control | 625 | ||
15.7 CheckedListBox Control | 629 | ||
15.8 ComboBox Control | 632 | ||
15.9 TreeView Control | 636 | ||
15.10 ListView Control | 641 | ||
15.11 TabControl Control | 647 | ||
15.12 Multiple Document Interface (MDI) Windows | 652 | ||
15.13 Visual Inheritance | 659 | ||
15.14 User-Defined Controls | 664 | ||
15.15 Wrap-Up | 668 | ||
16 Strings and Characters: A Deeper Look | 676 | ||
16.1 Introduction | 677 | ||
16.2 Fundamentals of Characters and Strings | 678 | ||
16.3 string Constructors | 679 | ||
16.4 string Indexer, Length Property and CopyTo Method | 680 | ||
16.5 Comparing strings | 681 | ||
16.6 Locating Characters and Substrings in strings | 684 | ||
16.7 Extracting Substrings from strings | 687 | ||
16.8 Concatenating strings | 688 | ||
16.9 Miscellaneous string Methods | 689 | ||
16.10 Class StringBuilder | 690 | ||
16.11 Length and Capacity Properties, EnsureCapacity Method and Indexer of Class StringBuilder | 691 | ||
16.12 Append and AppendFormat Methods of Class StringBuilder | 693 | ||
16.13 Insert, Remove and Replace Methods of Class StringBuilder | 695 | ||
16.14 Char Methods | 698 | ||
16.15 (Online) Introduction to Regular Expressions | 700 | ||
16.16 Wrap-Up | 701 | ||
17 Files and Streams | 707 | ||
17.1 Introduction | 708 | ||
17.2 Data Hierarchy | 708 | ||
17.3 Files and Streams | 710 | ||
17.4 Classes File and Directory | 711 | ||
17.5 Creating a Sequential-Access Text | 720 | ||
17.6 Reading Data from a Sequential-Access Text File | 729 | ||
17.7 Case Study: Credit Inquiry Program | 733 | ||
17.8 Serialization | 739 | ||
17.9 Creating a Sequential-Access File Using Object Serialization | 740 | ||
17.10 Reading and Deserializing Data from a Binary File | 744 | ||
17.11 Wrap-Up | 746 | ||
18 Searching and Sorting | 753 | ||
18.1 Introduction | 754 | ||
18.2 Searching Algorithms | 755 | ||
18.2.1 Linear Search | 755 | ||
18.2.2 Binary Search | 759 | ||
18.3 Sorting Algorithms | 764 | ||
18.3.1 Selection Sort | 764 | ||
18.3.2 Insertion Sort | 768 | ||
18.3.3 Merge Sort | 772 | ||
18.4 Summary of the Efficiency of Searching and Sorting Algorithms | 778 | ||
18.5 Wrap-Up | 779 | ||
19 Data Structures | 784 | ||
19.1 Introduction | 785 | ||
19.2 Simple-Type structs, Boxing and Unboxing | 785 | ||
19.3 Self-Referential Classes | 786 | ||
19.4 Linked Lists | 787 | ||
19.5 Stacks | 800 | ||
19.6 Queues | 804 | ||
19.7 Trees | 807 | ||
19.7.1 Binary Search Tree of Integer Values | 808 | ||
19.7.2 Binary Search Tree of IComparable Objects | 815 | ||
19.8 Wrap-Up | 820 | ||
20 Generics | 827 | ||
20.1 Introduction | 828 | ||
20.2 Motivation for Generic Methods | 829 | ||
20.3 Generic-Method Implementation | 831 | ||
20.4 Type Constraints | 834 | ||
20.5 Overloading Generic Methods | 836 | ||
20.6 Generic Classes | 837 | ||
20.7 Wrap-Up | 846 | ||
21 Collections | 852 | ||
21.1 Introduction | 853 | ||
21.2 Collections Overview | 853 | ||
21.3 Class Array and Enumerators | 856 | ||
21.4 Nongeneric Collections | 859 | ||
21.4.1 Class ArrayList | 859 | ||
21.4.2 Class Stack | 864 | ||
21.4.3 Class Hashtable | 866 | ||
21.5 Generic Collections | 871 | ||
21.5.1 Generic Class SortedDictionary | 872 | ||
21.5.2 Generic Class LinkedList | 874 | ||
21.6 Covariance and Contravariance for Generic Types | 878 | ||
21.7 Wrap-Up | 881 | ||
22 Databases and LINQ | 887 | ||
22.1 Introduction | 888 | ||
22.2 Relational Databases | 889 | ||
22.3 A Books Database | 890 | ||
22.4 LINQ to Entities and the ADO.NET Entity Framework | 894 | ||
22.5 Querying a Database with LINQ | 895 | ||
22.5.1 Creating the ADO.NET Entity Data Model Class Library | 896 | ||
22.5.2 Creating a Windows Forms Project and Configuring It to Use the Entity Data Model | 900 | ||
22.5.3 Data Bindings Between Controls and the Entity Data Model | 902 | ||
22.6 Dynamically Binding Query Results | 907 | ||
22.6.1 Creating the Display Query Results GUI | 908 | ||
22.6.2 Coding the Display Query Results App | 909 | ||
22.7 Retrieving Data from Multiple Tables with LINQ | 912 | ||
22.8 Creating a Master/Detail View App | 917 | ||
22.8.1 Creating the Master/Detail GUI | 918 | ||
22.8.2 Coding the Master/Detail App | 919 | ||
22.9 Address Book Case Study | 921 | ||
22.9.1 Creating the Address Book App’s GUI | 922 | ||
22.9.2 Coding the Address Book App | 923 | ||
22.10 Tools and Web Resources | 927 | ||
22.11 Wrap-Up | 927 | ||
23 Web App Development with ASP.NET | 935 | ||
23.1 Introduction | 936 | ||
23.2 Web Basics | 937 | ||
23.3 Multitier App Architecture | 938 | ||
23.4 Your First Web App | 940 | ||
23.4.1 Building the WebTime App | 942 | ||
23.4.2 Examining WebTime.aspx’s Code-Behind File | 951 | ||
23.5 Standard Web Controls: Designing a Form | 952 | ||
23.6 Validation Controls | 956 | ||
23.7 Session Tracking | 963 | ||
23.7.1 Cookies | 964 | ||
23.7.2 Session Tracking with HttpSessionState | 965 | ||
23.7.3 Options.aspx: Selecting a Programming Language | 966 | ||
23.7.4 Recommendations.aspx: Displaying Recommendations Based on Session Values | 970 | ||
23.8 Case Study: Database-Driven ASP.NET Guestbook | 971 | ||
23.8.1 Building a Web Form that Displays Data from a Database | 973 | ||
23.8.2 Modifying the Code-Behind File for the Guestbook App | 978 | ||
23.9 Online Case Study: ASP.NET AJAX | 979 | ||
23.10 Online Case Study: Password-Protected Books Database App | 980 | ||
23.11 Wrap-Up | 980 | ||
Chapters on the Web | 987 | ||
A: Operator Precedence Chart | 988 | ||
B: Simple Types | 990 | ||
C: ASCII Character Set | 992 | ||
Appendices on the Web | 993 | ||
Index | 995 | ||
A | 995 | ||
B | 996 | ||
C | 997 | ||
D | 1000 | ||
E | 1001 | ||
F | 1002 | ||
G | 1003 | ||
H | 1004 | ||
I | 1004 | ||
J | 1005 | ||
K | 1005 | ||
L | 1006 | ||
M | 1007 | ||
N | 1008 | ||
O | 1009 | ||
P | 1010 | ||
Q | 1011 | ||
R | 1011 | ||
S | 1012 | ||
T | 1015 | ||
U | 1016 | ||
V | 1016 | ||
W | 1017 | ||
X | 1017 | ||
Y | 1017 | ||
Z | 1017 |