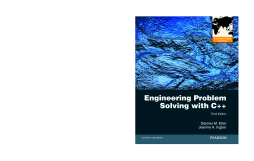
Additional Information
Book Details
Abstract
For one/two semester courses in Engineering and Computer Science at the freshman/sophomore level.
This text is a clear, concise introduction to problem solving and the C++ programming language. The authors proven five-step problem solving methodology is presented and then incorporated in every chapter of the text. Outstanding engineering and scientific applications are used throughout; all applications are centered around the theme of engineering challenges in the 21st century.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover\r | Cover | ||
Contents | 4 | ||
Preface | 11 | ||
Chapter 1: Introduction to Computing and Engineering Problem Solving\r | 18 | ||
1.1 Historical Perspective\r | 19 | ||
1.2 Recent Engineering Achievements\r | 23 | ||
Changing Engineering Environment | 25 | ||
1.3 Computing Systems\r | 27 | ||
Computer Hardware | 27 | ||
Computer Software | 28 | ||
1.4 Data Representation and Storage\r | 32 | ||
Number Systems | 33 | ||
Data Types and Storage | 39 | ||
1.5 An Engineering Problem-Solving Methodology\r | 42 | ||
Summary\r | 45 | ||
Chapter 2: Simple C++ Programs\r | 50 | ||
Engineering Challenge: Vehicle Performance\r | 50 | ||
2.1 Program Structure\r | 51 | ||
2.2 Constants and Variables\r | 55 | ||
Scientific Notation | 57 | ||
Numeric Data Types | 58 | ||
Boolean Data Type | 60 | ||
Character Data Type | 60 | ||
String Data | 62 | ||
Symbolic Constants | 63 | ||
2.3 C++ Classes\r | 64 | ||
Class Declaration | 64 | ||
Class Implementation | 65 | ||
2.4 C++ Operators\r | 68 | ||
Assignment Operator | 68 | ||
Arithmetic Operators | 70 | ||
Precedence of Operators | 72 | ||
Overflow and Underflow | 74 | ||
Increment and Decrement Operators | 75 | ||
Abbreviated Assignment Operators | 76 | ||
2.5 Standard Input and Output\r | 77 | ||
The cout Object\r | 77 | ||
Stream Objects | 78 | ||
Manipulators | 80 | ||
The cin Object\r | 83 | ||
2.6 Building C++ Solutions with IDEs: NetBeans\r | 85 | ||
NetBeans | 85 | ||
2.7 Basic Functions Included in the C++ Standard Library\r | 92 | ||
Elementary Math Functions | 93 | ||
Trigonometric Functions | 94 | ||
Hyperbolic Functions\r | 96 | ||
Character Functions | 97 | ||
2.8 Problem Solving Applied: Velocity Computation\r | 97 | ||
2.9 System Limitations\r | 101 | ||
Summary\r | 102 | ||
Chapter 3: Control Structures: Selection\r | 110 | ||
Engineering Challenge: Global Change\r | 110 | ||
3.1 Algorithm Development\r | 111 | ||
Top-Down Design | 111 | ||
3.2 Structured Programming\r | 113 | ||
Pseudocode | 113 | ||
Evaluation of Alternative Solutions | 115 | ||
3.3 Conditional Expressions\r | 115 | ||
Relational Operators | 115 | ||
Logical Operators | 116 | ||
Precedence and Associativity | 119 | ||
3.4 Selection Statements: if Statement\r | 120 | ||
Simple if Statements | 120 | ||
if/else Statement | 123 | ||
3.5 Numerical Technique: Linear Interpolation\r | 127 | ||
3.6 Problem Solving Applied: Freezing Temperature of Seawater\r | 131 | ||
3.7 Selection Statements: switch Statement\r | 135 | ||
3.8 Building C++ Solutions with IDEs: NetBeans\r | 138 | ||
NetBeans | 138 | ||
3.9 Defining Operators for Programmer-Defined Data Types\r | 144 | ||
Summary\r | 149 | ||
Chapter 4: Control Structures: Repetition\r | 154 | ||
Engineering Challenge: Data Collection\r | 154 | ||
4.1 Algorithm Development\r | 155 | ||
Pseudocode and Flowchart Description | 156 | ||
4.2 Repetition Structures\r | 156 | ||
while Loop\r | 157 | ||
do/while Loop\r | 161 | ||
for Loop\r | 164 | ||
4.3 Problem Solving Applied: GPS\r | 169 | ||
4.4 break and continue Statements\r | 173 | ||
4.5 Structuring Input Loops\r | 174 | ||
Counter-Controlled Loops | 174 | ||
Sentinel-Controlled Loop | 176 | ||
End-Of-Data Loop | 177 | ||
4.6 Problem Solving Applied: Weather Balloons\r | 179 | ||
4.7 Building C++ Solutions with IDEs: Microsoft Visual C++\r | 184 | ||
Microsoft Visual C | 186 | ||
Summary\r | 190 | ||
Chapter 5: Working with Data Files\r | 196 | ||
Engineering Challenge: Weather Prediction\r | 196 | ||
5.1 Defining File Streams\r | 197 | ||
Stream Class Hierarchy | 197 | ||
ifstream Class\r | 200 | ||
ofstream Class\r | 201 | ||
5.2 Reading Data Files\r | 203 | ||
Specified Number of Records | 204 | ||
Trailer or Sentinel Signals | 206 | ||
End-of-File | 208 | ||
5.3 Generating a Data File\r | 211 | ||
5.4 Problem Solving Applied: Data Filters.Modifying an HTML File\r | 214 | ||
5.5 Error Checking\r | 218 | ||
The Stream State\r | 219 | ||
5.6 Numerical Technique: Linear Modeling\r | 224 | ||
5.7 Problem Solving Applied: Ozone Measurements\r | 227 | ||
Summary\r | 233 | ||
Chapter 6: Modular Programming with Functions\r | 240 | ||
Engineering Challenge: Simulation\r | 240 | ||
6.1 Modularity\r | 241 | ||
6.2 Programmer-Defined Functions\r | 244 | ||
Function Definition | 247 | ||
Solution 1 | 250 | ||
Solution 2 | 251 | ||
Function Prototype | 254 | ||
6.3 Parameter Passing\r | 255 | ||
Pass by Value | 256 | ||
Pass by Reference | 258 | ||
Storage Class and Scope | 264 | ||
6.4 Problem Solving Applied: Calculating a Center of Gravity\r | 266 | ||
6.5 Random Numbers\r | 270 | ||
Integer Sequences | 270 | ||
Floating-Point Sequences | 274 | ||
6.6 Problem Solving Applied: Instrumentation Reliability\r | 275 | ||
6.7 Defining Class Methods\r | 282 | ||
Public Interface | 283 | ||
Accessor Methods | 284 | ||
Mutator Methods | 285 | ||
6.8 Problem Solving Applied: Design of Composite Materials\r | 290 | ||
Solution 1\r | 292 | ||
Solution 2\r | 293 | ||
6.9 Numerical Technique: Roots of Polynomials\r | 296 | ||
Polynomial Roots | 296 | ||
Incremental-Search Technique | 298 | ||
6.10 Problem Solving Applied: System Stability\r | 300 | ||
Newton–Raphson Method\r | 306 | ||
6.11 Numerical Technique: Integration\r | 309 | ||
Integration Using the Trapezoidal Rule | 309 | ||
Summary\r | 313 | ||
Chapter 7: One-Dimensional Arrays\r | 324 | ||
Engineering Challenge: Tsunami Warning Systems\r | 324 | ||
7.1 Arrays\r | 325 | ||
Definition and Initialization\r | 326 | ||
Pseudocode\r | 328 | ||
Computation and Output\r | 331 | ||
Function Arguments\r | 335 | ||
7.2 Problem Solving Applied: Hurricane Categories\r | 340 | ||
7.3 Statistical Measurements\r | 346 | ||
Simple Analysis | 346 | ||
Variance and Standard Deviation | 348 | ||
Custom Header Files | 351 | ||
7.4 Problem Solving Applied: Speech Signal Analysis\r | 351 | ||
7.5 Sorting and Searching Algorithms\r | 357 | ||
Selection Sort\r | 358 | ||
Search Algorithms\r | 359 | ||
Unordered Lists\r | 360 | ||
Ordered Lists\r | 360 | ||
7.6 Problem Solving Applied: Tsunami Warning Systems\r | 362 | ||
7.7 Character Strings\r | 368 | ||
C Style String Definition and I/O\r | 368 | ||
String Functions\r | 370 | ||
7.8 The string Class\r | 371 | ||
7.9 The vector class\r | 373 | ||
Parameter Passing\r | 376 | ||
7.10 Problem Solving Applied: Calculating Probabilities\r | 378 | ||
Summary\r | 391 | ||
Chapter 8: Two-Dimensional Arrays\r | 398 | ||
Engineering Challenge: Terrain Navigation\r | 398 | ||
8.1 Two-Dimensional Arrays\r | 399 | ||
Declaration and Initialization\r | 400 | ||
Computations and Output | 405 | ||
Function Arguments | 408 | ||
8.2 Problem Solving Applied: Terrain Navigation\r | 414 | ||
8.3 Two-Dimensional Arrays and the vector class\r | 419 | ||
Function Arguments\r | 422 | ||
8.4 Matrices\r | 423 | ||
Determinant\r | 423 | ||
Transpose | 424 | ||
Matrix Addition and Subtraction | 425 | ||
Matrix Multiplication | 426 | ||
8.5 Numerical Technique: Solution to Simultaneous Equations\r | 428 | ||
Graphical Interpretation | 428 | ||
Gauss Elimination | 432 | ||
8.6 Problem Solving Applied: Electrical Circuit Analysis\r | 434 | ||
8.7 Higher Dimensional Arrays\r | 440 | ||
Summary\r | 442 | ||
Chapter 9: An Introduction to Pointers\r | 450 | ||
Engineering Challenge: Weather Patterns\r | 450 | ||
9.1 Addresses and Pointers\r | 451 | ||
Address Operator | 452 | ||
Pointer Assignment | 454 | ||
Pointer Arithmetic | 457 | ||
9.2 Pointers to Array Elements\r | 460 | ||
One-Dimensional Arrays | 461 | ||
Character Strings | 463 | ||
Pointers as Function Arguments | 464 | ||
9.3 Problem Solving Applied: El Nino-Southern Oscillation Data\r | 469 | ||
9.4 Dynamic Memory Allocation\r | 472 | ||
The new Operator | 472 | ||
Dynamically Allocated Arrays | 474 | ||
The delete Operator | 474 | ||
9.5 Problem Solving Applied: Seismic Event Detection\r | 476 | ||
9.6 Common Errors Using new and delete\r | 482 | ||
9.7 Linked Data Structures\r | 484 | ||
Linked Lists | 484 | ||
Stacks | 486 | ||
Queue | 486 | ||
9.8 The C++ Standard Template Library\r | 488 | ||
The list class\r | 488 | ||
The stack class\r | 490 | ||
The queue class\r | 491 | ||
9.9 Problem Solving Applied: Concordance of a Text File\r | 493 | ||
Summary\r | 498 | ||
Chapter 10: Advanced Topics\r | 504 | ||
Engineering Challenge: Artificial Intelligence\r | 504 | ||
10.1 Generic Programming\r | 505 | ||
Function Templates \r | 506 | ||
10.2 Data Abstraction\r | 510 | ||
Overloading Operators | 510 | ||
The Pixel class | 511 | ||
Arithmetic Operators | 512 | ||
friend Functions | 517 | ||
Validating Objects | 522 | ||
Bitwise Operators | 526 | ||
10.3 Problem Solving Applied: Color Image Processing\r | 529 | ||
10.4 Recursion\r | 535 | ||
Factorial Function | 536 | ||
Fibonacci Sequence | 538 | ||
The BinaryTree class | 540 | ||
10.5 Class Templates\r | 552 | ||
10.6 Inheritance\r | 558 | ||
The Rectangle class\r | 558 | ||
The Square class\r | 561 | ||
The Cube class\r | 564 | ||
10.7 virtual Methods\r | 567 | ||
10.8 Problem Solving Applied: Iterated Prisoner's Dilemma\r | 570 | ||
Summary\r | 579 | ||
Appendix A: C++ Standard Library\r | 584 | ||
Appendix B: ASCII Character Codes\r | 592 | ||
Appendix C: Using MATLAB to Plot Data from ASCII Files\r | 596 | ||
C++ Program to Generate a Data File | 596 | ||
ASCII Data File Generated by the C++ Program | 597 | ||
Generating a Plot with MATLAB | 597 | ||
Appendix D: References\r | 599 | ||
Appendix E: Practice! Solutions\r | 600 | ||
Index | 617 |