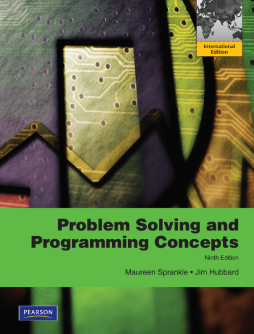
Additional Information
Book Details
Abstract
A core or supplementary text for one-semester, freshman/sophomore-level introductory courses taken by programming majors in Problem Solving for Programmers, Problem Solving for Applications, any Computer Language Course, or Introduction to Programming.
Revised to reflect the most current issues in the programming industry, this widely adopted text emphasizes that problem solving is the same in all computer languages, regardless of syntax. Sprankle and Hubbard use a generic, non-language-specific approach to present the tools and concepts required when using any programming language to develop computer applications. Designed for students with little or no computer experience but useful to programmers at any level the text provides step-by-step progression and consistent in-depth coverage of topics, with detailed explanations and many illustrations.
Instructor Supplements (see resources tab):
Instructor Manual with Solutions and Test Bank
Lecture Power Point Slides
Go to: www.pearsoninternationaleditions.com/sprankle
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Contents | 5 | ||
Preface | 9 | ||
UNIT ONE: INTRODUCTION TO PROBLEM SOLVING AND PROGRAMMING | 13 | ||
Chapter 1 General Problem-Solving Concepts | 15 | ||
Problem Solving in Everyday Life | 15 | ||
Types of Problems | 17 | ||
Problem Solving with Computers | 18 | ||
Difficulties with Problem Solving | 18 | ||
Summary | 19 | ||
New Terms | 19 | ||
Questions | 19 | ||
Problems | 20 | ||
Chapter 2 Beginning Problem-Solving Concepts for the Computer | 23 | ||
Constants and Variables | 25 | ||
Data Types | 28 | ||
How the Computer Stores Data | 32 | ||
Functions | 33 | ||
Operators | 35 | ||
Expressions and Equations | 39 | ||
Summary | 46 | ||
New Terms | 47 | ||
Questions | 47 | ||
Problems | 49 | ||
Chapter 3 Planning Your Solution | 53 | ||
Communicating with the Computer | 54 | ||
Organizing the Solution | 55 | ||
Introduction to UML (Unified Modeling Language) | 67 | ||
Using the Tools | 71 | ||
Testing the Solution | 73 | ||
Coding the Solution | 73 | ||
Software Development Cycle | 74 | ||
Summary | 74 | ||
New Terms | 75 | ||
Questions | 75 | ||
Problems | 75 | ||
UNIT ONE: Supplementary Exercises | 77 | ||
UNIT TWO: LOGIC STRUCTURES | 81 | ||
Chapter 4 An Introduction to Programming Structure | 83 | ||
Pointers for Structuring a Solution | 84 | ||
The Modules and Their Functions | 86 | ||
Cohesion and Coupling | 87 | ||
Local and Global Variables | 89 | ||
Parameters | 91 | ||
Return Values | 96 | ||
Variable Names and the Data Dictionary | 97 | ||
The Three Logic Structures | 97 | ||
Summary | 98 | ||
New Terms | 98 | ||
Questions | 99 | ||
Problems | 99 | ||
Chapter 5 Problem Solving with the Sequential Logic Structure | 101 | ||
Algorithm Instructions, Flowchart Symbols, and Pseudocode | 101 | ||
The Sequential Logic Structure | 104 | ||
Solution Development | 106 | ||
Summary | 113 | ||
Questions | 114 | ||
Problems | 114 | ||
Chapter 6 Problem Solving with Decisions | 117 | ||
The Decision Logic Structure | 118 | ||
Multiple If/Then/Else Instructions | 120 | ||
Using Straight-Through Logic | 122 | ||
Using Positive Logic | 123 | ||
Using Negative Logic | 127 | ||
Logic Conversion | 129 | ||
Which Decision Logic? | 132 | ||
Decision Tables | 132 | ||
Putting It All Together | 139 | ||
The Case Logic Structure | 147 | ||
Codes | 149 | ||
Putting It All Together | 150 | ||
Another Putting It All Together | 152 | ||
Summary | 153 | ||
New Terms | 154 | ||
Questions | 154 | ||
Problems | 155 | ||
Chapter 7 Problem Solving with Loops | 161 | ||
The Loop Logic Structure | 162 | ||
lncrementing | 163 | ||
Accumulating | 163 | ||
While/WhileEnd | 164 | ||
Putting It All Together | 166 | ||
Repeat/Until | 166 | ||
Putting It All Together | 169 | ||
Automatic-Counter Loop | 171 | ||
Putting It All Together | 175 | ||
Nested Loops | 175 | ||
Indicators | 178 | ||
Algorithm Instructions and Flowchart Symbols | 179 | ||
Recursion | 181 | ||
Summary | 181 | ||
New Terms | 186 | ||
Questions | 186 | ||
Problems | 186 | ||
UNIT TWO: Supplementary Exercises | 189 | ||
UNIT THREE: DATA STRUCTURES | 191 | ||
Chapter 8 Processing Arrays | 193 | ||
Arrays | 194 | ||
One-Dimensional Arrays | 196 | ||
Putting It All Together | 201 | ||
Two-Dimensional Arrays | 203 | ||
Putting It All Together | 211 | ||
Multidimensional Arrays | 220 | ||
Table Look-Up Technique | 221 | ||
The Pointer Technique | 225 | ||
Putting It All Together | 238 | ||
Summary | 247 | ||
New Terms | 247 | ||
Questions | 247 | ||
Problems | 248 | ||
Chapter 9 Sorting, Stacks, and Queues | 251 | ||
Sorting Techniques | 252 | ||
Stacks | 259 | ||
Queues | 260 | ||
Summary | 264 | ||
New Terms | 264 | ||
Questions | 264 | ||
Problems | 265 | ||
Chapter 10 File Concepts | 267 | ||
Beginning File Concepts | 268 | ||
Records as a Data Structure | 268 | ||
Primary and Secondary Keys | 268 | ||
Algorithm Instructions and Flowchart Symbols | 268 | ||
Systems Flowcharts | 271 | ||
Designing Records | 271 | ||
Summary | 275 | ||
New Terms | 275 | ||
Questions | 275 | ||
Problems | 275 | ||
Chapter 11 Linked Lists | 277 | ||
Creating Linked Lists | 277 | ||
Examples of Adding Data to/Deleting Data from Linked Lists | 278 | ||
Algorithms and Flowcharts to Add, Delete, and Access Data in a Linked List | 283 | ||
Summary | 296 | ||
New Terms | 296 | ||
Questions | 296 | ||
Problems | 296 | ||
Chapter 12 Binary Trees | 299 | ||
Creation of Binary Trees | 300 | ||
Accessing Data in a Binary Tree | 302 | ||
Traversal of Binary Trees | 306 | ||
Summary | 308 | ||
New Terms | 308 | ||
Questions | 308 | ||
Problems | 308 | ||
UNIT THREE: Supplementary Exercises | 309 | ||
UNIT FOUR: DATABASE MANAGEMENT SYSTEMS | 311 | ||
Chapter 13 Database Management Systems | 313 | ||
Why a DBMS? | 314 | ||
DBMS Components | 315 | ||
DBMS Models | 316 | ||
Client Server Model | 317 | ||
DBMS Tasks | 318 | ||
Summary | 319 | ||
New Terms | 320 | ||
Questions | 320 | ||
Chapter 14 Relational Database Management Systems | 321 | ||
Tables, Records, and Fields | 322 | ||
Normalizing Tables | 323 | ||
Entity Relation Model (ERM) | 327 | ||
Schema | 330 | ||
Creating Tables | 330 | ||
Queries | 332 | ||
Interface Design | 334 | ||
Reports | 335 | ||
Planning a Solution Using an RDBMS | 335 | ||
Summary | 344 | ||
New Terms | 344 | ||
Questions | 345 | ||
Problems | 345 | ||
UNIT FIVE: OBJECT-ORIENTED PROGRAMMING | 347 | ||
Chapter 15 Concepts of Object-Oriented Programming | 349 | ||
Object-Oriented Programming | 350 | ||
Graphical User Interface (GUI) | 360 | ||
Event-Driven Object-Oriented Programming | 360 | ||
Interactivity | 363 | ||
Summary | 363 | ||
New Terms | 364 | ||
Questions | 364 | ||
Problems | 365 | ||
Chapter 16 Object-Oriented Program Design | 367 | ||
Using UML as a Design Tool | 368 | ||
Designing an Object-Oriented Application | 374 | ||
Interface Design | 383 | ||
Summary | 391 | ||
New Terms | 392 | ||
Questions | 392 | ||
Problems | 393 | ||
UNIT SIX: INTRODUCTION TO GAME DEVELOPMENT | 395 | ||
Chapter 17 Introduction to Concepts of Game Development Using Object-Oriented Programming | 397 | ||
Game Development | 398 | ||
Planning the Game | 398 | ||
Steps to Develop a Simple Game | 399 | ||
Summary | 400 | ||
New Terms | 400 | ||
Questions | 400 | ||
Problems | 400 | ||
Chapter 18 Introduction to Assembly Language | 403 | ||
Assembly Language Versus High-Level Languages | 404 | ||
Assembly Language Concepts | 404 | ||
Some Basic Assembly Language Instructions | 404 | ||
Assembly Language Equivalents to the Four Logic Structures | 405 | ||
Summary | 407 | ||
New Terms | 407 | ||
Questions | 407 | ||
Problems | 407 | ||
UNIT SEVEN: FILE PROCESSING | 409 | ||
Chapter 19 Sequential-Access File Applications | 411 | ||
Processing Sequential-Access Files | 413 | ||
The Primer Read | 413 | ||
Designing Output Reports | 415 | ||
Headings and Line Counters | 415 | ||
Control-Breaks | 421 | ||
Multiple Control-Breaks | 425 | ||
Using Indicators for Program Control | 427 | ||
Error Handling | 432 | ||
Null Files | 434 | ||
Summary | 442 | ||
New Terms | 443 | ||
Questions | 443 | ||
Problems | 443 | ||
Chapter 20 Sequential-Access File Updating | 445 | ||
Creating Files | 446 | ||
The Master File | 447 | ||
Transaction Files | 447 | ||
Activity Files | 447 | ||
Backup Files | 447 | ||
Updating the Master File Using a Transaction File | 447 | ||
Putting It All Together | 454 | ||
A Useful Alternative Method | 464 | ||
Summary | 469 | ||
New Terms | 469 | ||
Questions | 469 | ||
Problems | 469 | ||
UNIT SEVEN: Supplementary Exercises | 471 | ||
APPENDIX A: Otto the Robot | 473 | ||
APPENDIX B: ASCII and EBCDIC Codes for Data Representation | 481 | ||
APPENDIX C: Forms to Use in Problem Solving | 485 | ||
APPENDIX D: Other Problem-Solving Tools | 505 | ||
APPENDIX E: Other Functions | 509 | ||
GLOSSARY | 511 | ||
A | 511 | ||
B | 511 | ||
C | 512 | ||
D | 513 | ||
E | 513 | ||
F | 514 | ||
G | 514 | ||
H | 514 | ||
I | 514 | ||
K | 515 | ||
L | 515 | ||
M | 515 | ||
N | 515 | ||
O | 515 | ||
P | 516 | ||
Q | 517 | ||
R | 517 | ||
S | 517 | ||
T | 518 | ||
U | 518 | ||
V | 518 | ||
W | 518 | ||
INDEX | 519 | ||
A | 519 | ||
B | 519 | ||
C | 519 | ||
D | 519 | ||
E | 519 | ||
F | 519 | ||
G | 520 | ||
H | 520 | ||
I | 520 | ||
K | 520 | ||
L | 520 | ||
M | 520 | ||
N | 520 | ||
O | 520 | ||
P | 520 | ||
Q | 521 | ||
R | 521 | ||
S | 521 | ||
T | 521 | ||
U | 521 | ||
V | 521 | ||
W | 521 |