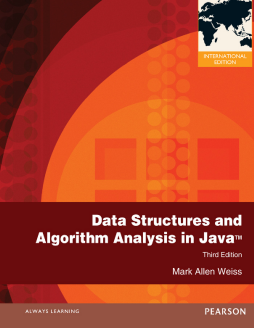
Additional Information
Book Details
Abstract
Data Structures and Algorithm Analysis in Java is an advanced algorithms book that fits between traditional CS2 and Algorithms Analysis courses. In the old ACM Curriculum Guidelines, this course was known as CS7. It is also suitable for a first-year graduate course in algorithm analysis
As the speed and power of computers increases, so does the need for effective programming and algorithm analysis. By approaching these skills in tandem, Mark Allen Weiss teaches readers to develop well-constructed, maximally efficient programs in Java.
Weiss clearly explains topics from binary heaps to sorting to NP-completeness, and dedicates a full chapter to amortized analysis and advanced data structures and their implementation. Figures and examples illustrating successive stages of algorithms contribute to Weiss careful, rigorous and in-depth analysis of each type of algorithm. A logical organization of topics and full access to source code complement the texts coverage.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Contents | 7 | ||
Preface | 17 | ||
Chapter 1 Introduction | 21 | ||
1.1 What's the Book About? | 21 | ||
1.2 Mathematics Review | 22 | ||
1.2.1 Exponents | 23 | ||
1.2.2 Logarithms | 23 | ||
1.2.3 Series | 24 | ||
1.2.4 Modular Arithmetic | 25 | ||
1.2.5 The P Word | 26 | ||
1.3 A Brief Introduction to Recursion | 28 | ||
1.4 Implementing Generic Components Pre-Java 5 | 32 | ||
1.4.1 Using Object for Genericity | 33 | ||
1.4.2 Wrappers for Primitive Types | 34 | ||
1.4.3 Using Interface Types for Genericity | 34 | ||
1.4.4 Compatibility of Array Types | 36 | ||
1.5 Implementing Generic Components Using Java 5 Generics | 36 | ||
1.5.1 Simple Generic Classes and Interfaces | 37 | ||
1.5.2 Autoboxing/Unboxing | 38 | ||
1.5.3 The Diamond Operator | 38 | ||
1.5.4 Wildcards with Bounds | 39 | ||
1.5.5 Generic Static Methods | 40 | ||
1.5.6 Type Bounds | 41 | ||
1.5.7 Type Erasure | 42 | ||
1.5.8 Restrictions on Generics | 43 | ||
1.6 Function Objects | 44 | ||
Summary | 46 | ||
Exercises | 46 | ||
References | 48 | ||
Chapter 2 Algorithm Analysis | 49 | ||
2.1 Mathematical Background | 49 | ||
2.2 Model | 52 | ||
2.3 What to Analyze | 53 | ||
2.4 Running Time Calculations | 55 | ||
2.4.1 A Simple Example | 56 | ||
2.4.2 General Rules | 56 | ||
2.4.3 Solutions for the Maximum Subsequence Sum Problem | 59 | ||
2.4.4 Logarithms in the Running Time | 65 | ||
2.4.5 A Grain of Salt | 69 | ||
Summary | 69 | ||
Exercises | 70 | ||
References | 75 | ||
Chapter 3 Lists, Stacks, and Queues | 77 | ||
3.1 Abstract Data Types (ADTs) | 77 | ||
3.2 The List ADT | 78 | ||
3.2.1 Simple Array Implementation of Lists | 78 | ||
3.2.2 Simple Linked Lists | 79 | ||
3.3 Lists in the Java Collections API | 81 | ||
3.3.1 Collection Interface | 81 | ||
3.3.2 Iterators | 81 | ||
3.3.3 The List Interface, ArrayList, and LinkedList | 83 | ||
3.3.4 Example: Using remove on a LinkedList | 85 | ||
3.3.5 ListIterators | 87 | ||
3.4 Implementation of ArrayList | 87 | ||
3.4.1 The Basic Class | 88 | ||
3.4.2 The Iterator and Java Nested and Inner Classes | 91 | ||
3.5 Implementation of LinkedList | 95 | ||
3.6 The Stack ADT | 102 | ||
3.6.1 Stack Model | 102 | ||
3.6.2 Implementation of Stacks | 103 | ||
3.6.3 Applications | 104 | ||
3.7 The Queue ADT | 112 | ||
3.7.1 Queue Model | 112 | ||
3.7.2 Array Implementation of Queues | 112 | ||
3.7.3 Applications of Queues | 115 | ||
Summary | 116 | ||
Exercises | 116 | ||
Chapter 4 Trees | 121 | ||
4.1 Preliminaries | 121 | ||
4.1.1 Implementation of Trees | 122 | ||
4.1.2 Tree Traversals with an Application | 123 | ||
4.2 Binary Trees | 127 | ||
4.2.1 Implementation | 128 | ||
4.2.2 An Example: Expression Trees | 129 | ||
4.3 The Search Tree ADT—Binary Search Trees | 132 | ||
4.3.1 contains | 133 | ||
4.3.2 findMin and findMax | 135 | ||
4.3.3 insert | 136 | ||
4.3.4 remove | 138 | ||
4.3.5 Average-Case Analysis | 140 | ||
4.4 AVL Trees | 143 | ||
4.4.1 Single Rotation | 145 | ||
4.4.2 Double Rotation | 148 | ||
4.5 Splay Trees | 157 | ||
4.5.1 A Simple Idea (That Does Not Work) | 157 | ||
4.5.2 Splaying | 159 | ||
4.6 Tree Traversals (Revisited) | 165 | ||
4.7 B-Trees | 167 | ||
4.8 Sets and Maps in the Standard Library | 172 | ||
4.8.1 Sets | 172 | ||
4.8.2 Maps | 173 | ||
4.8.3 Implementation of TreeSet and TreeMap | 173 | ||
4.8.4 An Example That Uses Several Maps | 174 | ||
Summary | 180 | ||
Exercises | 180 | ||
References | 187 | ||
Chapter 5 Hashing | 191 | ||
5.1 General Idea | 191 | ||
5.2 Hash Function | 192 | ||
5.3 Separate Chaining | 194 | ||
5.4 Hash Tables Without Linked Lists | 199 | ||
5.4.1 Linear Probing | 199 | ||
5.4.2 Quadratic Probing | 201 | ||
5.4.3 Double Hashing | 203 | ||
5.5 Rehashing | 208 | ||
5.6 Hash Tables in the Standard Library | 209 | ||
5.7 Hash Tables with Worst-Case O(1) Access | 212 | ||
5.7.1 Perfect Hashing | 213 | ||
5.7.2 Cuckoo Hashing | 215 | ||
5.7.3 Hopscotch Hashing | 225 | ||
5.8 Universal Hashing | 231 | ||
5.9 Extendible Hashing | 234 | ||
Summary | 237 | ||
Exercises | 238 | ||
References | 242 | ||
Chapter 6 Priority Queues (Heaps) | 245 | ||
6.1 Model | 245 | ||
6.2 Simple Implementations | 246 | ||
6.3 Binary Heap | 246 | ||
6.3.1 Structure Property | 247 | ||
6.3.2 Heap-Order Property | 249 | ||
6.3.3 Basic Heap Operations | 249 | ||
6.3.4 Other Heap Operations | 254 | ||
6.4 Applications of Priority Queues | 258 | ||
6.4.1 The Selection Problem | 258 | ||
6.4.2 Event Simulation | 259 | ||
6.5 d-Heaps | 260 | ||
6.6 Leftist Heaps | 261 | ||
6.6.1 Leftist Heap Property | 261 | ||
6.6.2 Leftist Heap Operations | 262 | ||
6.7 Skew Heaps | 269 | ||
6.8 Binomial Queues | 272 | ||
6.8.1 Binomial Queue Structure | 272 | ||
6.8.2 Binomial Queue Operations | 273 | ||
6.8.3 Implementation of Binomial Queues | 276 | ||
6.9 Priority Queues in the Standard Library | 281 | ||
Summary | 281 | ||
Exercises | 283 | ||
References | 287 | ||
Chapter 7 Sorting | 291 | ||
7.1 Preliminaries | 291 | ||
7.2 Insertion Sort | 292 | ||
7.2.1 The Algorithm | 292 | ||
7.2.2 Analysis of Insertion Sort | 292 | ||
7.3 A Lower Bound for Simple Sorting Algorithms | 293 | ||
7.4 Shellsort | 294 | ||
7.4.1 Worst-Case Analysis of Shellsort | 296 | ||
7.5 Heapsort | 298 | ||
7.5.1 Analysis of Heapsort | 299 | ||
7.6 Mergesort | 302 | ||
7.6.1 Analysis of Mergesort | 304 | ||
7.7 Quicksort | 308 | ||
7.7.1 Picking the Pivot | 310 | ||
7.7.2 Partitioning Strategy | 312 | ||
7.7.3 Small Arrays | 314 | ||
7.7.4 Actual Quicksort Routines | 314 | ||
7.7.5 Analysis of Quicksort | 317 | ||
7.7.6 A Linear-Expected-Time Algorithm for Selection | 320 | ||
7.8 A General Lower Bound for Sorting | 322 | ||
7.8.1 Decision Trees | 322 | ||
7.9 Decision-Tree Lower Bounds for Selection Problems | 324 | ||
7.10 Adversary Lower Bounds | 327 | ||
7.11 Linear-Time Sorts: Bucket Sort and Radix Sort | 330 | ||
7.12 External Sorting | 335 | ||
7.12.1 Why We Need New Algorithms | 336 | ||
7.12.2 Model for External Sorting | 336 | ||
7.12.3 The Simple Algorithm | 336 | ||
7.12.4 Multiway Merge | 337 | ||
7.12.5 Polyphase Merge | 338 | ||
7.12.6 Replacement Selection | 339 | ||
Summary | 341 | ||
Exercises | 341 | ||
References | 347 | ||
Chapter 8 The Disjoint Set Class | 351 | ||
8.1 Equivalence Relations | 351 | ||
8.2 The Dynamic Equivalence Problem | 352 | ||
8.3 Basic Data Structure | 353 | ||
8.4 Smart Union Algorithms | 357 | ||
8.5 Path Compression | 360 | ||
8.6 Worst Case for Union-by-Rank and Path Compression | 361 | ||
8.6.1 Slowly Growing Functions | 362 | ||
8.6.2 An Analysis By Recursive Decomposition | 363 | ||
8.6.3 An O( M log * N ) Bound | 370 | ||
8.6.4 An O( M α(M, N) ) Bound | 370 | ||
8.7 An Application | 372 | ||
Summary | 375 | ||
Exercises | 375 | ||
References | 377 | ||
Chapter 9 Graph Algorithms | 379 | ||
9.1 Definitions | 379 | ||
9.1.1 Representation of Graphs | 380 | ||
9.2 Topological Sort | 382 | ||
9.3 Shortest-Path Algorithms | 386 | ||
9.3.1 Unweighted Shortest Paths | 387 | ||
9.3.2 Dijkstra's Algorithm | 392 | ||
9.3.3 Graphs with Negative Edge Costs | 400 | ||
9.3.4 Acyclic Graphs | 400 | ||
9.3.5 All-Pairs Shortest Path | 404 | ||
9.3.6 Shortest-Path Example | 404 | ||
9.4 Network Flow Problems | 406 | ||
9.4.1 A Simple Maximum-Flow Algorithm | 408 | ||
9.5 Minimum Spanning Tree | 413 | ||
9.5.1 Prim's Algorithm | 414 | ||
9.5.2 Kruskal's Algorithm | 417 | ||
9.6 Applications of Depth-First Search | 419 | ||
9.6.1 Undirected Graphs | 420 | ||
9.6.2 Biconnectivity | 422 | ||
9.6.3 Euler Circuits | 425 | ||
9.6.4 Directed Graphs | 429 | ||
9.6.5 Finding Strong Components | 431 | ||
9.7 Introduction to NP-Completeness | 432 | ||
9.7.1 Easy vs. Hard | 433 | ||
9.7.2 The Class NP | 434 | ||
9.7.3 NP-Complete Problems | 435 | ||
Summary | 437 | ||
Exercises | 437 | ||
References | 445 | ||
Chapter 10 Algorithm Design Techniques | 449 | ||
10.1 Greedy Algorithms | 449 | ||
10.1.1 A Simple Scheduling Problem | 450 | ||
10.1.2 Huffman Codes | 453 | ||
10.1.3 Approximate Bin Packing | 459 | ||
10.2 Divide and Conquer | 468 | ||
10.2.1 Running Time of Divide-and-Conquer Algorithms | 469 | ||
10.2.2 Closest-Points Problem | 471 | ||
10.2.3 The Selection Problem | 475 | ||
10.2.4 Theoretical Improvements for Arithmetic Problems | 478 | ||
10.3 Dynamic Programming | 482 | ||
10.3.1 Using a Table Instead of Recursion | 483 | ||
10.3.2 Ordering Matrix Multiplications | 486 | ||
10.3.3 Optimal Binary Search Tree | 489 | ||
10.3.4 All-Pairs Shortest Path | 492 | ||
10.4 Randomized Algorithms | 494 | ||
10.4.1 Random Number Generators | 496 | ||
10.4.2 Skip Lists | 500 | ||
10.4.3 Primality Testing | 503 | ||
10.5 Backtracking Algorithms | 506 | ||
10.5.1 The Turnpike Reconstruction Problem | 507 | ||
10.5.2 Games | 510 | ||
Summary | 519 | ||
Exercises | 519 | ||
References | 528 | ||
Chapter 11 Amortized Analysis | 533 | ||
11.1 An Unrelated Puzzle | 534 | ||
11.2 Binomial Queues | 534 | ||
11.3 Skew Heaps | 539 | ||
11.4 Fibonacci Heaps | 542 | ||
11.4.1 Cutting Nodes in Leftist Heaps | 542 | ||
11.4.2 Lazy Merging for Binomial Queues | 545 | ||
11.4.3 The Fibonacci Heap Operations | 548 | ||
11.4.4 Proof of the Time Bound | 549 | ||
11.5 Splay Trees | 551 | ||
Summary | 556 | ||
Exercises | 556 | ||
References | 558 | ||
Chapter 12 Advanced Data Structures and Implementation | 561 | ||
12.1 Top-Down Splay Trees | 561 | ||
12.2 Red-Black Trees | 569 | ||
12.2.1 Bottom-Up Insertion | 569 | ||
12.2.2 Top-Down Red-Black Trees | 571 | ||
12.2.3 Top-Down Deletion | 576 | ||
12.3 Treaps | 578 | ||
12.4 Suffix Arrays and Suffix Trees | 580 | ||
12.4.1 Suffix Arrays | 581 | ||
12.4.2 Suffix Trees | 584 | ||
12.4.3 Linear-Time Construction of Suffix Arrays and Suffix Trees | 587 | ||
12.5 k-d Trees | 598 | ||
12.6 Pairing Heaps | 603 | ||
Summary | 608 | ||
Exercises | 610 | ||
References | 614 | ||
Index | 617 | ||
A | 617 | ||
B | 618 | ||
C | 619 | ||
D | 620 | ||
E | 621 | ||
F | 621 | ||
G | 622 | ||
H | 623 | ||
I | 623 | ||
J | 624 | ||
K | 624 | ||
L | 624 | ||
M | 625 | ||
N | 626 | ||
O | 626 | ||
P | 627 | ||
Q | 628 | ||
R | 628 | ||
S | 629 | ||
T | 630 | ||
U | 631 | ||
V | 631 | ||
W | 632 | ||
X | 632 | ||
Y | 632 | ||
Z | 632 |