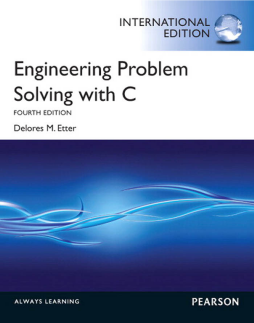
Additional Information
Book Details
Abstract
This introductory-level C programming book is designed primarily for engineering students required to learn how to program.
In Engineering Problem Solving with C, 4e, best-selling author, Delores Etter, uses real-world engineering and scientific examples and problems throughout the text. Solutions to the problems are developed using the language C and the author's signature five-step problem solving process. Since learning any new skill requires practice at a number of different levels of difficulty, four types of exercises are presented to develop problem-solving skills - Practice! problems, Modify! problems, Short-Answer problems, and Programming problems. The author's clear and precise style creates a highly accessible and readable text for students of all levels.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Contents | 13 | ||
1 Engineering Problem Solving | 23 | ||
Crime Scene Investigation | 22 | ||
1.1 Engineering in the 21[sup(st)] Century | 23 | ||
Recent Engineering Achievements | 23 | ||
Changing Engineering Environment | 29 | ||
1.2 Computing Systems: Hardware and Software | 30 | ||
Computer Hardware | 30 | ||
Computer Software | 31 | ||
Operating Systems | 32 | ||
Software Tools | 32 | ||
Computer Languages | 33 | ||
Executing a Computer Program | 34 | ||
Software Life Cycle | 35 | ||
1.3 An Engineering Problem-Solving Methodology | 36 | ||
Summary | 39 | ||
Key Terms | 39 | ||
Problems | 40 | ||
2 Simple C Programs | 45 | ||
Crime Scene Investigation: Forensic Anthropology | 44 | ||
2.1 Program Structure | 45 | ||
2.2 Constants and Variables | 49 | ||
Scientific Notation | 50 | ||
Numeric Data Types | 51 | ||
Character Data | 53 | ||
Symbolic Constants | 54 | ||
2.3 Assignment Statements | 55 | ||
Arithmetic Operators | 56 | ||
Priority of Operators | 58 | ||
Overflow and Underflow | 61 | ||
Increment and Decrement Operators | 61 | ||
Abbreviated Assignment Operators | 62 | ||
2.4 Standard Input and Output | 63 | ||
Printf Function | 64 | ||
Scanf Function | 67 | ||
2.5 Problem Solving Applied: Estimating Height from Bone Lengths | 68 | ||
2.6 Numerical Technique: Linear Interpolation | 72 | ||
2.7 Problem Solving Applied: Freezing Temperature of Seawater | 76 | ||
2.8 Mathematical Functions | 80 | ||
Elementary Math Functions | 81 | ||
Trigonometric Functions | 82 | ||
Hyperbolic Functions | 84 | ||
2.9 Character Functions | 85 | ||
Character I/O | 85 | ||
Character Comparisons | 86 | ||
2.10 Problem Solving Applied: Velocity Computation | 87 | ||
2.11 System Limitations | 91 | ||
Summary | 92 | ||
Key Terms | 92 | ||
C Statement Summary | 93 | ||
Style: Notes | 93 | ||
Debugging Notes | 94 | ||
Problems | 94 | ||
3 Control Structures and Data Files | 101 | ||
Crime Scene Investigation: Face Recognition and Surveillance Video | 100 | ||
3.1 Algorithm Development | 101 | ||
Top-Down Design | 101 | ||
Decomposition Outline | 102 | ||
Refinement with Pseudocode and Flowcharts | 102 | ||
Structured Programming | 102 | ||
Sequence | 102 | ||
Selection | 103 | ||
Repetition | 104 | ||
Evaluation of Alternative Solutions | 105 | ||
Error Conditions | 106 | ||
Generation of Test Data | 107 | ||
3.2 Conditional Expressions | 108 | ||
Relational Operators | 108 | ||
Logical Operators | 109 | ||
Precedence and Associativity | 109 | ||
3.3 Selection Statements | 110 | ||
Simple if Statement | 110 | ||
If/else Statement | 112 | ||
Switch Statement | 115 | ||
3.4 Problem Solving Applied: Face Recognition | 117 | ||
3.5 Loop Structures | 121 | ||
While Loop | 122 | ||
Do/while Loop | 123 | ||
For Loop | 124 | ||
Break and continue Statements | 127 | ||
3.6 Problem Solving Applied: Wave Interaction | 128 | ||
3.7 Data Files | 136 | ||
I/O Statements | 137 | ||
Reading Data Files | 139 | ||
Specified Number of Records | 139 | ||
Trailer or Sentinel Signals | 142 | ||
End-of-File | 144 | ||
Generating a Data File | 146 | ||
3.8 Numerical Technique: Linear Modeling* | 148 | ||
3.9 Problem Solving Applied: Ozone Measurements* | 151 | ||
Summary | 157 | ||
Key Terms | 157 | ||
C Statement Summary | 157 | ||
Style: Notes | 159 | ||
Debugging Notes | 159 | ||
Problems | 160 | ||
4 Modular Programming with Functions | 169 | ||
Crime Scene Investigation: Iris Recognition | 168 | ||
4.1 Modularity | 169 | ||
4.2 Programmer-Defined Functions | 172 | ||
Function Example | 172 | ||
Function Definition | 176 | ||
Function Prototype | 177 | ||
Parameter List | 178 | ||
Storage Class and Scope | 180 | ||
4.3 Problem Solving Applied: Computing the Boundaries of the Iris | 183 | ||
4.4 Problem Solving Applied: Iceberg Tracking | 189 | ||
4.5 Random Numbers | 195 | ||
Integer Sequences | 195 | ||
Floating-Point Sequences | 199 | ||
4.6 Problem Solving Applied: Instrumentation Reliability | 200 | ||
4.7 Numerical Technique: Roots of Polynomials* | 206 | ||
Polynomial Roots | 206 | ||
Incremental-Search Technique | 208 | ||
4.8 Problem Solving Applied: System Stability* | 210 | ||
4.9 Macros* | 216 | ||
4.10 Recursion* | 219 | ||
Factorial Computation | 220 | ||
Fibonacci Sequence | 222 | ||
Summary | 224 | ||
Key Terms | 224 | ||
C Statement Summary | 224 | ||
Style: Notes | 225 | ||
Debugging Notes | 225 | ||
Problems | 225 | ||
5 Arrays and Matrices | 233 | ||
Crime Scene Investigation: Speech Analysis and Speech Recognition | 232 | ||
5.1 One-Dimensional Arrays | 233 | ||
Definition and Initialization | 234 | ||
Computations and Output | 236 | ||
Function Arguments | 238 | ||
5.2 Problem Solving Applied: Hurricane Categories | 241 | ||
5.3 Problem Solving Applied: Molecular Weights | 246 | ||
5.4 Statistical Measurements | 251 | ||
Simple Analysis | 251 | ||
Maximum and Minimum | 252 | ||
Average | 252 | ||
Median | 252 | ||
Variance and Standard Deviation | 253 | ||
Custom Header File | 255 | ||
5.5 Problem Solving Applied: Speech Signal Analysis | 256 | ||
5.6 Sorting Algorithms | 262 | ||
5.7 Search Algorithms | 264 | ||
Unordered List | 264 | ||
Ordered List | 265 | ||
5.8 Two-Dimensional Arrays | 268 | ||
Definition and Initialization | 269 | ||
Computations and Output | 271 | ||
Function Arguments | 273 | ||
5.9 Problem Solving Applied: Terrain Navigation | 276 | ||
5.10 Matrices and Vectors* | 280 | ||
Dot Product | 280 | ||
Determinant | 281 | ||
Transpose | 282 | ||
Matrix Addition and Subtraction | 283 | ||
Matrix Multiplication | 283 | ||
5.11 Numerical Technique: Solution to Simultaneous Equations* | 285 | ||
Graphical Interpretation | 285 | ||
Gauss Elimination | 290 | ||
5.12 Problem Solving Applied: Electrical Circuit Analysis* | 292 | ||
5.13 Higher Dimensional Arrays* | 297 | ||
Summary | 299 | ||
Key Terms | 299 | ||
C Statement Summary | 300 | ||
Style: Notes | 300 | ||
Debugging Notes | 300 | ||
Problems | 301 | ||
6 Programming with Pointers | 309 | ||
Crime Scene Investigation:DNA Analysis | 308 | ||
6.1 Addresses and Pointers | 309 | ||
Address Operator | 310 | ||
Pointer Assignment | 312 | ||
Address Arithmetic | 315 | ||
6.2 Pointers to Array Elements | 317 | ||
One-Dimensional Arrays | 318 | ||
Two-Dimensional Arrays | 320 | ||
6.3 Problem Solving Applied: E1 NiƱo-Southern Oscillation Data | 323 | ||
6.4 Pointers in Function References | 326 | ||
6.5 Problem Solving Applied: Seismic Event Detection | 329 | ||
6.6 Character Strings | 334 | ||
String Definition and I/O | 334 | ||
String Functions | 335 | ||
6.7 Problem Solving Applied: DNA Sequencing | 338 | ||
6.8 Dynamic Memory Allocation* | 341 | ||
6.9 A Quicksort Algorithm* | 345 | ||
Summary | 348 | ||
Key Terms | 349 | ||
C Statement Summary | 349 | ||
Style: Notes | 349 | ||
Debugging Notes | 349 | ||
Problems | 349 | ||
7 Programming with Structures | 355 | ||
Crime Scene Investigation: Fingerprint Recognition | 354 | ||
7.1 Structures | 355 | ||
Definition and Initialization | 356 | ||
Input and Output | 357 | ||
Computations | 359 | ||
7.2 Using Functions with Structures | 360 | ||
Structures as Function Arguments | 360 | ||
Functions that Return Structures | 361 | ||
7.3 Problem Solving Applied: Fingerprint Analysis | 362 | ||
7.4 Arrays of Structures | 366 | ||
7.5 Problem Solving Applied: Tsunami Analysis | 369 | ||
7.6 Dynamic Data Structures* | 373 | ||
Additional Dynamic Data Structures | 381 | ||
Circularly Linked List | 381 | ||
Doubly Linked List | 382 | ||
Stack | 383 | ||
Queue | 383 | ||
Binary Tree | 384 | ||
Summary | 386 | ||
Key Terms | 386 | ||
C Statement Summary | 386 | ||
Style: Notes | 387 | ||
Debugging Notes | 387 | ||
Problems | 387 | ||
8 An Introduction to C | 393 | ||
Crime Scene Investigation: Hand Recognition | 392 | ||
8.1 Object-Oriented Programming | 393 | ||
8.2 C++ Program Structure | 394 | ||
8.3 Input and Output | 395 | ||
The cout Object | 395 | ||
Stream Functions | 396 | ||
The cin Object | 397 | ||
Defining File Streams | 398 | ||
8.4 C++ Program Examples | 399 | ||
Simple Computations | 399 | ||
Loops | 400 | ||
Functions, One-Dimensional Arrays, and Data Files | 400 | ||
8.5 Problem Solving Applied: Hand Recognition | 402 | ||
8.6 Problem Solving Applied: Surface Wind Directions | 405 | ||
8.7 Classes | 409 | ||
Defining a Class Data Type | 409 | ||
Constructor Functions | 412 | ||
Class Operators | 414 | ||
8.8 Numerical Technique: Complex Roots | 415 | ||
Complex Class Definition | 416 | ||
Complex Roots for Quadratic Equations | 419 | ||
Summary | 422 | ||
Key Terms | 422 | ||
C++ Statement Summary | 422 | ||
Style: Notes | 423 | ||
Debugging Notes | 423 | ||
Problems | 423 | ||
Appendices | 427 | ||
A: ANSI C Standard Library | 427 | ||
427 | |||
427 | |||
428 | |||
428 | |||
429 | |||
430 | |||
430 | |||
431 | |||
431 | |||
431 | |||
431 | |||
431 | |||
434 | |||
435 | |||
436 | |||
B: ASCII Character Codes | 438 | ||
C: Using MATLAB to Plot Data from Text Files | 441 | ||
Complete Solutions to Practice! Problems | 444 | ||
Selected Solutions to Modify! Problems | 456 | ||
Complete Solutions to End-of-Chapter Short-Answer Problems | 458 | ||
Selected Solutions to End-of-Chapter Programming Problems | 462 | ||
Glossary | 466 | ||
A | 466 | ||
B | 466 | ||
C | 466 | ||
D | 467 | ||
E | 468 | ||
F | 468 | ||
G | 469 | ||
H | 469 | ||
I | 469 | ||
K | 469 | ||
L | 469 | ||
M | 469 | ||
N | 470 | ||
O | 470 | ||
P | 470 | ||
Q | 471 | ||
R | 471 | ||
S | 471 | ||
T | 472 | ||
U | 473 | ||
V | 473 | ||
W | 473 | ||
Z | 473 | ||
Index | 474 | ||
A | 474 | ||
B | 475 | ||
C | 475 | ||
D | 475 | ||
E | 476 | ||
F | 476 | ||
G | 477 | ||
H | 477 | ||
I | 477 | ||
J | 477 | ||
K | 477 | ||
L | 477 | ||
M | 478 | ||
N | 478 | ||
O | 478 | ||
P | 478 | ||
Q | 479 | ||
R | 479 | ||
S | 479 | ||
T | 480 | ||
U | 480 | ||
V | 480 | ||
W | 480 | ||
Z | 480 |