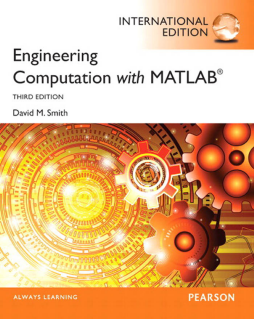
Additional Information
Book Details
Abstract
This textbook is ideal for MATLAB/Introduction to Programming courses in both Engineering and Computer Science departments.
Engineering Computation with MATLAB introduces the power of computing to engineering students who have no programming experience. The book places the fundamental tenets of computer programming into the context of MATLAB, employing hands-on exercises, examples from the engineering industry, and a variety of core tools to increase programming proficiency and capability. With this knowledge, students are prepared to adapt learned concepts to other programming languages.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Contents | 5 | ||
Chapter 1 Introduction to Computers and Programming | 19 | ||
1.1 Background | 20 | ||
1.2 History of Computer Architectures | 21 | ||
1.2.1 Babbage’s Difference Engine | 21 | ||
1.2.2 Colossus | 21 | ||
1.2.3 The von Neumann Architecture | 22 | ||
1.3 Computing Systems Today | 23 | ||
1.3.1 Computer Hardware | 23 | ||
1.3.2 Computer Memory | 24 | ||
1.3.3 Computer Software | 26 | ||
1.3.4 Running a Computer Program | 29 | ||
1.4 Running an Interpreted Program | 31 | ||
1.5 Anticipated Outcomes | 31 | ||
1.5.1 Introduction to MATLAB | 31 | ||
1.5.2 Learning Programming Concepts | 32 | ||
1.5.3 Problem-Solving Skills | 32 | ||
Chapter 2 Getting Started | 35 | ||
2.1 Programming Language Background | 36 | ||
2.1.1 Abstraction | 36 | ||
2.1.2 Algorithms | 37 | ||
2.1.3 Programming Paradigms | 38 | ||
2.2 Basic Data Manipulation | 38 | ||
2.2.1 Starting and Stopping MATLAB | 38 | ||
2.2.2 Assigning Values to Variables | 39 | ||
2.2.3 Data Typing | 40 | ||
2.2.4 Classes and Objects | 42 | ||
2.3 MATLAB User Interface | 42 | ||
2.3.1 Command Window | 43 | ||
2.3.2 Command History | 44 | ||
2.3.3 Workspace Window | 45 | ||
2.3.4 Current Directory Window | 48 | ||
2.3.5 Variable Editor | 49 | ||
2.3.6 Figure Window | 49 | ||
2.3.7 Editor Window | 50 | ||
2.4 Scripts | 51 | ||
2.4.1 Text Files | 51 | ||
2.4.2 Creating Scripts | 51 | ||
2.4.3 The Current Directory | 52 | ||
2.4.4 Running Scripts | 53 | ||
2.4.5 Punctuating Scripts | 53 | ||
2.4.6 Debugging Scripts | 54 | ||
2.5 Engineering Example—Spacecraft Launch | 54 | ||
Chapter 3 Vectors and Arrays | 63 | ||
3.1 Concept: Using Built-in Functions | 64 | ||
3.2 Concept: Data Collections | 64 | ||
3.2.1 Data Abstraction | 64 | ||
3.2.2 Homogeneous Collection | 64 | ||
3.3 Vectors | 64 | ||
3.3.1 Creating a Vector | 65 | ||
3.3.2 Size of a Vector | 66 | ||
3.3.3 Indexing a Vector | 66 | ||
3.3.4 Shortening a Vector | 68 | ||
3.3.5 Operating on Vectors | 69 | ||
3.4 Engineering Example—Forces and Moments | 76 | ||
3.5 Arrays | 78 | ||
3.5.1 Properties of an Array | 79 | ||
3.5.2 Creating an Array | 80 | ||
3.5.3 Accessing Elements of an Array | 80 | ||
3.5.4 Removing Elements of an Array | 81 | ||
3.5.5 Operating on Arrays | 82 | ||
3.6 Engineering Example—Computing Soil Volume | 89 | ||
Chapter 4 Execution Control | 99 | ||
4.1 Concept: Code Blocks | 100 | ||
4.2 Conditional Execution in General | 100 | ||
4.3 if Statements | 101 | ||
4.3.1 General Template | 102 | ||
4.3.2 MATLAB Implementation | 102 | ||
4.3.3 Important Ideas | 104 | ||
4.4 switch Statements | 106 | ||
4.4.1 General Template | 106 | ||
4.4.2 MATLAB Implementation | 107 | ||
4.5 Iteration in General | 108 | ||
4.6 for Loops | 108 | ||
4.6.1 General for Loop Template | 109 | ||
4.6.2 MATLAB Implementation | 109 | ||
4.6.3 Indexing Implementation | 111 | ||
4.6.4 Breaking out of a for Loop | 112 | ||
4.7 while Loops | 112 | ||
4.7.1 General while Template | 112 | ||
4.7.2 MATLAB while Loop Implementation | 113 | ||
4.7.3 Loop-and-a-Half Implementation | 114 | ||
4.7.4 Breaking a while Loop | 115 | ||
4.8 Engineering Example—Computing Liquid Levels | 115 | ||
Chapter 5 Functions | 123 | ||
5.1 Concepts: Abstraction and Encapsulation | 124 | ||
5.2 Black Box View of a Function | 124 | ||
5.3 MATLAB Implementation | 125 | ||
5.3.1 General Template | 125 | ||
5.3.2 Function Definition | 125 | ||
5.3.3 Storing and Using Functions | 127 | ||
5.3.4 Calling Functions | 127 | ||
5.3.5 Variable Numbers of Parameters | 127 | ||
5.3.6 Returning Multiple Results | 128 | ||
5.3.7 Auxiliary Local Functions | 129 | ||
5.3.8 Encapsulation in MATLAB Functions | 129 | ||
5.3.9 Global Variables | 130 | ||
5.4 Engineering Example—Measuring a Solid Object | 131 | ||
Chapter 6 Character Strings | 139 | ||
6.1 Character String Concepts: Mapping Casting, Tokens, and Delimiting | 140 | ||
6.2 MATLAB Implementation | 141 | ||
6.2.1 Slicing and Concatenating Strings | 142 | ||
6.2.2 Arithmetic and Logical Operations | 143 | ||
6.2.3 Useful Functions | 143 | ||
6.3 Format Conversion Functions | 143 | ||
6.3.1 Conversion from Numbers to Strings | 143 | ||
6.3.2 Conversion from Strings to Numbers | 145 | ||
6.4 Character String Operations | 147 | ||
6.4.1 Simple Data Output: The disp(...) Function | 147 | ||
6.4.2 Complex Output | 147 | ||
6.4.3 Comparing Strings | 147 | ||
6.5 Arrays of Strings | 149 | ||
6.6 Engineering Example—Encryption | 150 | ||
Chapter 7 Cell Arrays and Structures | 159 | ||
7.1 Concept: Collecting Dissimilar Objects | 160 | ||
7.2 Cell Arrays | 160 | ||
7.2.1 Creating Cell Arrays | 160 | ||
7.2.2 Accessing Cell Arrays | 161 | ||
7.2.3 Using Cell Arrays | 163 | ||
7.2.4 Processing Cell Arrays | 163 | ||
7.3 Structures | 164 | ||
7.3.1 Constructing and Accessing One Structure | 165 | ||
7.3.2 Constructor Functions | 166 | ||
7.4 Structure Arrays | 168 | ||
7.4.1 Constructing Structure Arrays | 168 | ||
7.4.2 Accessing Structure Elements | 170 | ||
7.4.3 Manipulating Structures | 172 | ||
7.5 Engineering Example—Assembling a Physical Structure | 174 | ||
Chapter 8 File Input and Output | 185 | ||
8.1 Concept: Serial Input and Output (I/O) | 186 | ||
8.2 Workspace I/O | 186 | ||
8.3 High-Level I/O Functions | 187 | ||
8.3.1 Exploration | 187 | ||
8.3.2 Spreadsheets | 188 | ||
8.3.3 Delimited Text Files | 190 | ||
8.4 Lower-Level File I/O | 192 | ||
8.4.1 Opening and Closing Files | 192 | ||
8.4.2 Reading Text Files | 192 | ||
8.4.3 Examples of Reading Text Files | 193 | ||
8.4.4 Writing Text Files | 194 | ||
8.5 Engineering Example—Spreadsheet Data | 195 | ||
Chapter 9 Recursion | 203 | ||
9.1 Concept: The Activation Stack | 204 | ||
9.1.1 A Stack | 204 | ||
9.1.2 Activation Stack | 204 | ||
9.1.3 Function Instances | 205 | ||
9.2 Recursion Defined | 205 | ||
9.3 Implementing a Recursive Function | 206 | ||
9.4 Exceptions | 208 | ||
9.4.1 Historical Approaches | 209 | ||
9.4.2 Generic Exception Implementation | 209 | ||
9.4.3 MATLAB Implementation | 211 | ||
9.5 Wrapper Functions | 213 | ||
9.6 Examples of Recursion | 215 | ||
9.6.1 Detecting Palindromes | 215 | ||
9.6.2 Fibonacci Series | 216 | ||
9.6.3 Zeros of a Function | 217 | ||
9.7 Engineering Example—Robot Arm Motion | 220 | ||
Chapter 10 Principles of Problem Solving | 229 | ||
10.1 Solving Simple Problems | 230 | ||
10.2 Assembling Solution Steps | 230 | ||
10.3 Summary of Operations | 230 | ||
10.3.1 Basic Arithmetic Operations | 231 | ||
10.3.2 Inserting into a Collection | 231 | ||
10.3.3 Traversing a Collection | 233 | ||
10.3.4 Building a Collection | 234 | ||
10.3.5 Mapping a Collection | 234 | ||
10.3.6 Filtering a Collection | 235 | ||
10.3.7 Summarizing a Collection | 236 | ||
10.3.8 Searching a Collection | 237 | ||
10.3.9 Sorting a Collection | 238 | ||
10.4 Solving Larger Problems | 238 | ||
10.5 Engineering Example—Processing Geopolitical Data | 239 | ||
Chapter 11 Plotting | 249 | ||
11.1 Plotting in General | 250 | ||
11.1.1 A Figure—The Plot Container | 250 | ||
11.1.2 Simple Functions for Enhancing Plots | 250 | ||
11.1.3 Multiple Plots on One Figure—Subplots | 251 | ||
11.1.4 Manually Editing Plots | 252 | ||
11.2 2-D Plotting | 253 | ||
11.2.1 Simple Plots | 253 | ||
11.2.2 Plot Options | 255 | ||
11.2.3 Parametric Plots | 255 | ||
11.2.4 Other 2-D Plot Capabilities | 257 | ||
11.3 3-D Plotting | 257 | ||
11.3.1 Linear 3-D Plots | 257 | ||
11.3.2 Linear Parametric 3-D Plots | 259 | ||
11.3.3 Other 3-D Plot Capabilities | 260 | ||
11.4 Surface Plots | 261 | ||
11.4.1 Basic Capabilities | 261 | ||
11.4.2 Simple Exercises | 261 | ||
11.4.3 3-D Parametric Surfaces | 266 | ||
11.4.4 Bodies of Rotation | 268 | ||
11.4.5 Other 3-D Surface Plot Capabilities | 273 | ||
11.4.6 Assembling Compound Surfaces | 274 | ||
11.5 Manipulating Plotted Data | 274 | ||
11.6 Engineering Example—Visualizing Geographic Data | 274 | ||
11.6.1 Analyzing the Data | 275 | ||
11.6.2 Displaying the Data | 276 | ||
Chapter 12 Matrices | 285 | ||
12.1 Concept: Behavioral Abstraction | 286 | ||
12.2 Matrix Operations | 286 | ||
12.2.1 Matrix Multiplication | 286 | ||
12.2.2 Matrix Division | 289 | ||
12.2.3 Matrix Exponentiation | 289 | ||
12.3 Implementation | 289 | ||
12.3.1 Matrix Multiplication | 290 | ||
12.3.2 Matrix Division | 291 | ||
12.4 Rotating Coordinates | 292 | ||
12.4.1 2-D Rotation | 293 | ||
12.4.2 3-D Rotation | 296 | ||
12.5 Solving Simultaneous Linear Equations | 299 | ||
12.5.1 Intersecting Lines | 300 | ||
12.6 Engineering Examples | 301 | ||
12.6.1 Ceramic Composition | 301 | ||
12.6.2 Analyzing an Electrical Circuit | 303 | ||
Chapter 13 Images | 309 | ||
13.1 Nature of an Image | 310 | ||
13.2 Image Types | 311 | ||
13.2.1 True Color Images | 311 | ||
13.2.2 Gray Scale Images | 311 | ||
13.2.3 Color Mapped Images | 312 | ||
13.2.4 Preferred Image Format | 312 | ||
13.3 Reading, Displaying, and Writing Images | 313 | ||
13.4 Operating on Images | 313 | ||
13.4.1 Stretching or Shrinking Images | 313 | ||
13.4.2 Color Masking | 314 | ||
13.4.3 Creating a Kaleidoscope | 319 | ||
13.4.4 Images on a Surface | 321 | ||
13.5 Engineering Example—Detecting Edges | 324 | ||
Chapter 14 Processing Sound | 333 | ||
14.1 The Physics of Sound | 334 | ||
14.2 Recording and Playback | 334 | ||
14.3 Implementation | 335 | ||
14.4 Time Domain Operations | 336 | ||
14.4.1 Slicing and Concatenating Sound | 336 | ||
14.4.2 Musical Background | 339 | ||
14.4.3 Changing Sound Frequency | 340 | ||
14.5 The Fast Fourier Transform | 342 | ||
14.5.1 Background | 343 | ||
14.5.2 Implementation | 344 | ||
14.5.3 Simple Spectral Analysis | 345 | ||
14.6 Frequency Domain Operations | 346 | ||
14.7 Engineering Example—Music Synthesizer | 350 | ||
Chapter 15 Numerical Methods | 357 | ||
15.1 Interpolation | 358 | ||
15.1.1 Linear Interpolation | 358 | ||
15.1.2 Cubic Spline Interpolation | 361 | ||
15.1.3 Extrapolation | 362 | ||
15.2 Curve Fitting | 363 | ||
15.2.1 Linear Regression | 363 | ||
15.2.2 Polynomial Regression | 365 | ||
15.2.3 Practical Application | 367 | ||
15.3 Numerical Integration | 369 | ||
15.3.1 Determination of the Complete Integral | 369 | ||
15.3.2 Continuous Integration Problems | 371 | ||
15.4 Numerical Differentiation | 374 | ||
15.4.1 Difference Expressions | 374 | ||
15.5 Analytical Operations | 375 | ||
15.5.1 Analytical Integration | 375 | ||
15.5.2 Analytical Differentiation | 375 | ||
15.6 Implementation | 375 | ||
15.7 Engineering Example—Shaping the Synthesizer Notes | 377 | ||
Chapter 16 Sorting | 385 | ||
16.1 Measuring Algorithm Cost | 386 | ||
16.1.1 Specific Big O Examples | 387 | ||
16.1.2 Analyzing Complex Algorithms | 388 | ||
16.2 Algorithms for Sorting Data | 389 | ||
16.2.1 Insertion Sort | 389 | ||
16.2.2 Bubble Sort | 391 | ||
16.2.3 Quick Sort | 393 | ||
16.2.4 Merge Sort | 395 | ||
16.2.5 Radix Sort | 397 | ||
16.3 Performance Analysis | 398 | ||
16.4 Applications of Sorting Algorithms | 400 | ||
16.4.1 Using sort(…) | 400 | ||
16.4.2 Insertion Sort | 400 | ||
16.4.3 Bubble Sort | 401 | ||
16.4.4 Quick Sort | 401 | ||
16.4.5 Merge Sort | 401 | ||
16.4.6 Radix Sort | 401 | ||
16.5 Engineering Example—A Selection of Countries | 402 | ||
Chapter 17 Processing Graphs | 407 | ||
17.1 Queues | 408 | ||
17.1.1 The Nature of a Queue | 408 | ||
17.1.2 Implementing Queues | 408 | ||
17.1.3 Priority Queues | 409 | ||
17.1.4 Testing Queues | 411 | ||
17.2 Graphs | 414 | ||
17.2.1 Graph Examples | 414 | ||
17.2.2 Processing Graphs | 415 | ||
17.2.3 Building Graphs | 416 | ||
17.2.4 Traversing Graphs | 419 | ||
17.2.5 Searching Graphs | 421 | ||
17.3 Minimum Spanning Trees | 422 | ||
17.4 Finding Paths through a Graph | 424 | ||
17.4.1 Exact Algorithms | 425 | ||
17.4.2 Breadth-First Search (BFS) | 425 | ||
17.4.3 Dijkstra’s Algorithm | 426 | ||
17.4.4 Approximation Algorithm | 429 | ||
17.4.5 Testing Graph Search Algorithms | 431 | ||
17.5 Engineering Applications | 433 | ||
17.5.1 Simple Applications | 433 | ||
17.5.2 Complex Extensions | 433 | ||
Appendices | A-1 | ||
Appendix A: MATLAB Special Characters, Reserved Words, and Functions | A-1 | ||
Appendix B: The ASCII Character Set | B-1 | ||
Appendix C: Internal Number Representation | C-1 | ||
Appendix D: Answers to True or False and Fill in the Blanks | D-1 | ||
Index | I-1 | ||
A | I-1 | ||
B | I-2 | ||
C | I-2 | ||
D | I-2 | ||
E | I-3 | ||
F | I-3 | ||
G | I-5 | ||
H | I-5 | ||
I | I-5 | ||
J | I-5 | ||
K | I-5 | ||
L | I-5 | ||
M | I-6 | ||
N | I-6 | ||
O | I-6 | ||
P | I-6 | ||
Q | I-7 | ||
R | I-7 | ||
S | I-7 | ||
T | I-8 | ||
U | I-8 | ||
V | I-8 | ||
W | I-8 | ||
X | I-8 | ||
Y | I-8 | ||
Z | I-8 |