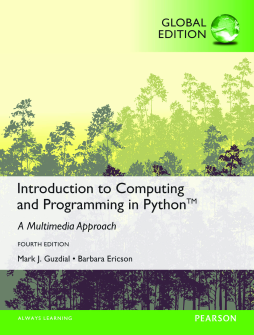
BOOK
Introduction to Computing and Programming in Python, Global Edition
Mark J. Guzdial | Barbara Ericson
(2016)
Additional Information
Book Details
Abstract
For courses in Computer Programming with Python.
This package includes MyProgrammingLab®
Social Computing and Programming with Python
Introduction to Computing and Programming in Python is a uniquely researched and up-to-date volume that is widely recognized for its successful introduction to the subject of Media Computation. Emphasizing creativity, classroom interaction, and in-class programming examples, Introduction to Computing and Programming in Python takes a bold and unique approach to computation that engages students and applies the subject matter to the relevancy of digital media. The Fourth Edition teaches students to program in an effort to communicate via social computing outlets, providing a unique approach that serves the interests of a broad range of students.
Personalize Learning with MyProgrammingLab®
This package includes MyProgrammingLab, an online homework, tutorial, and assessment program designed to work with this text to engage students and improve results. Within its structured environment, students practice what they learn, test their understanding, and pursue a personalized study plan that helps them better absorb course material and understand difficult concepts.
MyProgrammingLab should only be purchased when required by an instructor. Please be sure you have the correct ISBN and Course ID. Instructors, contact your Pearson representative for more information.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Title Page | 1 | ||
Copyright Page | 2 | ||
ACKNOWLEDGMENTS | 16 | ||
Contents | 5 | ||
Preface for the Fourth Edition | 13 | ||
Preface to the First Edition | 18 | ||
About the Authors | 24 | ||
1 INTRODUCTION | 25 | ||
1 Introduction to Computer Science and Media Computation | 27 | ||
1.1 What Is Computer Science About? | 27 | ||
1.2 Programming Languages | 30 | ||
1.3 What Computers Understand | 33 | ||
1.4 Media Computation: Why Digitize Media? | 35 | ||
1.5 Computer Science for Everyone | 37 | ||
1.5.1 It’s About Communication | 37 | ||
1.5.2 It’s About Process | 37 | ||
1.5.3 You Will Probably Need It | 38 | ||
2 Introduction to Programming | 42 | ||
2.1 Programming Is About Naming | 42 | ||
2.1.1 Files and Their Names | 44 | ||
2.2 Programming in Python | 45 | ||
2.3 Programming in JES | 46 | ||
2.4 Media Computation in JES | 47 | ||
2.4.1 Showing a Picture | 51 | ||
2.4.2 Playing a Sound | 54 | ||
2.4.3 Naming Values | 54 | ||
2.5 Making a Program | 57 | ||
2.5.1 Functions: Real Math-Like Functions That Take Input | 61 | ||
3 Creating and Modifying Text | 68 | ||
3.1 Strings: Making Human Text in a Computer | 68 | ||
3.1.1 Making Strings from Strings: Telling Stories | 70 | ||
3.2 Taking Strings Apart with For | 73 | ||
3.2.1 Testing the Pieces | 75 | ||
3.2.2 Taking String Apart, and Putting Strings Together | 78 | ||
3.2.3 Taking Strings Apart with Indices | 81 | ||
3.2.4 Mirroring, Reversing, and Separating Strings with Index | 83 | ||
3.2.5 Encoding and Decoding Strings Using a Keyword Cipher | 85 | ||
3.3 Taking Strings Apart by Words | 87 | ||
3.4 What’s Inside a String | 90 | ||
3.5 What a Computer Can Do | 91 | ||
4 Modifying Pictures Using Loops | 98 | ||
4.1 How Pictures Are Encoded | 99 | ||
4.2 Manipulating Pictures | 104 | ||
4.2.1 Exploring Pictures | 108 | ||
4.3 Changing Color Values | 110 | ||
4.3.1 Using Loops in Pictures | 110 | ||
4.3.2 Increasing/Decreasing Red (Green, Blue) | 112 | ||
4.3.3 Testing the Program: Did That Really Work? | 117 | ||
4.3.4 Changing One Color at a Time | 118 | ||
4.4 Creating a Sunset | 119 | ||
4.4.1 Making Sense of Functions | 119 | ||
4.5 Lightening and Darkening | 124 | ||
4.6 Creating a Negative | 125 | ||
4.7 Converting to Grayscale | 126 | ||
4.8 Specifying Pixels by Index | 128 | ||
5 Picture Techniques with Selection | 138 | ||
5.1 Replacing Colors: Red-Eye, Sepia Tones, and Posterizing | 138 | ||
5.1.1 Reducing Red-Eye | 142 | ||
5.1.2 Sepia-Toned and Posterized Pictures: Using Conditionals to Choose the Color | 144 | ||
5.2 Comparing Pixels: Edge Detection | 150 | ||
5.3 Background Subtraction | 153 | ||
5.4 Chromakey | 156 | ||
5.5 Coloring in ranges | 161 | ||
5.5.1 Adding a Border | 161 | ||
5.5.2 Lightening the Right Half of a Picture | 162 | ||
5.6 Selecting without Retesting | 163 | ||
6 Modifying Pixels by Position | 169 | ||
6.1 Processing Pixels Faster | 169 | ||
6.1.1 Looping across the Pixels with Range | 171 | ||
6.1.2 Writing Faster Pixel Loops | 173 | ||
6.2 Mirroring a Picture | 175 | ||
6.3 Copying and Transforming Pictures | 182 | ||
6.3.1 Copying | 183 | ||
6.3.2 Copying Smaller and Modifying | 189 | ||
6.3.3 Copying and Referencing | 191 | ||
6.3.4 Creating a Collage | 193 | ||
6.3.5 General Copying | 196 | ||
6.3.6 Rotation | 197 | ||
6.3.7 Scaling | 200 | ||
6.4 Combining Pixels: Blurring | 205 | ||
6.5 Blending Pictures | 208 | ||
6.6 Drawing on Images | 210 | ||
6.6.1 Drawing with Drawing Commands | 212 | ||
6.6.2 Vector and Bitmap Representations | 213 | ||
6.7 Programs as Specifying Drawing Process | 215 | ||
6.7.1 Why Do We Write Programs? | 216 | ||
2 SOUND | 225 | ||
7 Modifying Sounds Using Loops | 227 | ||
7.1 How Sound Is Encoded | 227 | ||
7.1.1 The Physics of Sound | 227 | ||
7.1.2 Investigating Different Sounds | 230 | ||
7.1.3 Encoding the Sound | 235 | ||
7.1.4 Binary Numbers and Two’s Complement | 236 | ||
7.1.5 Storing Digitized Sounds | 237 | ||
7.2 Manipulating Sounds | 239 | ||
7.2.1 Open Sounds and Manipulating Samples | 239 | ||
7.2.2 Using the JES Media Tools | 242 | ||
7.2.3 Looping | 243 | ||
7.3 Changing the Volume of Sounds | 244 | ||
7.3.1 Increasing Volume | 244 | ||
7.3.2 Did That Really Work? | 245 | ||
7.3.3 Decreasing Volume | 249 | ||
7.3.4 Using Array Index Notation | 250 | ||
7.3.5 Making Sense of Functions in Sounds | 251 | ||
7.4 Normalizing Sounds | 251 | ||
7.4.1 Generating Clipping | 253 | ||
8 Modifying Samples in a Range | 259 | ||
8.1 Manipulating Different Sections of the Sound Differently | 259 | ||
8.1.1 Revisiting Index Array Notation | 260 | ||
8.2 Splicing Sounds | 262 | ||
8.3 General Clip and Copy | 269 | ||
8.4 Reversing Sounds | 271 | ||
8.5 Mirroring | 273 | ||
8.6 On Functions and Scope | 273 | ||
9 Making Sounds by Combining Pieces | 279 | ||
9.1 Composing Sounds Through Addition | 279 | ||
9.2 Blending Sounds | 280 | ||
9.3 Creating an Echo | 282 | ||
9.3.1 Creating Multiple Echoes | 284 | ||
9.3.2 Creating Chords | 284 | ||
9.4 How Sampling Keyboards Work | 285 | ||
9.4.1 Sampling as an Algorithm | 289 | ||
9.5 Additive Synthesis | 289 | ||
9.5.1 Making Sine Waves | 289 | ||
9.5.2 Adding Sine Waves Together | 291 | ||
9.5.3 Checking Our Result | 292 | ||
9.5.4 Square Waves | 293 | ||
9.5.5 Triangular Waves | 295 | ||
9.6 Modern Music Synthesis | 297 | ||
9.6.1 MP3 | 297 | ||
9.6.2 MIDI | 298 | ||
10 Building Bigger Programs | 302 | ||
10.1 Designing Programs Top-Down | 303 | ||
10.1.1 A Top-Down Design Example | 304 | ||
10.1.2 Designing the Top-Level Function | 305 | ||
10.1.3 Writing the Subfunctions | 307 | ||
10.2 Designing Programs Bottom-Up | 311 | ||
10.2.1 An Example Bottom-Up Process | 312 | ||
10.3 Testing Your Program | 312 | ||
10.3.1 Testing the Edge Conditions | 314 | ||
10.4 Tips on Debugging | 315 | ||
10.4.1 Finding Which Statement to Worry About | 316 | ||
10.4.2 Seeing the Variables | 316 | ||
10.4.3 Debugging the Adventure Game | 318 | ||
10.5 Algorithms and Design | 321 | ||
10.6 Connecting to Data Outside a Function | 322 | ||
10.7 Running Programs Outside of JES | 326 | ||
3 TEXT, FILES, NETWORKS, DATABASES, AND UNIMEDIA | 333 | ||
11 Manipulating Text with Methods and Files | 335 | ||
11.1 Text as Unimedia | 335 | ||
11.2 Manipulating Parts of Strings | 336 | ||
11.2.1 String Methods: Introducing Objects and Dot Notation | 337 | ||
11.2.2 Lists: Powerful, Structured Text | 339 | ||
11.2.3 Strings Have No Font | 341 | ||
11.3 Files: Places to Put Your Strings and Other Stuff | 341 | ||
11.3.1 Opening and Manipulating Files | 343 | ||
11.3.2 Generating Form Letters | 344 | ||
11.3.3 Reading and Manipulating Data from the Internet | 345 | ||
11.3.4 Scraping Information from a Web Page | 348 | ||
11.3.5 Reading CSV Data | 349 | ||
11.3.6 Writing Out Programs | 351 | ||
11.4 The Python Standard Library | 352 | ||
11.4.1 More on Import and Your Own Modules | 353 | ||
11.4.2 Adding Unpredictably to Your Program with Random | 354 | ||
11.4.3 Reading CSV Files with a Library | 356 | ||
11.4.4 A Sampling of Python Standard Libraries | 356 | ||
12 Advanced Text Techniques: Web and Information | 361 | ||
12.1 Networks: Getting Our Text from the Web | 361 | ||
12.1.1 Automating Access to CSV Data | 365 | ||
12.1.2 Accessing FTP | 367 | ||
12.2 Using Text to Shift Between Media | 368 | ||
12.3 Moving Information Between Media | 371 | ||
12.4 Using Lists as Structured Text for Media Representations | 374 | ||
12.5 Hiding Information ina Picture | 375 | ||
12.5.1 Hiding a Sound Inside a Picture | 377 | ||
13 Making Text for the Web | 383 | ||
13.1 HTML: The Notation of the Web | 383 | ||
13.2 Writing Programs to Generate HTML | 388 | ||
13.2.1 Making Home Pages | 390 | ||
13.3 Databases: A Place to Store Our Text | 393 | ||
13.3.1 Relational Databases | 395 | ||
13.3.2 An Example Relational Database Using Hash Tables | 396 | ||
13.3.3 Working with SQL | 399 | ||
13.3.4 Using a Database to Build Web Pages | 401 | ||
4 MOVIES | 407 | ||
14 Creating and Modifying Movies | 409 | ||
14.1 Generating Animations | 410 | ||
14.2 Working with Video Source | 419 | ||
14.2.1 Video Manipulating Examples | 419 | ||
14.3 Building a Video Effect Bottom-Up | 423 | ||
15 Speed | 430 | ||
15.1 Focusing on Computer Science | 430 | ||
15.2 What Makes Programs Fast? | 430 | ||
15.2.1 What Computers Really Understand | 431 | ||
15.2.2 Compilers and Interpreters | 432 | ||
15.2.3 What Limits Computer Speed? | 436 | ||
15.2.4 Does It Really Make a Difference? | 438 | ||
15.2.5 Making Searching Faster | 441 | ||
15.2.6 Algorithms That Never Finish or Can’t Be Written | 443 | ||
15.2.7 Why Is Photoshop Faster than JES? | 444 | ||
15.3 What Makes a Computer Fast? | 444 | ||
15.3.1 Clock Rates and Actual Computation | 445 | ||
15.3.2 Storage: What Makes a Computer Slow? | 446 | ||
15.3.3 Display | 447 | ||
16 Functional Programming | 450 | ||
16.1 Using Functions to Make Programming Easier | 450 | ||
16.2 Functional Programming with Map and Reduce | 454 | ||
16.3 Functional Programming for Media | 457 | ||
16.3.1 Media Manipulation without Changing State | 458 | ||
16.4 Recursion: A Powerful Idea | 459 | ||
16.4.1 Recursive Directory Traversals | 464 | ||
16.4.2 Recursive Media Functions | 466 | ||
17 Object-Oriented Programming | 471 | ||
17.1 History of Objects | 471 | ||
17.2 Working with Turtles | 473 | ||
17.2.1 Classes and Objects | 473 | ||
17.2.2 Sending Messages to Objects | 474 | ||
17.2.3 Objects Control Their State | 476 | ||
17.3 Teaching Turtles New Tricks | 478 | ||
17.3.1 Overriding an Existing Turtle Method | 480 | ||
17.3.2 Working with Multiple Turtles at Once | 481 | ||
17.3.3 Turtles with Pictures | 483 | ||
17.3.4 Dancing Turtles | 484 | ||
17.3.5 Recursion and Turtles | 487 | ||
17.4 An Object-Oriented Slide Show | 488 | ||
17.4.1 Making the Slide Class More Object-Oriented | 491 | ||
17.5 Object-Oriented Media | 493 | ||
17.6 Joe the Box | 498 | ||
17.7 Why Objects? | 499 | ||
APPENDIX | 506 | ||
A Quick Reference to Python | 506 | ||
A.1 Variables | 506 | ||
A.2 Function Creation | 507 | ||
A.3 Loops and Conditionals | 507 | ||
A.4 Operators andRepresentation Functions | 508 | ||
A.5 Numeric Functions | 509 | ||
A.6 Sequence Operations | 509 | ||
A.7 String Escapes | 509 | ||
A.8 Useful String Methods | 509 | ||
A.9 Files | 510 | ||
A.10 Lists | 510 | ||
A.11 Dictionaries, Hash Tables, or Associative Arrays | 510 | ||
A.12 External Modules | 510 | ||
A.13 Classes | 511 | ||
A.14 Functional Methods | 511 | ||
Bibliography | 512 | ||
Index | 515 | ||
A | 515 | ||
B | 515 | ||
C | 516 | ||
D | 516 | ||
E | 516 | ||
F | 517 | ||
G | 517 | ||
H | 518 | ||
I | 518 | ||
J | 518 | ||
K | 518 | ||
L | 518 | ||
M | 519 | ||
N | 519 | ||
O | 519 | ||
P | 520 | ||
Q | 520 | ||
R | 520 | ||
S | 521 | ||
T | 522 | ||
U | 522 | ||
V | 522 | ||
W | 522 | ||
X | 523 | ||
Z | 523 |