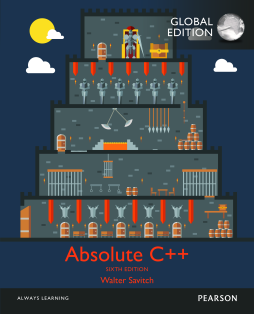
Additional Information
Book Details
Abstract
For courses in computer programming for business and engineering.
Introduction and Advancement in C++ Programming
Absolute C++ is a comprehensive introduction to the C++ programming language. The text is organized around the specific use of C++, providing students with an opportunity to master the language completely. Adaptable to a wide range of users, the text is appropriate for beginner to advanced programmers familiar with the C++ language.
The Sixth Edition covers everything from basic syntax to more advanced topics, such as polymorphism, exception handling, and the Standard Template Library, making it ideal for both beginner and intermediate students. Updated to reflect the most recent changes in the C++ language, Absolute C++ teaches students to become proficient in a widely used and important programming language.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Title Page | 3 | ||
Copyright Page | 4 | ||
Preface | 5 | ||
Acknowledgments | 7 | ||
Brief Contents | 13 | ||
Contents | 15 | ||
Chapter 1 C++ Basics | 29 | ||
1.1 INTRODUCTION TO C | 30 | ||
Origins of the C++ Language | 30 | ||
C++ and Object-Oriented Programming | 31 | ||
The Character of C | 31 | ||
C++ Terminology | 32 | ||
A Sample C++ Program | 32 | ||
TIP: Compiling C++ Programs | 33 | ||
1.2 VARIABLES, EXPRESSIONS, AND ASSIGNMENT STATEMENTS | 34 | ||
Identifiers | 35 | ||
Variables | 36 | ||
Assignment Statements | 39 | ||
Introduction to the string class | 40 | ||
PITFALL: Uninitialized Variables | 41 | ||
TIP: Use Meaningful Names | 42 | ||
More Assignment Statements | 42 | ||
Assignment Compatibility | 43 | ||
Literals | 44 | ||
Escape Sequences | 46 | ||
Raw String Literals | 47 | ||
Naming Constants | 47 | ||
Arithmetic Operators and Expressions | 48 | ||
Integer and Floating-Point Division | 50 | ||
PITFALL: Division with Whole Numbers | 51 | ||
Type Casting | 52 | ||
Increment and Decrement Operators | 54 | ||
PITFALL: Order of Evaluation | 56 | ||
1.3 CONSOLE INPUT/OUTPUT | 57 | ||
Output Using cout | 57 | ||
New Lines in Output | 58 | ||
TIP: End Each Program with \\n or endl | 59 | ||
Formatting for Numbers with a Decimal Point | 59 | ||
Output with cerr | 61 | ||
Input Using cin | 61 | ||
TIP: Line Breaks in I/O | 64 | ||
1.4 PROGRAM STYLE | 65 | ||
Comments | 65 | ||
1.5 LIBRARIES AND NAMESPACES | 66 | ||
Libraries and include Directives | 66 | ||
Namespaces | 66 | ||
PITFALL: Problems with Library Names | 67 | ||
Chapter Summary | 68 | ||
Answers to Self-Test Exercises | 69 | ||
Programming Projects | 71 | ||
Chapter 2 Flow of Control | 75 | ||
2.1 BOOLEAN EXPRESSIONS | 76 | ||
Building Boolean Expressions | 76 | ||
PITFALL: Strings of Inequalities | 77 | ||
Evaluating Boolean Expressions | 78 | ||
Precedence Rules | 80 | ||
PITFALL: Integer Values Can Be Used as Boolean Values | 84 | ||
2.2 BRANCHING MECHANISMS | 86 | ||
if-else Statements | 86 | ||
Compound Statements | 88 | ||
PITFALL: Using = in Place of = = | 89 | ||
Omitting the else | 91 | ||
Nested Statements | 91 | ||
Multiway if-else Statement | 91 | ||
The switch Statement | 92 | ||
PITFALL: Forgetting a break in a switch Statement | 95 | ||
TIP: Use switch Statements for Menus | 95 | ||
Enumeration Types | 95 | ||
The Conditional Operator | 97 | ||
2.3 LOOPS | 97 | ||
The while and do-while Statements | 98 | ||
Increment and Decrement Operators Revisited | 101 | ||
The Comma Operator | 102 | ||
The for Statement | 104 | ||
TIP: Repeat-N-Times Loops | 106 | ||
PITFALL: Extra Semicolon in a for Statement | 107 | ||
PITFALL: Infinite Loops | 107 | ||
The break and continue Statements | 110 | ||
Nested Loops | 113 | ||
2.4 INTRODUCTION TO FILE INPUT | 113 | ||
Reading From a Text File Using ifstream | 114 | ||
Chapter Summary | 117 | ||
Answers to Self-Test Exercises | 117 | ||
Programming Projects | 123 | ||
Chapter 3 Function Basics | 129 | ||
3.1 PREDEFINED FUNCTIONS | 130 | ||
Predefined Functions That Return a Value | 130 | ||
Predefined void Functions | 135 | ||
A Random Number Generator | 137 | ||
3.2 PROGRAMMER-DEFINED FUNCTIONS | 141 | ||
Defining Functions That Return a Value | 142 | ||
Alternate Form for Function Declarations | 144 | ||
PITFALL: Arguments in the Wrong Order | 145 | ||
PITFALL: Use of the Terms Parameter and Argument | 145 | ||
Functions Calling Functions | 145 | ||
EXAMPLE: A Rounding Function | 145 | ||
Functions That Return a Boolean Value | 148 | ||
Defining void Functions | 149 | ||
return Statements in void Functions | 151 | ||
Preconditions and Postconditions | 151 | ||
main Is a Function | 153 | ||
Recursive Functions | 153 | ||
3.3 SCOPE RULES | 155 | ||
Local Variables | 155 | ||
Procedural Abstraction | 157 | ||
Global Constants and Global Variables | 158 | ||
Blocks | 161 | ||
Nested Scopes | 162 | ||
TIP: Use Function Calls in Branching and Loop Statements | 162 | ||
Variables Declared in a for Loop | 163 | ||
Chapter Summary | 164 | ||
Answers to Self-Test Exercises | 164 | ||
Programming Projects | 168 | ||
Chapter 4 Parameters and Overloading | 175 | ||
4.1 PARAMETERS | 176 | ||
Call-by-Value Parameters | 176 | ||
A First Look at Call-by-Reference Parameters | 178 | ||
Call-by-Reference Mechanism in Detail | 181 | ||
Constant Reference Parameters | 183 | ||
EXAMPLE: The swapValues Function | 183 | ||
TIP: Think of Actions, Not Code | 184 | ||
Mixed Parameter Lists | 185 | ||
TIP: What Kind of Parameter to Use | 186 | ||
PITFALL: Inadvertent Local Variables | 188 | ||
TIP: Choosing Formal Parameter Names | 189 | ||
EXAMPLE: Buying Pizza | 190 | ||
4.2 OVERLOADING AND DEFAULT ARGUMENTS | 193 | ||
Introduction to Overloading | 193 | ||
PITFALL: Automatic Type Conversion and Overloading | 196 | ||
Rules for Resolving Overloading | 197 | ||
EXAMPLE: Revised Pizza-Buying Program | 199 | ||
Default Arguments | 201 | ||
4.3 TESTING AND DEBUGGING FUNCTIONS | 203 | ||
The assert Macro | 203 | ||
Stubs and Drivers | 204 | ||
Chapter Summary | 207 | ||
Answers to Self-Test Exercises | 207 | ||
Programming Projects | 209 | ||
Chapter 5 Arrays | 215 | ||
5.1 INTRODUCTION TO ARRAYS | 216 | ||
Declaring and Referencing Arrays | 216 | ||
TIP: Use for Loops with Arrays | 219 | ||
PITFALL: Array Indexes Always Start with Zero | 219 | ||
TIP: Use a Defined Constant for the Size of an Array | 219 | ||
Arrays in Memory | 220 | ||
PITFALL: Array Index out of Range | 222 | ||
The Range-Based for Loop | 222 | ||
Initializing Arrays | 223 | ||
5.2 ARRAYS IN FUNCTIONS | 225 | ||
Indexed Variables as Function Arguments | 225 | ||
Entire Arrays as Function Arguments | 226 | ||
The const Parameter Modifier | 230 | ||
PITFALL: Inconsistent Use of const Parameters | 231 | ||
Functions That Return an Array | 232 | ||
EXAMPLE: Production Graph | 232 | ||
5.3 PROGRAMMING WITH ARRAYS | 237 | ||
Partially Filled Arrays | 237 | ||
TIP: Do Not Skimp on Formal Parameters | 238 | ||
EXAMPLE: Searching an Array | 241 | ||
EXAMPLE: Sorting an Array | 243 | ||
EXAMPLE: Bubble Sort | 247 | ||
5.4 MULTIDIMENSIONAL ARRAYS | 251 | ||
Multidimensional Array Basics | 251 | ||
Multidimensional Array Parameters | 252 | ||
EXAMPLE: Two-Dimensional Grading Program | 253 | ||
Chapter Summary | 258 | ||
Answers to Self-Test Exercises | 259 | ||
Programming Projects | 263 | ||
Chapter 6 Structures and Classes | 273 | ||
6.1 STRUCTURES | 274 | ||
Structure Types | 276 | ||
PITFALL: Forgetting a Semicolon in a Structure Definition | 280 | ||
Structures as Function Arguments | 280 | ||
TIP: Use Hierarchical Structures | 281 | ||
Initializing Structures | 283 | ||
6.2 CLASSES | 286 | ||
Defining Classes and Member Functions | 286 | ||
Encapsulation | 292 | ||
Public and Private Members | 293 | ||
Accessor and Mutator Functions | 296 | ||
TIP: Separate Interface and Implementation | 298 | ||
TIP: A Test for Encapsulation | 299 | ||
Structures versus Classes | 300 | ||
TIP: Thinking Objects | 302 | ||
Chapter Summary | 302 | ||
Answers to Self-Test Exercises | 303 | ||
Programming Projects | 305 | ||
Chapter 7 Constructors and Other Tools | 309 | ||
7.1 CONSTRUCTORS | 310 | ||
Constructor Definitions | 310 | ||
PITFALL: Constructors with No Arguments | 315 | ||
Explicit Constructor Calls | 316 | ||
TIP: Always Include a Default Constructor | 317 | ||
EXAMPLE: BankAccount Class | 319 | ||
Class Type Member Variables | 326 | ||
Member Initializers and Constructor Delegation in C++11 | 329 | ||
7.2 MORE TOOLS | 330 | ||
The const Parameter Modifier | 330 | ||
PITFALL: Inconsistent Use of const | 332 | ||
Inline Functions | 336 | ||
Static Members | 338 | ||
Nested and Local Class Definitions | 341 | ||
7.3 VECTORS—A PREVIEW OF THE STANDARD TEMPLATE LIBRARY | 342 | ||
Vector Basics | 342 | ||
PITFALL: Using Square Brackets beyond the Vector Size | 344 | ||
TIP: Vector Assignment Is Well Behaved | 346 | ||
Efficiency Issues | 346 | ||
Chapter Summary | 348 | ||
Answers to Self-Test Exercises | 348 | ||
Programming Projects | 350 | ||
Chapter 8 Operator Overloading, Friends, and References | 355 | ||
8.1 BASIC OPERATOR OVERLOADING | 356 | ||
Overloading Basics | 357 | ||
TIP: A Constructor Can Return an Object | 362 | ||
Returning by const Value | 363 | ||
Overloading Unary Operators | 366 | ||
Overloading as Member Functions | 366 | ||
TIP: A Class Has Access to All Its Objects | 369 | ||
Overloading Function Application ( ) | 369 | ||
PITFALL: Overloading &&,||, and the Comma Operator | 370 | ||
8.2 FRIEND FUNCTIONS AND AUTOMATIC TYPE CONVERSION | 370 | ||
Constructors for Automatic Type Conversion | 370 | ||
PITFALL: Member Operators and Automatic Type Conversion | 371 | ||
Friend Functions | 372 | ||
Friend Classes | 375 | ||
PITFALL: Compilers without Friends | 376 | ||
8.3 REFERENCES AND MORE OVERLOADED OPERATORS | 377 | ||
References | 378 | ||
TIP: Returning Member Variables of a Class Type | 379 | ||
Overloading >> and << | 380 | ||
TIP: What Mode of Returned Value to Use | 386 | ||
The Assignment Operator | 389 | ||
Overloading the Increment and Decrement Operators | 389 | ||
Overloading the Array Operator [ ] | 392 | ||
Overloading Based on L-Value versus R-Value | 394 | ||
Chapter Summary | 394 | ||
Answers to Self-Test Exercises | 395 | ||
Programming Projects | 397 | ||
Chapter 9 Strings | 401 | ||
9.1 AN ARRAY TYPE FOR STRINGS | 402 | ||
C-String Values and C-String Variables | 403 | ||
PITFALL: Using = and == with C-strings | 406 | ||
Other Functions in |
408 | ||
EXAMPLE: Command-Line Arguments | 410 | ||
C-String Input and Output | 413 | ||
9.2 CHARACTER MANIPULATION TOOLS | 415 | ||
Character I/O | 415 | ||
The Member Functions get and put | 416 | ||
EXAMPLE: Checking Input Using a Newline Function | 418 | ||
PITFALL: Unexpected '\\n' in Input | 420 | ||
The putback, peek, and ignore Member Functions | 421 | ||
Character-Manipulating Functions | 423 | ||
PITFALL: toupper and tolower Return int Values | 425 | ||
9.3 THE STANDARD CLASS String | 427 | ||
Introduction to the Standard Class string | 427 | ||
I/O with the Class string | 430 | ||
TIP: More Versions of getline | 433 | ||
String Processing with the Class string | 435 | ||
EXAMPLE: Palindrome Testing | 438 | ||
Converting between string Objects and C-Strings | 442 | ||
Converting between string Objects and Numbers | 442 | ||
Chapter Summary | 443 | ||
Answers to Self-Test Exercises | 444 | ||
Programming Projects | 447 | ||
Chapter 10 Pointers and Dynamic Arrays | 453 | ||
10.1 POINTERS | 454 | ||
Pointer Variables | 455 | ||
Basic Memory Management | 463 | ||
nullptr | 465 | ||
PITFALL: Dangling Pointers | 466 | ||
Dynamic Variables and Automatic Variables | 466 | ||
TIP: Define Pointer Types | 467 | ||
PITFALL: Pointers as Call-by-Value Parameters | 469 | ||
Uses for Pointers | 470 | ||
10.2 DYNAMIC ARRAYS | 471 | ||
Array Variables and Pointer Variables | 471 | ||
Creating and Using Dynamic Arrays | 473 | ||
EXAMPLE: A Function That Returns an Array | 476 | ||
Pointer Arithmetic | 478 | ||
Multidimensional Dynamic Arrays | 479 | ||
10.3 CLASSES, POINTERS, AND DYNAMIC ARRAYS | 482 | ||
The -> Operator | 482 | ||
The this Pointer | 483 | ||
Overloading the Assignment Operator | 483 | ||
EXAMPLE: A Class for Partially Filled Arrays | 490 | ||
Destructors | 493 | ||
Copy Constructors | 494 | ||
Chapter Summary | 499 | ||
Answers to Self-Test Exercises | 499 | ||
Programming Projects | 501 | ||
Chapter 11 Separate Compilation and Namespaces | 505 | ||
11.1 SEPARATE COMPILATION | 506 | ||
Encapsulation Reviewed | 507 | ||
Header Files and Implementation Files | 507 | ||
EXAMPLE: DigitalTime Class | 516 | ||
TIP: Reusable Components | 517 | ||
Using ifndef | 517 | ||
TIP: Defining Other Libraries | 519 | ||
11.2 NAMESPACES | 521 | ||
Namespaces and using Directives | 521 | ||
Creating a Namespace | 523 | ||
using Declarations | 526 | ||
Qualifying Names | 527 | ||
TIP: Choosing a Name for a Namespace | 529 | ||
EXAMPLE: A Class Definition in a Namespace | 530 | ||
Unnamed Namespaces | 531 | ||
PITFALL: Confusing the Global Namespace and the Unnamed Namespace | 537 | ||
TIP: Unnamed Namespaces Replace the static Qualifier | 538 | ||
TIP: Hiding Helping Functions | 538 | ||
Nested Namespaces | 539 | ||
TIP: What Namespace Specification Should You Use? | 539 | ||
Chapter Summary | 542 | ||
Answers to Self-Test Exercises | 542 | ||
Programming Projects | 544 | ||
Chapter 12 Streams and File I/O | 549 | ||
12.1 I/O STREAMS | 551 | ||
File I/O | 551 | ||
PITFALL: Restrictions on Stream Variables | 556 | ||
Appending to a File | 556 | ||
TIP: Another Syntax for Opening a File | 558 | ||
TIP: Check That a File Was Opened Successfully | 560 | ||
Character I/O | 562 | ||
Checking for the End of a File | 563 | ||
12.2 TOOLS FOR STREAM I/O | 567 | ||
File Names as Input | 567 | ||
Formatting Output with Stream Functions | 568 | ||
Manipulators | 572 | ||
Saving Flag Settings | 573 | ||
More Output Stream Member Functions | 574 | ||
EXAMPLE: Cleaning Up a File Format | 576 | ||
EXAMPLE: Editing a Text File | 578 | ||
12.3 STREAM HIERARCHIES: A PREVIEW OF INHERITANCE | 581 | ||
Inheritance among Stream Classes | 581 | ||
EXAMPLE: Another newLine Function | 583 | ||
Parsing Strings with the stringstream Class | 587 | ||
12.4 RANDOM ACCESS TO FILES | 590 | ||
Chapter Summary | 592 | ||
Answers to Self-Test Exercises | 592 | ||
Programming Projects | 595 | ||
Chapter 13 Recursion | 605 | ||
13.1 RECURSIVE Void FUNCTIONS | 607 | ||
EXAMPLE: Vertical Numbers | 607 | ||
Tracing a Recursive Call | 610 | ||
A Closer Look at Recursion | 613 | ||
PITFALL: Infinite Recursion | 614 | ||
Stacks for Recursion | 616 | ||
PITFALL: Stack Overflow | 617 | ||
Recursion versus Iteration | 618 | ||
13.2 RECURSIVE FUNCTIONS THAT RETURN A VALUE | 619 | ||
General Form for a Recursive Function That Returns a Value | 619 | ||
EXAMPLE: Another Powers Function | 620 | ||
Mutual Recursion | 625 | ||
13.3 THINKING RECURSIVELY | 627 | ||
Recursive Design Techniques | 627 | ||
Binary Search | 628 | ||
Coding | 630 | ||
Checking the Recursion | 634 | ||
Efficiency | 634 | ||
Chapter Summary | 636 | ||
Answers to Self-Test Exercises | 637 | ||
Programming Projects | 641 | ||
Chapter 14 Inheritance | 647 | ||
14.1 INHERITANCE BASICS | 648 | ||
Derived Classes | 648 | ||
Constructors in Derived Classes | 658 | ||
PITFALL: Use of Private Member Variables from the Base Class | 660 | ||
PITFALL: Private Member Functions Are Effectively Not Inherited | 662 | ||
The protected Qualifier | 662 | ||
Redefinition of Member Functions | 665 | ||
Redefining versus Overloading | 666 | ||
Access to a Redefined Base Function | 668 | ||
Functions That Are Not Inherited | 669 | ||
14.2 PROGRAMMING WITH INHERITANCE | 670 | ||
Assignment Operators and Copy Constructors in Derived Classes | 670 | ||
Destructors in Derived Classes | 671 | ||
EXAMPLE: Partially Filled Array with Backup | 672 | ||
PITFALL: Same Object on Both Sides of the Assignment Operator | 681 | ||
EXAMPLE: Alternate Implementation of PFArrayDBak | 681 | ||
TIP: A Class Has Access to Private Members of All Objects of the Class | 684 | ||
TIP: “Is a” versus “Has a” | 684 | ||
Protected and Private Inheritance | 685 | ||
Multiple Inheritance | 686 | ||
Chapter Summary | 687 | ||
Answers to Self-Test Exercises | 687 | ||
Programming Projects | 689 | ||
Chapter 15 Polymorphism and Virtual Functions | 697 | ||
15.1 VIRTUAL FUNCTION BASICS | 698 | ||
Late Binding | 698 | ||
Virtual Functions in C | 699 | ||
Provide Context with C++11’s override Keyword | 705 | ||
Preventing a Virtual Function from Being Overridden | 706 | ||
TIP: The Virtual Property Is Inherited | 706 | ||
TIP: When to Use a Virtual Function | 707 | ||
PITFALL: Omitting the Definition of a Virtual Member Function | 707 | ||
Abstract Classes and Pure Virtual Functions | 708 | ||
EXAMPLE: An Abstract Class | 709 | ||
15.2 POINTERS AND VIRTUAL FUNCTIONS | 711 | ||
Virtual Functions and Extended Type Compatibility | 711 | ||
PITFALL: The Slicing Problem | 715 | ||
TIP: Make Destructors Virtual | 716 | ||
Downcasting and Upcasting | 717 | ||
How C++ Implements Virtual Functions | 718 | ||
Chapter Summary | 720 | ||
Answers to Self-Test Exercises | 721 | ||
Programming Projects | 721 | ||
Chapter 16 Templates | 729 | ||
16.1 FUNCTION TEMPLATES | 730 | ||
Syntax for Function Templates | 731 | ||
PITFALL: Compiler Complications | 734 | ||
TIP: How to Define Templates | 736 | ||
EXAMPLE: A Generic Sorting Function | 737 | ||
PITFALL: Using a Template with an Inappropriate Type | 741 | ||
16.2 CLASS TEMPLATES | 743 | ||
Syntax for Class Templates | 744 | ||
EXAMPLE: An Array Template Class | 748 | ||
The vector and basic_string Templates | 754 | ||
16.3 TEMPLATES AND INHERITANCE | 754 | ||
EXAMPLE: Template Class For a Partially Filled Array with Backup | 755 | ||
Chapter Summary | 760 | ||
Answers to Self-Test Exercises | 760 | ||
Programming Projects | 764 | ||
Chapter 17 Linked Data Structures | 767 | ||
17.1 NODES AND LINKED LISTS | 769 | ||
Nodes | 769 | ||
Linked Lists | 774 | ||
Inserting a Node at the Head of a List | 776 | ||
PITFALL: Losing Nodes | 779 | ||
Inserting and Removing Nodes Inside a List | 779 | ||
PITFALL: Using the Assignment Operator with Dynamic Data Structures | 783 | ||
Searching a Linked List | 783 | ||
Doubly Linked Lists | 786 | ||
Adding a Node to a Doubly Linked List | 788 | ||
Deleting a Node from a Doubly Linked List | 788 | ||
EXAMPLE: A Generic Sorting Template Version of Linked List Tools | 795 | ||
17.2 LINKED LIST APPLICATIONS | 799 | ||
EXAMPLE: A Stack Template Class | 799 | ||
EXAMPLE: A Queue Template Class | 806 | ||
TIP: A Comment on Namespaces | 809 | ||
Friend Classes and Similar Alternatives | 810 | ||
EXAMPLE: Hash Tables With Chaining | 813 | ||
Efficiency of Hash Tables | 819 | ||
EXAMPLE: A Set Template Class | 820 | ||
Efficiency of Sets Using Linked Lists | 826 | ||
17.3 ITERATORS | 827 | ||
Pointers as Iterators | 828 | ||
Iterator Classes | 828 | ||
EXAMPLE: An Iterator Class | 830 | ||
17.4 TREES | 836 | ||
Tree Properties | 837 | ||
EXAMPLE: A Tree Template Class | 839 | ||
Chapter Summary | 844 | ||
Answers to Self-Test Exercises | 845 | ||
Programming Projects | 854 | ||
Chapter 18 Exception Handling | 861 | ||
18.1 EXCEPTION HANDLING BASICS | 863 | ||
A Toy Example of Exception Handling | 863 | ||
Defining Your Own Exception Classes | 872 | ||
Multiple Throws and Catches | 872 | ||
PITFALL: Catch the More Specific Exception First | 876 | ||
TIP: Exception Classes Can Be Trivial | 877 | ||
Throwing an Exception in a Function | 877 | ||
EXAMPLE: Returning the High Score | 879 | ||
Exception Specification | 882 | ||
PITFALL: Exception Specification in Derived Classes | 884 | ||
18.2 PROGRAMMING TECHNIQUES FOR EXCEPTION HANDLING | 885 | ||
When to Throw an Exception | 886 | ||
PITFALL: Uncaught Exceptions | 887 | ||
PITFALL: Nested try-catch Blocks | 888 | ||
PITFALL: Overuse of Exceptions | 888 | ||
Exception Class Hierarchies | 889 | ||
Testing for Available Memory | 889 | ||
Rethrowing an Exception | 890 | ||
Chapter Summary | 890 | ||
Answers to Self-Test Exercises | 890 | ||
Programming Projects | 892 | ||
Chapter 19 Standard Template Library | 895 | ||
19.1 ITERATORS | 897 | ||
Iterator Basics | 897 | ||
PITFALL: Compiler Problems | 902 | ||
TIP: Use auto to Simplify Variable Declarations | 903 | ||
Kinds of Iterators | 903 | ||
Constant and Mutable Iterators | 906 | ||
Reverse Iterators | 908 | ||
Other Kinds of Iterators | 909 | ||
19.2 CONTAINERS | 910 | ||
Sequential Containers | 910 | ||
PITFALL: Iterators and Removing Elements | 915 | ||
TIP: Type Definitions in Containers | 916 | ||
The Container Adapters stack and queue | 916 | ||
PITFALL: Underlying Containers | 917 | ||
The Associative Containers set and map | 920 | ||
Efficiency | 925 | ||
TIP: Use Initialization, Ranged for, and auto with Containers | 927 | ||
19.3 GENERIC ALGORITHMS | 928 | ||
Running Times and Big-O Notation | 928 | ||
Container Access Running Times | 932 | ||
Nonmodifying Sequence Algorithms | 933 | ||
Modifying Sequence Algorithms | 937 | ||
Set Algorithms | 939 | ||
Sorting Algorithms | 940 | ||
Chapter Summary | 941 | ||
Answers to Self-Test Exercises | 941 | ||
Appendix 1 C++ Keywords | AP-1 | ||
Appendix 2 Precedence of Operators | AP-3 | ||
Appendix 3 The ASCII Character Set | AP-5 | ||
Appendix 4 Some Library Functions | AP-7 | ||
Appendix 5 Old and New Header Files | AP-15 | ||
Appendix 6 Additional C++11 Language Features | AP-17 | ||
Index | I-1 | ||
A | I-1 | ||
B | I-3 | ||
C | I-3 | ||
D | I-6 | ||
E | I-7 | ||
F | I-7 | ||
G | I-9 | ||
H | I-9 | ||
I | I-9 | ||
K | I-11 | ||
L | I-11 | ||
M | I-12 | ||
N | I-13 | ||
O | I-14 | ||
P | I-15 | ||
Q | I-16 | ||
R | I-16 | ||
S | I-17 | ||
T | I-19 | ||
U | I-20 | ||
V | I-20 | ||
W | I-21 | ||
Z | I-21 |