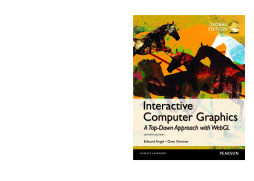
Additional Information
Book Details
Abstract
This book is suitable for undergraduate students in computer science and engineering, for students in other disciplines who have good programming skills, and for professionals.
Computer animation and graphics are now prevalent in everyday life from the computer screen, to the movie screen, to the smart phone screen. The growing excitement about WebGL applications and their ability to integrate HTML5, inspired the authors to exclusively use WebGL in the Seventh Edition of Interactive Computer Graphics with WebGL. Thisis the only introduction to computer graphics text for undergraduates that fully integrates WebGL and emphasizes application-based programming. The top-down, programming-oriented approach allows for coverage of engaging 3D material early in the course so students immediately begin to create their own 3D graphics.
Teaching and Learning Experience
This program will provide a better teaching and learning experience–for you and your students. It will help:
- Engage Students Immediately with 3D Material: A top-down, programming-oriented approach allows for coverage of engaging 3D material early in the course so students immediately begin to create their own graphics.
- Introduce Computer Graphics Programming with WebGL and JavaScript: WebGL is not only fully shader-based–each application must provide at least a vertex shader and a fragment shader–but also a version that works within the latest web browsers.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Dedication | 5 | ||
Contents\r | 7 | ||
Preface | 21 | ||
Chapter 1: Graphics Systems and Models | 31 | ||
1.1 Applications of Computer Graphics | 32 | ||
1.1.1 Display of Information | 32 | ||
1.1.2 Design | 33 | ||
1.1.3 Simulation and Animation | 33 | ||
1.1.4 User Interfaces | 34 | ||
1.2 A Graphics System | 35 | ||
1.2.1 Pixels and the Framebuffer | 35 | ||
1.2.2 The CPU and the GPU | 36 | ||
1.2.3 Output Devices | 37 | ||
1.2.4 Input Devices | 39 | ||
1.3 Images: Physical and Synthetic | 40 | ||
1.3.1 Objects and Viewers | 40 | ||
1.3.2 Light and Images | 42 | ||
1.3.3 Imaging Models | 43 | ||
1.4 Imaging Systems | 45 | ||
1.4.1 The Pinhole Camera | 45 | ||
1.4.2 The Human Visual System | 47 | ||
1.5 The Synthetic-Camera Model | 48 | ||
1.6 The Programmer’s Interface | 50 | ||
1.6.1 The Pen-Plotter Model | 51 | ||
1.6.2 Three-Dimensional APIs | 53 | ||
1.6.3 A Sequence of Images | 56 | ||
1.6.4 The Modeling–Rendering Paradigm | 57 | ||
1.7 Graphics Architectures | 58 | ||
1.7.1 Display Processors | 59 | ||
1.7.2 Pipeline Architectures | 59 | ||
1.7.3 The Graphics Pipeline | 60 | ||
1.7.4 Vertex Processing | 61 | ||
1.7.5 Clipping and Primitive Assembly | 61 | ||
1.7.6 Rasterization | 62 | ||
1.7.7 Fragment Processing | 62 | ||
1.8 Programmable Pipelines | 62 | ||
1.9 Performance Characteristics | 63 | ||
1.10 OpenGL Versions and WebGL | 64 | ||
Summary and Notes | 66 | ||
Suggested Readings | 66 | ||
Exercises | 67 | ||
Chapter 2: Graphics Programming | 69 | ||
2.1 The Sierpinski Gasket | 69 | ||
2.2 Programming Two-Dimensional Applications | 72 | ||
2.3 The WebGL Application Programming Interface | 77 | ||
2.3.1 Graphics Functions | 77 | ||
2.3.2 The Graphics Pipeline and State Machines | 79 | ||
2.3.3 OpenGL and WebGL | 80 | ||
2.3.4 The WebGL Interface | 80 | ||
2.3.5 Coordinate Systems | 81 | ||
2.4 Primitives and Attributes | 83 | ||
2.4.1 Polygon Basics | 85 | ||
2.4.2 Polygons in WebGL | 86 | ||
2.4.3 Approximating a Sphere | 87 | ||
2.4.4 Triangulation | 88 | ||
2.4.5 Text | 89 | ||
2.4.6 Curved Objects | 90 | ||
2.4.7 Attributes | 91 | ||
2.5 Color | 92 | ||
2.5.1 RGB Color | 94 | ||
2.5.2 Indexed Color | 96 | ||
2.5.3 Setting of Color Attributes | 97 | ||
2.6 Viewing | 98 | ||
2.6.1 The Orthographic View | 98 | ||
2.6.2 Two-Dimensional Viewing | 101 | ||
2.7 Control Functions | 101 | ||
2.7.1 Interaction with the Window System | 102 | ||
2.7.2 Aspect Ratio and Viewports | 103 | ||
2.7.3 Application Organization | 104 | ||
2.8 The Gasket Program | 105 | ||
2.8.1 Sending Data to the GPU | 108 | ||
2.8.2 Rendering the Points | 108 | ||
2.8.3 The Vertex Shader | 109 | ||
2.8.4 The Fragment Shader | 110 | ||
2.8.5 Combining the Parts | 110 | ||
2.8.6 The initShaders Function | 111 | ||
2.8.7 The init Function | 112 | ||
2.8.8 Reading the Shaders from the Application | 113 | ||
2.9 Polygons and Recursion | 113 | ||
2.10 The Three-Dimensional Gasket | 116 | ||
2.10.1 Use of Three-Dimensional Points | 116 | ||
2.10.2 Naming Conventions | 118 | ||
2.10.3 Use of Polygons in Three Dimensions | 118 | ||
2.10.4 Hidden-Surface Removal | 121 | ||
Summary and Notes | 123 | ||
Suggested Readings | 124 | ||
Exercises | 125 | ||
Chapter 3: Interaction and Animation | 129 | ||
3.1 Animation | 129 | ||
3.1.1 The Rotating Square | 130 | ||
3.1.2 The Display Process | 132 | ||
3.1.3 Double Buffering | 133 | ||
3.1.4 Using a Timer | 134 | ||
3.1.5 Using requestAnimFrame | 135 | ||
3.2 Interaction | 136 | ||
3.3 Input Devices | 137 | ||
3.4 Physical Input Devices | 138 | ||
3.4.1 Keyboard Codes | 138 | ||
3.4.2 The Light Pen | 139 | ||
3.4.3 The Mouse and the Trackball | 139 | ||
3.4.4 Data Tablets,Touch Pads, and Touch Screens | 140 | ||
3.4.5 The Joystick | 141 | ||
3.4.6 Multidimensional Input Devices | 141 | ||
3.4.7 Logical Devices | 142 | ||
3.4.8 Input Modes | 143 | ||
3.5 Clients and Servers | 145 | ||
3.6 Programming Event-Driven Input | 146 | ||
3.6.1 Events and Event Listeners | 147 | ||
3.6.2 Adding a Button | 147 | ||
3.6.3 Menus | 149 | ||
3.6.4 Using Keycodes | 150 | ||
3.6.5 Sliders | 151 | ||
3.7 Position Input | 152 | ||
3.8 Window Events | 153 | ||
3.9 Picking | 155 | ||
3.10 Building Models Interactively | 156 | ||
3.11 Design of Interactive Programs | 160 | ||
Summary and Notes | 160 | ||
Suggested Readings | 161 | ||
Exercises | 162 | ||
Chapter 4: Geometric Objects and Transformations | 165 | ||
4.1 Scalars, Points, and Vectors | 166 | ||
4.1.1 Geometric Objects | 166 | ||
4.1.2 Coordinate-Free Geometry | 168 | ||
4.1.3 The Mathematical View: Vector and Affine Spaces | 168 | ||
4.1.4 The Computer Science View | 169 | ||
4.1.5 Geometric ADTs | 170 | ||
4.1.6 Lines | 171 | ||
4.1.7 Affine Sums | 171 | ||
4.1.8 Convexity | 172 | ||
4.1.9 Dot and Cross Products | 172 | ||
4.1.10 Planes | 173 | ||
4.2 Three-Dimensional Primitives | 175 | ||
4.3 Coordinate Systems and Frames | 176 | ||
4.3.1 Representations and N-Tuples | 178 | ||
4.3.2 Change of Coordinate Systems | 179 | ||
4.3.3 Example: Change of Representation | 181 | ||
4.3.4 Homogeneous Coordinates | 183 | ||
4.3.5 Example: Change in Frames | 185 | ||
4.3.6 Working with Representations | 187 | ||
4.4 Frames in WebGL | 189 | ||
4.5 Matrix and Vector Types | 193 | ||
4.5.1 Row versus Column Major Matrix Representations | 195 | ||
4.6 Modeling a Colored Cube | 195 | ||
4.6.1 Modeling the Faces | 196 | ||
4.6.2 Inward- and Outward-Pointing Faces | 197 | ||
4.6.3 Data Structures for Object Representation | 197 | ||
4.6.4 The Colored Cube | 198 | ||
4.6.5 Color Interpolation | 200 | ||
4.6.6 Displaying the Cube | 200 | ||
4.6.7 Drawing with Elements | 201 | ||
4.7 Affine Transformations | 202 | ||
4.8 Translation, Rotation, and Scaling | 205 | ||
4.8.1 Translation | 205 | ||
4.8.2 Rotation | 206 | ||
4.8.3 Scaling | 207 | ||
4.9 Transformations in Homogeneous Coordinates | 209 | ||
4.9.1 Translation | 209 | ||
4.9.2 Scaling | 211 | ||
4.9.3 Rotation | 211 | ||
4.9.4 Shear | 213 | ||
4.10 Concatenation of Transformations | 214 | ||
4.10.1 Rotation About a Fixed Point | 215 | ||
4.10.2 General Rotation | 216 | ||
4.10.3 The Instance Transformation | 217 | ||
4.10.4 Rotation About an Arbitrary Axis | 218 | ||
4.11 Transformation Matrices in WebGL | 221 | ||
4.11.1 Current Transformation Matrices | 222 | ||
4.11.2 Basic Matrix Functions | 223 | ||
4.11.3 Rotation, Translation, and Scaling | 224 | ||
4.11.4 Rotation About a Fixed Point | 225 | ||
4.11.5 Order of Transformations | 225 | ||
4.12 Spinning of the Cube | 226 | ||
4.12.1 Uniform Matrices | 228 | ||
4.13 Interfaces to Three-Dimensional Applications | 230 | ||
4.13.1 Using Areas of the Screen | 231 | ||
4.13.2 A Virtual Trackball | 231 | ||
4.13.3 Smooth Rotations | 234 | ||
4.13.4 Incremental Rotation | 235 | ||
4.14 Quaternions | 236 | ||
4.14.1 Complex Numbers and Quaternions | 236 | ||
4.14.2 Quaternions and Rotation | 237 | ||
4.14.3 Quaternions and Gimbal Lock | 239 | ||
Summary and Notes | 240 | ||
Suggested Readings | 241 | ||
Exercises | 241 | ||
Chapter 5: Viewing | 245 | ||
5.1 Classical and Computer Viewing | 245 | ||
5.1.1 Classical Viewing | 247 | ||
5.1.2 Orthographic Projections | 247 | ||
5.1.3 Axonometric Projections | 248 | ||
5.1.4 Oblique Projections | 250 | ||
5.1.5 Perspective Viewing | 251 | ||
5.2 Viewing with a Computer | 252 | ||
5.3 Positioning of the Camera | 254 | ||
5.3.1 Positioning of the Camera Frame | 254 | ||
5.3.2 Two Viewing APIs | 259 | ||
5.3.3 The Look-At Function | 262 | ||
5.3.4 Other Viewing APIs | 263 | ||
5.4 Parallel Projections | 264 | ||
5.4.1 Orthogonal Projections | 264 | ||
5.4.2 Parallel Viewing with WebGL | 265 | ||
5.4.3 Projection Normalization | 266 | ||
5.4.4 Orthogonal Projection Matrices | 267 | ||
5.4.5 Oblique Projections | 269 | ||
5.4.6 An Interactive Viewer | 272 | ||
5.5 Perspective Projections | 274 | ||
5.5.1 Simple Perspective Projections | 275 | ||
5.6 Perspective Projections with WebGL | 278 | ||
5.6.1 Perspective Functions | 279 | ||
5.7 Perspective Projection Matrices | 280 | ||
5.7.1 Perspective Normalization | 280 | ||
5.7.2 WebGL Perspective Transformations | 284 | ||
5.7.3 Perspective Example | 286 | ||
5.8 Hidden-Surface Removal | 286 | ||
5.8.1 Culling | 288 | ||
5.9 Displaying Meshes | 289 | ||
5.9.1 Displaying Meshes as Surfaces | 292 | ||
5.9.2 Polygon Offset | 294 | ||
5.9.3 Walking through a Scene | 295 | ||
5.10 Projections and Shadows | 295 | ||
5.10.1 Projected Shadows | 296 | ||
5.11 Shadow Maps | 300 | ||
Summary and Notes | 301 | ||
Suggested Readings | 302 | ||
Exercises | 302 | ||
Chapter 6: Lighting and Shading | 305 | ||
6.1 Light and Matter | 306 | ||
6.2 Light Sources | 309 | ||
6.2.1 Color Sources | 310 | ||
6.2.2 Ambient Light | 310 | ||
6.2.3 Point Sources | 311 | ||
6.2.4 Spotlights | 312 | ||
6.2.5 Distant Light Sources | 312 | ||
6.3 The Phong Reflection Model | 313 | ||
6.3.1 Ambient Reflection | 315 | ||
6.3.2 Diffuse Reflection | 315 | ||
6.3.3 Specular Reflection | 316 | ||
6.3.4 The Modified Phong Model | 318 | ||
6.4 Computation of Vectors | 319 | ||
6.4.1 Normal Vectors | 319 | ||
6.4.2 Angle of Reflection | 322 | ||
6.5 Polygonal Shading | 323 | ||
6.5.1 Flat Shading | 323 | ||
6.5.2 Smooth and Gouraud Shading | 324 | ||
6.5.3 Phong Shading | 326 | ||
6.6 Approximation of a Sphere by Recursive Subdivision | 327 | ||
6.7 Specifying Lighting Parameters | 329 | ||
6.7.1 Light Sources | 329 | ||
6.7.2 Materials | 331 | ||
6.8 Implementing a Lighting Model | 331 | ||
6.8.1 Applying the Lighting Model in the Application | 332 | ||
6.8.2 Efficiency | 334 | ||
6.8.3 Lighting in the Vertex Shader | 335 | ||
6.9 Shading of the Sphere Model | 340 | ||
6.10 Per-Fragment Lighting | 341 | ||
6.11 Nonphotorealistic Shading | 343 | ||
6.12 Global Illumination | 344 | ||
Summary and Notes | 345 | ||
Suggested Readings | 346 | ||
Exercises | 346 | ||
Chapter 7: Discrete Techniques | 349 | ||
7.1 Buffers | 350 | ||
7.2 Digital Images | 351 | ||
7.3 Mapping Methods | 355 | ||
7.4 Two-Dimensional Texture Mapping | 357 | ||
7.5 Texture Mapping in WebGL | 363 | ||
7.5.1 Texture Objects | 364 | ||
7.5.2 The Texture Image Array | 365 | ||
7.5.3 Texture Coordinates and Samplers | 366 | ||
7.5.4 Texture Sampling | 371 | ||
7.5.5 Working with Texture Coordinates | 374 | ||
7.5.6 Multitexturing | 375 | ||
7.6 Texture Generation | 378 | ||
7.7 Environment Maps | 379 | ||
7.8 Reflection Map Example | 383 | ||
7.9 Bump Mapping | 387 | ||
7.9.1 Finding Bump Maps | 388 | ||
7.9.2 Bump Map Example | 391 | ||
7.10 Blending Techniques | 395 | ||
7.10.1 Opacity and Blending | 396 | ||
7.10.2 Image Blending | 397 | ||
7.10.3 Blending in WebGL | 397 | ||
7.10.4 Antialiasing Revisited | 399 | ||
7.10.5 Back-to-Front and Front-to-Back Rendering | 401 | ||
7.10.6 Scene Antialiasing and Multisampling | 401 | ||
7.10.7 Image Processing | 402 | ||
7.10.8 Other Multipass Methods | 404 | ||
7.11 GPGPU | 404 | ||
7.12 Framebuffer Objects | 408 | ||
7.13 Buffer Ping-Ponging | 414 | ||
7.14 Picking | 417 | ||
Summary and Notes | 422 | ||
Suggested Readings | 423 | ||
Exercises | 424 | ||
Chapter 8: From Geometry To Pixels | 427 | ||
8.1 Basic Implementation Strategies | 428 | ||
8.2 Four Major Tasks | 430 | ||
8.2.1 Modeling | 430 | ||
8.2.2 Geometry Processing | 431 | ||
8.2.3 Rasterization | 432 | ||
8.2.4 Fragment Processing | 433 | ||
8.3 Clipping | 433 | ||
8.4 Line-Segment Clipping | 434 | ||
8.4.1 Cohen-Sutherland Clipping | 434 | ||
8.4.2 Liang-Barsky Clipping | 437 | ||
8.5 Polygon Clipping | 438 | ||
8.6 Clipping of Other Primitives | 440 | ||
8.6.1 Bounding Boxes and Volumes | 440 | ||
8.6.2 Curves, Surfaces, and Text | 442 | ||
8.6.3 Clipping in the Framebuffer | 443 | ||
8.7 Clipping in Three Dimensions | 443 | ||
8.8 Rasterization | 446 | ||
8.9 Bresenham’s Algorithm | 448 | ||
8.10 Polygon Rasterization | 450 | ||
8.10.1 Inside–Outside Testing | 451 | ||
8.10.2 WebGL and Concave Polygons | 452 | ||
8.10.3 Fill and Sort | 453 | ||
8.10.4 Flood Fill | 453 | ||
8.10.5 Singularities | 454 | ||
8.11 Hidden-Surface Removal | 454 | ||
8.11.1 Object-Space and Image-Space Approaches | 454 | ||
8.11.2 Sorting and Hidden-Surface Removal | 456 | ||
8.11.3 Scan Line Algorithms | 456 | ||
8.11.4 Back-Face Removal | 457 | ||
8.11.5 The z-Buffer Algorithm | 459 | ||
8.11.6 Scan Conversion with the z-Buffer | 461 | ||
8.11.7 Depth Sort and the Painter’s Algorithm | 462 | ||
8.12 Antialiasing | 465 | ||
8.13 Display Considerations | 467 | ||
8.13.1 Color Systems | 467 | ||
8.13.2 The Color Matrix | 471 | ||
8.13.3 Gamma Correction | 471 | ||
8.13.4 Dithering and Halftoning | 472 | ||
Summary and Notes | 473 | ||
Suggested Readings | 475 | ||
Exercises | 475 | ||
Chapter 9: Modeling and Hierarchy | 479 | ||
9.1 Symbols and Instances | 480 | ||
9.2 Hierarchical Models | 481 | ||
9.3 A Robot Arm | 483 | ||
9.4 Trees and Traversal | 486 | ||
9.4.1 A Stack-Based Traversal | 487 | ||
9.5 Use of Tree Data Structures | 490 | ||
9.6 Animation | 494 | ||
9.7 Graphical Objects | 495 | ||
9.7.1 Methods, Attributes, and Messages | 496 | ||
9.7.2 A Cube Object | 497 | ||
9.7.3 Objects and Hierarchy | 498 | ||
9.7.4 Geometric and Nongeometric Objects | 499 | ||
9.8 Scene Graphs | 500 | ||
9.9 Implementing Scene Graphs | 502 | ||
9.10 Other Tree Structures | 504 | ||
9.10.1 CSG Trees | 504 | ||
9.10.2 BSP Trees | 505 | ||
9.10.3 Quadtrees and Octrees | 508 | ||
Summary and Notes | 509 | ||
Suggested Readings | 510 | ||
Exercises | 510 | ||
Chapter 10: Procedural Methods | 513 | ||
10.1 Algorithmic Models | 513 | ||
10.2 Physically Based Models and Particle Systems | 515 | ||
10.3 Newtonian Particles | 516 | ||
10.3.1 Independent Particles | 518 | ||
10.3.2 Spring Forces | 518 | ||
10.3.3 Attractive and Repulsive Forces | 520 | ||
10.4 Solving Particle Systems | 521 | ||
10.5 Constraints | 524 | ||
10.5.1 Collisions | 524 | ||
10.5.2 Soft Constraints | 526 | ||
10.6 A Simple Particle System | 527 | ||
10.6.1 Displaying the Particles | 528 | ||
10.6.2 Updating Particle Positions | 528 | ||
10.6.3 Collisions | 529 | ||
10.6.4 Forces | 530 | ||
10.6.5 Flocking | 530 | ||
10.7 Agent-Based Models | 531 | ||
10.8 Language-Based Models | 533 | ||
10.9 Recursive Methods and Fractals | 537 | ||
10.9.1 Rulers and Length | 538 | ||
10.9.2 Fractal Dimension | 539 | ||
10.9.3 Midpoint Division and Brownian Motion | 540 | ||
10.9.4 Fractal Mountains | 541 | ||
10.9.5 The Mandelbrot Set | 542 | ||
10.9.6 Mandelbrot Fragment Shader | 546 | ||
10.10 Procedural Noise | 547 | ||
Summary and Notes | 551 | ||
Suggested Readings | 551 | ||
Exercises | 552 | ||
Chapter 11: Curves and Surfaces | 555 | ||
11.1 Representation of Curves and Surfaces | 555 | ||
11.1.1 Explicit Representation | 555 | ||
11.1.2 Implicit Representations | 557 | ||
11.1.3 Parametric Form | 558 | ||
11.1.4 Parametric Polynomial Curves | 559 | ||
11.1.5 Parametric Polynomial Surfaces | 560 | ||
11.2 Design Criteria | 560 | ||
11.3 Parametric Cubic Polynomial Curves | 562 | ||
11.4 Interpolation | 563 | ||
11.4.1 Blending Functions | 564 | ||
11.4.2 The Cubic Interpolating Patch | 566 | ||
11.5 Hermite Curves and Surfaces | 568 | ||
11.5.1 The Hermite Form | 568 | ||
11.5.2 Geometric and Parametric Continuity | 570 | ||
11.6 Be´zier Curves and Surfaces | 571 | ||
11.6.1 Be´zier Curves | 572 | ||
11.6.2 Be´zier Surface Patches | 574 | ||
11.7 Cubic B-Splines | 575 | ||
11.7.1 The Cubic B-Spline Curve | 575 | ||
11.7.2 B-Splines and Basis | 578 | ||
11.7.3 Spline Surfaces | 579 | ||
11.8 General B-Splines | 580 | ||
11.8.1 Recursively Defined B-Splines | 581 | ||
11.8.2 Uniform Splines | 582 | ||
11.8.3 Nonuniform B-Splines | 582 | ||
11.8.4 NURBS | 583 | ||
11.8.5 Catmull-Rom Splines | 584 | ||
11.9 Rendering Curves and Surfaces | 585 | ||
11.9.1 Polynomial Evaluation Methods | 586 | ||
11.9.2 Recursive Subdivision of Be´zier Polynomials | 587 | ||
11.9.3 Rendering Other Polynomial Curves by Subdivision | 590 | ||
11.9.4 Subdivision of Be´zier Surfaces | 591 | ||
11.10 The Utah Teapot | 592 | ||
11.11 Algebraic Surfaces | 595 | ||
11.11.1 Quadrics | 595 | ||
11.11.2 Rendering of Surfaces by Ray Casting | 596 | ||
11.12 Subdivision Curves and Surfaces | 597 | ||
11.12.1 Mesh Subdivision | 598 | ||
11.13 Mesh Generation from Data | 601 | ||
11.13.1 Height Fields Revisited | 601 | ||
11.13.2 Delaunay Triangulation | 601 | ||
11.13.3 Point Clouds | 605 | ||
11.14 Graphics API support for Curves and Surfaces | 606 | ||
11.14.1 Tessellation Shading | 606 | ||
11.14.2 Geometry Shading | 607 | ||
Summary and Notes | 607 | ||
Suggested Readings | 608 | ||
Exercises | 608 | ||
Chapter 12: Advanced Rendering | 611 | ||
12.1 Going Beyond Pipeline Rendering | 611 | ||
12.2 Ray Tracing | 612 | ||
12.3 Building a Simple Ray Tracer | 616 | ||
12.3.1 Recursive Ray Tracing | 616 | ||
12.3.2 Calculating Intersections | 618 | ||
12.3.3 Ray-Tracing Variations | 620 | ||
12.4 The Rendering Equation | 621 | ||
12.5 Radiosity | 623 | ||
12.5.1 The Radiosity Equation | 624 | ||
12.5.2 Solving the Radiosity Equation | 625 | ||
12.5.3 Computing Form Factors | 627 | ||
12.5.4 Carrying Out Radiosity | 629 | ||
12.6 Global Illumination and Path Tracing | 630 | ||
12.7 RenderMan | 632 | ||
12.8 Parallel Rendering | 633 | ||
12.8.1 Sort-Middle Rendering | 635 | ||
12.8.2 Sort-Last Rendering | 636 | ||
12.8.3 Sort-First Rendering | 640 | ||
12.9 Hardware GPU Implementations | 641 | ||
12.10 Implicit Functions and Contour Maps | 642 | ||
12.10.1 Marching Squares | 643 | ||
12.10.2 Marching Triangles | 647 | ||
12.11 Volume Rendering | 648 | ||
12.11.1 Volumetric Data Sets | 648 | ||
12.11.2 Visualization of Implicit Functions | 649 | ||
12.12 Isosurfaces and Marching Cubes | 651 | ||
12.13 Marching Tetrahedra | 654 | ||
12.14 Mesh Simplification | 655 | ||
12.15 Direct Volume Rendering | 655 | ||
12.15.1 Assignment of Color and Opacity | 656 | ||
12.15.2 Splatting | 657 | ||
12.15.3 Volume Ray Tracing | 658 | ||
12.15.4 Texture Mapping of Volumes | 659 | ||
12.16 Image-Based Rendering | 660 | ||
12.16.1 A Simple Example | 660 | ||
Summary and Notes | 662 | ||
Suggested Readings | 663 | ||
Exercises | 664 | ||
Appendix | 667 | ||
Appendix A: Initializing Shaders | 667 | ||
A.1 Shaders in the HTML file | 667 | ||
A.2 Reading Shaders from Source Files | 670 | ||
Appendix B: Spaces | 673 | ||
B.1 Scalars | 673 | ||
B.2 Vector Spaces | 674 | ||
B.3 Affine Spaces | 676 | ||
B.4 Euclidean Spaces | 677 | ||
B.5 Projections | 678 | ||
B.6 Gram-Schmidt Orthogonalization | 679 | ||
Suggested Readings | 680 | ||
Exercises | 680 | ||
Appendix C: Matrices | 681 | ||
C.1 Definitions | 681 | ||
C.2 Matrix Operations | 682 | ||
C.3 Row and Column Matrices | 683 | ||
C.4 Rank | 684 | ||
C.5 Change of Representation | 685 | ||
C.6 The Cross Product | 687 | ||
C.7 Eigenvalues and Eigenvectors | 687 | ||
C.8 Vector and Matrix Objects | 689 | ||
Suggested Readings | 689 | ||
Exercises | 690 | ||
Appendix D: Sampling and Aliasing | 691 | ||
D.1 Sampling Theory | 691 | ||
D.2 Reconstruction | 696 | ||
D.3 Quantization | 698 | ||
References | 699 | ||
WebGL Index | 711 | ||
Subject Index | 713 |