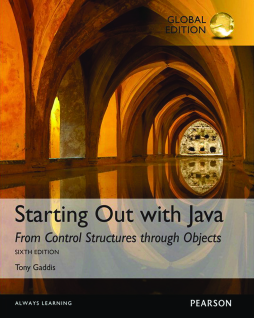
BOOK
Starting Out with Java: From Control Structures through Objects, Global Edition
(2016)
Additional Information
Book Details
Abstract
For courses in computer programming in Java.
Starting Out with Java: From Control Structures through Objects provides a step-by-step introduction to programming in Java. Gaddis covers procedural programming—control structures and methods—before introducing object-oriented programming, ensuring that students understand fundamental programming and problem-solving concepts.
As with all Gaddis texts, every chapter contains clear and easy-to-read code listings, concise and practical real-world examples, and an abundance of exercises.
MyProgrammingLab® not included. Students, if MyProgrammingLab is a recommended/mandatory component of the course, please ask your instructor for the correct ISBN and course ID. MyProgrammingLab should only be purchased when required by an instructor. Instructors, contact your Pearson representative for more information.
MyProgrammingLab is an online homework, tutorial, and assessment product designed to personalize learning and improve results. With a wide range of interactive, engaging, and assignable activities, students are encouraged to actively learn and retain tough course concepts.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Title Page | 3 | ||
Copyright Page | 4 | ||
Acknowledgments | 30 | ||
About the Author | 32 | ||
Contents | 7 | ||
Preface | 23 | ||
Chapter 1 Introduction to Computers and Java | 37 | ||
1.1 Introduction | 37 | ||
1.2 Why Program? | 37 | ||
1.3 Computer Systems: Hardware and Software | 38 | ||
Hardware | 38 | ||
Software | 41 | ||
1.4 Programming Languages | 42 | ||
What Is a Program? | 42 | ||
A History of Java | 44 | ||
Java Applications and Applets | 44 | ||
1.5 What Is a Program Made Of? | 45 | ||
Language Elements | 45 | ||
Lines and Statements | 47 | ||
Variables | 47 | ||
The Compiler and the Java Virtual Machine | 48 | ||
Java Software Editions | 50 | ||
Compiling and Running a Java Program | 50 | ||
1.6 The Programming Process | 52 | ||
Software Engineering | 54 | ||
1.7 Object-Oriented Programming | 55 | ||
Review Questions and Exercises | 57 | ||
Programming Challenge | 61 | ||
Chapter 2 Java Fundamentals | 63 | ||
2.1 The Parts of a Java Program | 63 | ||
2.2 The print and println Methods, and the Java API | 69 | ||
2.3 Variables and Literals | 75 | ||
Displaying Multiple Items with the + Operator | 76 | ||
Be Careful with Quotation Marks | 77 | ||
More about Literals | 78 | ||
Identifiers | 78 | ||
Class Names | 80 | ||
2.4 Primitive Data Types | 80 | ||
The Integer Data Types | 82 | ||
Floating-Point Data Types | 83 | ||
The boolean Data Type | 86 | ||
The char Data Type | 86 | ||
Variable Assignment and Initialization | 88 | ||
Variables Hold Only One Value at a Time | 89 | ||
2.5 Arithmetic Operators | 90 | ||
Integer Division | 93 | ||
Operator Precedence | 93 | ||
Grouping with Parentheses | 95 | ||
The Math Class | 98 | ||
2.6 Combined Assignment Operators | 99 | ||
2.7 Conversion between Primitive Data Types | 101 | ||
Mixed Integer Operations | 103 | ||
Other Mixed Mathematical Expressions | 104 | ||
2.8 Creating Named Constants with final | 105 | ||
2.9 The String Class | 106 | ||
Objects Are Created from Classes | 106 | ||
The String Class | 107 | ||
Primitive Type Variables and Class Type Variables | 107 | ||
Creating a String Object | 108 | ||
2.10 Scope | 111 | ||
2.11 Comments | 113 | ||
2.12 Programming Style | 118 | ||
2.13 Reading Keyboard Input | 120 | ||
Reading a Character | 124 | ||
Mixing Calls to nextLine with Calls to Other Scanner Methods | 124 | ||
2.14 Dialog Boxes | 128 | ||
Displaying Message Dialogs | 128 | ||
Displaying Input Dialogs | 129 | ||
An Example Program | 129 | ||
Converting String Input to Numbers | 131 | ||
2.15 Common Errors to Avoid | 135 | ||
Review Questions and Exercises | 136 | ||
Programming Challenges | 141 | ||
Chapter 3 Decision Structures | 147 | ||
3.1 The if Statement | 147 | ||
Using Relational Operators to Form Conditions | 149 | ||
Putting It All Together | 150 | ||
Programming Style and the if Statement | 154 | ||
Be Careful with Semicolons | 155 | ||
Having Multiple Conditionally Executed Statements | 155 | ||
Flags | 156 | ||
Comparing Characters | 156 | ||
3.2 The if-else Statement | 157 | ||
3.3 Nested if Statements | 160 | ||
3.4 The if-else-if Statement | 167 | ||
3.5 Logical Operators | 173 | ||
The Precedence of Logical Operators | 179 | ||
Checking Numeric Ranges with Logical Operators | 180 | ||
3.6 Comparing String Objects | 181 | ||
Ignoring Case in String Comparisons | 186 | ||
3.7 More about Variable Declaration and Scope | 187 | ||
3.8 The Conditional Operator (Optional) | 188 | ||
3.9 The switch Statement | 190 | ||
3.10 Displaying Formatted Output with System.out.printf and String.format | 200 | ||
Format Specifier Syntax | 203 | ||
Precision | 203 | ||
Specifying a Minimum Field Width | 204 | ||
Flags | 206 | ||
Formatting String Arguments | 210 | ||
The String.format Method | 211 | ||
3.11 Common Errors to Avoid | 214 | ||
Review Questions and Exercises | 215 | ||
Programming Challenges | 220 | ||
Chapter 4 Loops and Files | 225 | ||
4.1 The Increment and Decrement Operators | 225 | ||
The Difference between Postfix and Prefix Modes | 228 | ||
4.2 The while Loop | 229 | ||
The while Loop Is a Pretest Loop | 232 | ||
Infinite Loops | 232 | ||
Don’t Forget the Braces with a Block of Statements | 233 | ||
Programming Style and the while Loop | 234 | ||
4.3 Using the while Loop for Input Validation | 236 | ||
4.4 The do-while Loop | 240 | ||
4.5 The for Loop | 243 | ||
The for Loop Is a Pretest Loop | 246 | ||
Avoid Modifying the Control Variable in the Body of the for Loop | 247 | ||
Other Forms of the Update Expression | 247 | ||
Declaring a Variable in the for Loop’s Initialization Expression | 247 | ||
Creating a User Controlled for Loop | 248 | ||
Using Multiple Statements in the Initialization and Update Expressions | 249 | ||
4.6 Running Totals and Sentinel Values | 252 | ||
Using a Sentinel Value | 255 | ||
4.7 Nested Loops | 257 | ||
4.8 The break and continue Statements (Optional) | 265 | ||
4.9 Deciding Which Loop to Use | 265 | ||
4.10 Introduction to File Input and Output | 266 | ||
Using the PrintWriter Class to Write Data to a File | 266 | ||
Appending Data to a File | 272 | ||
Specifying the File Location | 273 | ||
Reading Data from a File | 273 | ||
Reading Lines from a File with the nextLine Method | 274 | ||
Adding a throws Clause to the Method Header | 277 | ||
Checking for a File’s Existence | 281 | ||
4.11 Generating Random Numbers with the Random Class | 285 | ||
4.12 Common Errors to Avoid | 291 | ||
Review Questions and Exercises | 292 | ||
Programming Challenges | 298 | ||
Chapter 5 Methods | 305 | ||
5.1 Introduction to Methods | 305 | ||
void Methods and Value-Returning Methods | 306 | ||
Defining a void Method | 307 | ||
Calling a Method | 308 | ||
Hierarchical Method Calls | 313 | ||
Using Documentation Comments with Methods | 314 | ||
5.2 Passing Arguments to a Method | 315 | ||
Argument and Parameter Data Type Compatibility | 317 | ||
Parameter Variable Scope | 318 | ||
Passing Multiple Arguments | 318 | ||
Arguments Are Passed by Value | 320 | ||
Passing Object References to a Method | 321 | ||
Using the @param Tag in Documentation Comments | 324 | ||
5.3 More about Local Variables | 327 | ||
Local Variable Lifetime | 328 | ||
Initializing Local Variables with Parameter Values | 328 | ||
5.4 Returning a Value from a Method | 329 | ||
Defining a Value-Returning Method | 329 | ||
Calling a Value-Returning Method | 331 | ||
Using the @return Tag in Documentation Comments | 332 | ||
Returning a boolean Value | 336 | ||
Returning a Reference to an Object | 336 | ||
5.5 Problem Solving with Methods | 338 | ||
Calling Methods That Throw Exceptions | 342 | ||
5.6 Common Errors to Avoid | 342 | ||
Review Questions and Exercises | 343 | ||
Programming Challenges | 348 | ||
Chapter 6 A First Look at Classes | 355 | ||
6.1 Objects and Classes | 355 | ||
Classes: Where Objects Come From | 356 | ||
Classes in the Java API | 357 | ||
Primitive Variables vs. Objects | 359 | ||
6.2 Writing a Simple Class, Step by Step | 362 | ||
Accessor and Mutator Methods | 376 | ||
The Importance of Data Hiding | 376 | ||
Avoiding Stale Data | 377 | ||
Showing Access Specification in UML Diagrams | 377 | ||
Data Type and Parameter Notation in UML Diagrams | 377 | ||
Layout of Class Members | 378 | ||
6.3 Instance Fields and Methods | 379 | ||
6.4 Constructors | 384 | ||
Showing Constructors in a UML Diagram | 386 | ||
Uninitialized Local Reference Variables | 386 | ||
The Default Constructor | 386 | ||
Writing Your Own No-Arg Constructor | 387 | ||
The String Class Constructor | 388 | ||
6.5 Passing Objects as Arguments | 396 | ||
6.6 Overloading Methods and Constructors | 408 | ||
The BankAccount Class | 410 | ||
Overloaded Methods Make Classes More Useful | 416 | ||
6.7 Scope of Instance Fields | 416 | ||
Shadowing | 417 | ||
6.8 Packages and import Statements | 418 | ||
Explicit and Wildcard import Statements | 418 | ||
The java.lang Package | 419 | ||
Other API Packages | 419 | ||
6.9 Focus on Object-Oriented Design: Finding the Classes and Their Responsibilities | 420 | ||
Finding the Classes | 420 | ||
Identifying a Class’s Responsibilities | 423 | ||
This Is Only the Beginning | 426 | ||
6.10 Common Errors to Avoid | 426 | ||
Review Questions and Exercises | 427 | ||
Programming Challenges | 432 | ||
Chapter 7 Arrays and the ArrayList Class | 441 | ||
7.1 Introduction to Arrays | 441 | ||
Accessing Array Elements | 443 | ||
Inputting and Outputting Array Contents | 444 | ||
Java Performs Bounds Checking | 447 | ||
Watch Out for Off-by-One Errors | 448 | ||
Array Initialization | 449 | ||
Alternate Array Declaration Notation | 450 | ||
7.2 Processing Array Elements | 451 | ||
Array Length | 453 | ||
The Enhanced for Loop | 454 | ||
Letting the User Specify an Array’s Size | 455 | ||
Reassigning Array Reference Variables | 457 | ||
Copying Arrays | 458 | ||
7.3 Passing Arrays as Arguments to Methods | 460 | ||
7.4 Some Useful Array Algorithms and Operations | 464 | ||
Comparing Arrays | 464 | ||
Summing the Values in a Numeric Array | 465 | ||
Getting the Average of the Values in a Numeric Array | 466 | ||
Finding the Highest and Lowest Values in a Numeric Array | 466 | ||
The SalesData Class | 467 | ||
Partially Filled Arrays | 475 | ||
Working with Arrays and Files | 476 | ||
7.5 Returning Arrays from Methods | 477 | ||
7.6 String Arrays | 479 | ||
Calling String Methods from an Array Element | 481 | ||
7.7 Arrays of Objects | 482 | ||
7.8 The Sequential Search Algorithm | 485 | ||
7.9 Two-Dimensional Arrays | 488 | ||
Initializing a Two-Dimensional Array | 492 | ||
The length Field in a Two-Dimensional Array | 493 | ||
Displaying All the Elements of a Two-Dimensional Array | 495 | ||
Summing All the Elements of a Two-Dimensional Array | 495 | ||
Summing the Rows of a Two-Dimensional Array | 496 | ||
Summing the Columns of a Two-Dimensional Array | 496 | ||
Passing Two-Dimensional Arrays to Methods | 497 | ||
Ragged Arrays | 499 | ||
7.10 Arrays with Three or More Dimensions | 500 | ||
7.11 The Selection Sort and the Binary Search Algorithms | 501 | ||
The Selection Sort Algorithm | 501 | ||
The Binary Search Algorithm | 504 | ||
7.12 Command-Line Arguments and Variable-Length Argument Lists | 506 | ||
Command-Line Arguments | 507 | ||
Variable-Length Argument Lists | 508 | ||
7.13 The ArrayList Class | 510 | ||
Creating and Using an ArrayList Object | 511 | ||
Using the Enhanced for Loop with an ArrayList | 512 | ||
The ArrayList Class’s toString method | 513 | ||
Removing an Item from an ArrayList | 514 | ||
Inserting an Item | 515 | ||
Replacing an Item | 516 | ||
Capacity | 517 | ||
Using the Diamond Operator for Type Inference (Java 7) | 518 | ||
7.14 Common Errors to Avoid | 519 | ||
Review Questions and Exercises | 519 | ||
Programming Challenges | 524 | ||
Chapter 8 A Second Look at Classes and Objects | 531 | ||
8.1 Static Class Members | 531 | ||
A Quick Review of Instance Fields and Instance Methods | 531 | ||
Static Members | 532 | ||
Static Fields | 532 | ||
Static Methods | 535 | ||
8.2 Passing Objects as Arguments to Methods | 538 | ||
8.3 Returning Objects from Methods | 541 | ||
8.4 The toString Method | 543 | ||
8.5 Writing an equals Method | 547 | ||
8.6 Methods That Copy Objects | 550 | ||
Copy Constructors | 552 | ||
8.7 Aggregation | 553 | ||
Aggregation in UML Diagrams | 561 | ||
Security Issues with Aggregate Classes | 561 | ||
Avoid Using null References | 563 | ||
8.8 The this Reference Variable | 566 | ||
Using this to Overcome Shadowing | 567 | ||
Using this to Call an Overloaded Constructor from Another Constructor | 568 | ||
8.9 Enumerated Types | 569 | ||
Enumerated Types Are Specialized Classes | 570 | ||
Switching On an Enumerated Type | 576 | ||
8.10 Garbage Collection | 578 | ||
The finalize Method | 580 | ||
8.11 Focus on Object-Oriented Design: Class Collaboration | 580 | ||
Determining Class Collaborations with CRC Cards | 583 | ||
8.12 Common Errors to Avoid | 584 | ||
Review Questions and Exercises | 585 | ||
Programming Challenges | 589 | ||
Chapter 9 Text Processing and More about Wrapper Classes | 595 | ||
9.1 Introduction to Wrapper Classes | 595 | ||
9.2 Character Testing and Conversion with the Character Class | 596 | ||
Character Case Conversion | 601 | ||
9.3 More String Methods | 604 | ||
Searching for Substrings | 604 | ||
Extracting Substrings | 611 | ||
Methods That Return a Modified String | 615 | ||
The Static valueOf Methods | 616 | ||
9.4 The StringBuilder Class | 618 | ||
The StringBuilder Constructors | 619 | ||
Other StringBuilder Methods | 620 | ||
The toString Method | 623 | ||
9.5 Tokenizing Strings | 629 | ||
9.6 Wrapper Classes for the Numeric Data Types | 633 | ||
The Static toString Methods | 634 | ||
The toBinaryString, toHexString, and toOctalString Methods | 634 | ||
The MIN_VALUE and MAX_VALUE Constants | 634 | ||
Autoboxing and Unboxing | 634 | ||
9.7 Focus on Problem Solving: The TestScoreReader Class | 636 | ||
9.8 Common Errors to Avoid | 640 | ||
Review Questions and Exercises | 641 | ||
Programming Challenges | 644 | ||
Chapter 10 Inheritance | 649 | ||
10.1 What Is Inheritance? | 649 | ||
Generalization and Specialization | 649 | ||
Inheritance and the “Is a” Relationship | 650 | ||
Inheritance in UML Diagrams | 658 | ||
The Superclass’s Constructor | 659 | ||
Inheritance Does Not Work in Reverse | 661 | ||
10.2 Calling the Superclass Constructor | 662 | ||
When the Superclass Has No Default or No-Arg Constructors | 668 | ||
Summary of Constructor Issues in Inheritance | 669 | ||
10.3 Overriding Superclass Methods | 670 | ||
Overloading versus Overriding | 675 | ||
Preventing a Method from Being Overridden | 678 | ||
10.4 Protected Members | 679 | ||
Package Access | 684 | ||
10.5 Chains of Inheritance | 685 | ||
Class Hierarchies | 691 | ||
10.6 The Object Class | 691 | ||
10.7 Polymorphism | 693 | ||
Polymorphism and Dynamic Binding | 694 | ||
The “Is-a” Relationship Does Not Work in Reverse | 696 | ||
The instanceof Operator | 697 | ||
10.8 Abstract Classes and Abstract Methods | 698 | ||
Abstract Classes in UML | 704 | ||
10.9 Interfaces | 705 | ||
An Interface is a Contract | 707 | ||
Fields in Interfaces | 711 | ||
Implementing Multiple Interfaces | 711 | ||
Interfaces in UML | 711 | ||
Default Methods | 712 | ||
Polymorphism and Interfaces | 714 | ||
10.10 Anonymous Inner Classes | 719 | ||
10.11 Functional Interfaces and Lambda Expressions | 722 | ||
10.12 Common Errors to Avoid | 727 | ||
Review Questions and Exercises | 728 | ||
Programming Challenges | 734 | ||
Chapter 11 Exceptions and Advanced File I/O | 739 | ||
11.1 Handling Exceptions | 739 | ||
Exception Classes | 740 | ||
Handling an Exception | 741 | ||
Retrieving the Default Error Message | 745 | ||
Polymorphic References to Exceptions | 748 | ||
Using Multiple catch Clauses to Handle Multiple Exceptions | 748 | ||
The finally Clause | 756 | ||
The Stack Trace | 758 | ||
Handling Multiple Exceptions with One catch Clause (Java 7) | 759 | ||
When an Exception Is Not Caught | 761 | ||
Checked and Unchecked Exceptions | 762 | ||
11.2 Throwing Exceptions | 763 | ||
Creating Your Own Exception Classes | 766 | ||
Using the @exception Tag in Documentation Comments | 769 | ||
11.3 Advanced Topics: Binary Files, Random Access Files, and Object Serialization | 770 | ||
Binary Files | 770 | ||
Random Access Files | 777 | ||
Object Serialization | 782 | ||
Serializing Aggregate Objects | 786 | ||
11.4 Common Errors to Avoid | 787 | ||
Review Questions and Exercises | 787 | ||
Programming Challenges | 793 | ||
Chapter 12 A First Look at GUI Applications | 797 | ||
12.1 Introduction | 797 | ||
The JFC, AWT, and Swing | 798 | ||
Event-Driven Programming | 800 | ||
The javax.swing and java.awt Packages | 800 | ||
12.2 Creating Windows | 800 | ||
Using Inheritance to Extend the JFrame Class | 803 | ||
Equipping GUI Classes with a main Method | 805 | ||
Adding Components to a Window | 807 | ||
Handling Events with Action Listeners | 813 | ||
Writing an Event Listener for the KiloConverter Class | 815 | ||
Background and Foreground Colors | 820 | ||
The ActionEvent Object | 824 | ||
12.3 Layout Managers | 829 | ||
Adding a Layout Manager to a Container | 830 | ||
The FlowLayout Manager | 830 | ||
The BorderLayout Manager | 833 | ||
The GridLayout Manager | 840 | ||
12.4 Radio Buttons and Check Boxes | 846 | ||
Radio Buttons | 846 | ||
Check Boxes | 852 | ||
12.5 Borders | 857 | ||
12.6 Focus on Problem Solving: Extending Classes from JPanel | 860 | ||
The Brandi’s Bagel House Application | 860 | ||
The GreetingPanel Class | 861 | ||
The BagelPanel Class | 862 | ||
The ToppingPanel Class | 864 | ||
The CoffeePanel Class | 866 | ||
Putting It All Together | 868 | ||
12.7 Splash Screens | 872 | ||
12.8 Using Console Output to Debug a GUI Application | 873 | ||
12.9 Common Errors to Avoid | 878 | ||
Review Questions and Exercises | 878 | ||
Programming Challenges | 881 | ||
Chapter 13 Advanced GUI Applications | 885 | ||
13.1 The Swing and AWT Class Hierarchy | 885 | ||
13.2 Read-Only Text Fields | 886 | ||
13.3 Lists | 888 | ||
Selection Modes | 888 | ||
Responding to List Events | 889 | ||
Retrieving the Selected Item | 890 | ||
Placing a Border around a List | 894 | ||
Adding a Scroll Bar to a List | 894 | ||
Adding Items to an Existing JList Component | 899 | ||
Multiple Selection Lists | 899 | ||
13.4 Combo Boxes | 904 | ||
Retrieving the Selected Item | 905 | ||
13.5 Displaying Images in Labels and Buttons | 910 | ||
13.6 Mnemonics and Tool Tips | 916 | ||
Mnemonics | 916 | ||
Tool Tips | 918 | ||
13.7 File Choosers and Color Choosers | 918 | ||
File Choosers | 919 | ||
Color Choosers | 921 | ||
13.8 Menus | 922 | ||
13.9 More about Text Components: Text Areas and Fonts | 931 | ||
Text Areas | 931 | ||
Fonts | 934 | ||
13.10 Sliders | 935 | ||
13.11 Look and Feel | 940 | ||
13.12 Common Errors to Avoid | 942 | ||
Review Questions and Exercises | 943 | ||
Programming Challenges | 948 | ||
Chapter 14 Applets and More | 953 | ||
14.1 Introduction to Applets | 953 | ||
14.2 A Brief Introduction to HTML | 955 | ||
Hypertext | 955 | ||
Markup Language | 956 | ||
Document Structure Tags | 956 | ||
Text Formatting Tags | 958 | ||
Creating Breaks in Text | 960 | ||
Inserting Links | 963 | ||
14.3 Creating Applets with Swing | 964 | ||
Running an Applet | 966 | ||
Handling Events in an Applet | 968 | ||
14.4 Using AWT for Portability | 973 | ||
14.5 Drawing Shapes | 978 | ||
The XY Coordinate System | 978 | ||
Graphics Objects | 978 | ||
The repaint Method | 992 | ||
Drawing on Panels | 993 | ||
14.6 Handling Mouse Events | 999 | ||
Handling Mouse Events | 999 | ||
14.7 Timer Objects | 1009 | ||
14.8 Playing Audio | 1013 | ||
Using an AudioClip Object | 1014 | ||
Playing Audio in an Application | 1017 | ||
14.9 Common Errors to Avoid | 1018 | ||
Review Questions and Exercises | 1018 | ||
Programming Challenges | 1024 | ||
Chapter 15 Creating GUI Applications with JavaFX and Scene Builder | 1027 | ||
15.1 Introduction | 1027 | ||
Event-Driven Programming | 1029 | ||
15.2 Scene Graphs | 1029 | ||
15.3 Using Scene Builder to Create JavaFX Applications | 1031 | ||
Starting Scene Builder | 1032 | ||
The Scene Builder Main Window | 1033 | ||
15.4 Writing the Application Code | 1045 | ||
The Main Application Class | 1046 | ||
The Controller Class | 1048 | ||
Using the Sample Controller Skeleton | 1053 | ||
Summary of Creating a JavaFX Application | 1054 | ||
15.5 RadioButtons and CheckBoxes | 1055 | ||
RadioButtons | 1055 | ||
Determining in Code Whether a RadioButton Is Selected | 1057 | ||
Responding to RadioButton Events | 1060 | ||
CheckBoxes | 1063 | ||
Determining in Code Whether a CheckBox Is Selected | 1064 | ||
Responding to CheckBox Events | 1066 | ||
15.6 Displaying Images | 1069 | ||
Displaying an Image with Code | 1070 | ||
15.7 Common Errors to Avoid | 1074 | ||
Review Questions and Exercises | 1074 | ||
Programming Challenges | 1078 | ||
Chapter 16 Recursion | 1083 | ||
16.1 Introduction to Recursion | 1083 | ||
16.2 Solving Problems with Recursion | 1086 | ||
Direct and Indirect Recursion | 1090 | ||
16.3 Examples of Recursive Methods | 1091 | ||
Summing a Range of Array Elements with Recursion | 1091 | ||
Drawing Concentric Circles | 1092 | ||
The Fibonacci Series | 1094 | ||
Finding the Greatest Common Divisor | 1096 | ||
16.4 A Recursive Binary Search Method | 1097 | ||
16.5 The Towers of Hanoi | 1100 | ||
16.6 Common Errors to Avoid | 1105 | ||
Review Questions and Exercises | 1105 | ||
Programming Challenges | 1108 | ||
Chapter 17 Databases | 1111 | ||
17.1 Introduction to Database Management Systems | 1111 | ||
JDBC | 1112 | ||
SQL | 1113 | ||
Using a DBMS | 1113 | ||
Java DB | 1114 | ||
Creating the CoffeeDB Database | 1114 | ||
Connecting to the CoffeeDB Database | 1114 | ||
Connecting to a Password-Protected Database | 1116 | ||
17.2 Tables, Rows, and Columns | 1117 | ||
Column Data Types | 1119 | ||
Primary Keys | 1119 | ||
17.3 Introduction to the SQL SELECT Statement | 1120 | ||
Passing an SQL Statement to the DBMS | 1122 | ||
Specifying Search Criteria with the WHERE Clause | 1132 | ||
Sorting the Results of a SELECT Query | 1138 | ||
Mathematical Functions | 1139 | ||
17.4 Inserting Rows | 1142 | ||
Inserting Rows with JDBC | 1144 | ||
17.5 Updating and Deleting Existing Rows | 1146 | ||
Updating Rows with JDBC | 1147 | ||
Deleting Rows with the DELETE Statement | 1151 | ||
Deleting Rows with JDBC | 1151 | ||
17.6 Creating and Deleting Tables | 1155 | ||
Removing a Table with the DROP TABLE Statement | 1158 | ||
17.7 Creating a New Database with JDBC | 1158 | ||
17.8 Scrollable Result Sets | 1160 | ||
17.9 Result Set Metadata | 1161 | ||
17.10 Displaying Query Results in a JTable | 1165 | ||
17.11 Relational Data | 1175 | ||
Joining Data from Multiple Tables | 1178 | ||
An Order Entry System | 1179 | ||
17.12 Advanced Topics | 1197 | ||
Transactions | 1197 | ||
Stored Procedures | 1198 | ||
17.13 Common Errors to Avoid | 1199 | ||
Review Questions and Exercises | 1199 | ||
Programming Challenges | 1204 | ||
Index | 1207 | ||
A | 1207 | ||
B | 1208 | ||
C | 1209 | ||
D | 1210 | ||
E | 1211 | ||
F | 1212 | ||
G | 1213 | ||
H | 1213 | ||
I | 1214 | ||
J | 1214 | ||
K | 1216 | ||
L | 1216 | ||
M | 1216 | ||
N | 1217 | ||
O | 1217 | ||
P | 1218 | ||
Q | 1219 | ||
R | 1219 | ||
S | 1220 | ||
T | 1222 | ||
U | 1223 | ||
V | 1223 | ||
W | 1223 | ||
X | 1224 | ||
Z | 1224 |