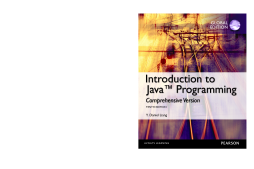
Additional Information
Book Details
Abstract
This text is intended for a 1-, 2-, or 3-semester CS1 course sequence.
Daniel Liang teaches concepts of problem-solving and object-oriented programming using a fundamentals-first approach. Beginning programmers learn critical problem-solving techniques then move on to grasp the key concepts of object-oriented, GUI programming, advanced GUI and Web programming using Java.
Teaching and Learning Experience
To provide a better teaching and learning experience, for both instructors and students, this program offers:
-
Fundamentals-First Approach: Basic programming concepts are introduced on control statements, loops, functions, and arrays before object-oriented programming is discussed.
-
Problem-Driven Motivation: The examples and exercises throughout the book emphasize problem solving and foster the concept of developing reusable components and using them to create practical projects.
-
A Superior Pedagogical Design that Fosters Student Interest: Key concepts are reinforced with objectives lists, introduction and chapter overviews, easy-to-follow examples, chapter summaries, review questions, programming exercises, and interactive self-tests.
-
The Most Extensive Instructor and Student Support Package Available
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Title | Title | ||
Copyright | Copyright | ||
Contents | 12 | ||
Chapter 1 Introduction to Computers, Programs, and Java | 23 | ||
1.1 Introduction | 24 | ||
1.2 What Is a Computer? | 24 | ||
1.3 Programming Languages | 29 | ||
1.4 Operating Systems | 31 | ||
1.5 Java, the World Wide Web, and Beyond | 32 | ||
1.6 The Java Language Specification, API, JDK, and IDE | 33 | ||
1.7 A Simple Java Program | 34 | ||
1.8 Creating, Compiling, and Executing a Java Program | 37 | ||
1.9 Programming Style and Documentation | 40 | ||
1.10 Programming Errors | 42 | ||
1.11 Developing Java Programs Using NetBeans | 45 | ||
1.12 Developing Java Programs Using Eclipse | 47 | ||
Chapter 2 Elementary Programming | 55 | ||
2.1 Introduction | 56 | ||
2.2 Writing a Simple Program | 56 | ||
2.3 Reading Input from the Console | 59 | ||
2.4 Identifiers | 61 | ||
2.5 Variables | 62 | ||
2.6 Assignment Statements and Assignment Expressions | 63 | ||
2.7 Named Constants | 65 | ||
2.8 Naming Conventions | 66 | ||
2.9 Numeric Data Types and Operations | 66 | ||
2.10 Numeric Literals | 70 | ||
2.11 Evaluating Expressions and Operator Precedence | 72 | ||
2.12 Case Study: Displaying the Current Time | 74 | ||
2.13 Augmented Assignment Operators | 76 | ||
2.14 Increment and Decrement Operators | 77 | ||
2.15 Numeric Type Conversions | 78 | ||
2.16 Software Development Process | 81 | ||
2.17 Case Study: Counting Monetary Units | 85 | ||
2.18 Common Errors and Pitfalls | 87 | ||
Chapter 3 Selections | 97 | ||
3.1 Introduction | 98 | ||
3.2 boolean Data Type | 98 | ||
3.3 if Statements | 100 | ||
3.4 Two-Way if-else Statements | 102 | ||
3.5 Nested if and Multi-Way if-else Statements | 103 | ||
3.6 Common Errors and Pitfalls | 105 | ||
3.7 Generating Random Numbers | 109 | ||
3.8 Case Study: Computing Body Mass Index | 111 | ||
3.9 Case Study: Computing Taxes | 112 | ||
3.10 Logical Operators | 115 | ||
3.11 Case Study: Determining Leap Year | 119 | ||
3.12 Case Study: Lottery | 120 | ||
3.13 switch Statements | 122 | ||
3.14 Conditional Expressions | 125 | ||
3.15 Operator Precedence and Associativity | 126 | ||
3.16 Debugging | 128 | ||
Chapter 4 Mathematical Functions, Characters, and Strings | 141 | ||
4.1 Introduction | 142 | ||
4.2 Common Mathematical Functions | 142 | ||
4.3 Character Data Type and Operations | 147 | ||
4.4 The String Type | 152 | ||
4.5 Case Studies | 161 | ||
4.6 Formatting Console Output | 167 | ||
Chapter 5 Loops | 179 | ||
5.1 Introduction | 180 | ||
5.2 The while Loop | 180 | ||
5.3 The do-while Loop | 190 | ||
5.4 The for Loop | 192 | ||
5.5 Which Loop to Use? | 196 | ||
5.6 Nested Loops | 198 | ||
5.7 Minimizing Numeric Errors | 200 | ||
5.8 Case Studies | 201 | ||
5.9 Keywords break and continue | 206 | ||
5.10 Case Study: Checking Palindromes | 209 | ||
5.11 Case Study: Displaying Prime Numbers | 210 | ||
Chapter 6 Methods | 225 | ||
6.1 Introduction | 226 | ||
6.2 Defining a Method | 226 | ||
6.3 Calling a Method | 228 | ||
6.4 Void Method Example | 231 | ||
6.5 Passing Arguments by Values | 234 | ||
6.6 Modularizing Code | 237 | ||
6.7 Case Study: Converting Hexadecimals to Decimals | 239 | ||
6.8 Overloading Methods | 241 | ||
6.9 The Scope of Variables | 244 | ||
6.10 Case Study: Generating Random Characters | 245 | ||
6.11 Method Abstraction and Stepwise Refinement | 247 | ||
Chapter 7 Single-Dimensional Arrays | 267 | ||
7.1 Introduction | 268 | ||
7.2 Array Basics | 268 | ||
7.3 Case Study: Analyzing Numbers | 275 | ||
7.4 Case Study: Deck of Cards | 276 | ||
7.5 Copying Arrays | 278 | ||
7.6 Passing Arrays to Methods | 279 | ||
7.7 Returning an Array from a Method | 282 | ||
7.8 Case Study: Counting the Occurrences of Each Letter | 283 | ||
7.9 Variable-Length Argument Lists | 286 | ||
7.10 Searching Arrays | 287 | ||
7.11 Sorting Arrays | 291 | ||
7.12 The Arrays Class | 292 | ||
7.13 Command-Line Arguments | 294 | ||
Chapter 8 Multidimensional Arrays | 309 | ||
8.1 Introduction | 310 | ||
8.2 Two-Dimensional Array Basics | 310 | ||
8.3 Processing Two-Dimensional Arrays | 313 | ||
8.4 Passing Two-Dimensional Arrays to Methods | 315 | ||
8.5 Case Study: Grading a Multiple-Choice Test | 316 | ||
8.6 Case Study: Finding the Closest Pair | 318 | ||
8.7 Case Study: Sudoku | 320 | ||
8.8 Multidimensional Arrays | 323 | ||
Chapter 9 Objects and Classes | 343 | ||
9.1 Introduction | 344 | ||
9.2 Defining Classes for Objects | 344 | ||
9.3 Example: Defining Classes and Creating Objects | 346 | ||
9.4 Constructing Objects Using Constructors | 351 | ||
9.5 Accessing Objects via Reference Variables | 352 | ||
9.6 Using Classes from the Java Library | 356 | ||
9.7 Static Variables, Constants, and Methods | 359 | ||
9.8 Visibility Modifiers | 364 | ||
9.9 Data Field Encapsulation | 366 | ||
9.10 Passing Objects to Methods | 369 | ||
9.11 Array of Objects | 373 | ||
9.12 Immutable Objects and Classes | 375 | ||
9.13 The Scope of Variables | 377 | ||
9.14 The this Reference | 378 | ||
Chapter 10 Object-Oriented Thinking | 387 | ||
10.1 Introduction | 388 | ||
10.2 Class Abstraction and Encapsulation | 388 | ||
10.3 Thinking in Objects | 392 | ||
10.4 Class Relationships | 395 | ||
10.5 Case Study: Designing the Course Class | 398 | ||
10.6 Case Study: Designing a Class for Stacks | 400 | ||
10.7 Processing Primitive Data Type Values as Objects | 402 | ||
10.8 Automatic Conversion between Primitive Types and Wrapper Class Types\r | 405 | ||
10.9 The BigInteger and BigDecimal Classes | 406 | ||
10.10 The String Class | 408 | ||
10.11 The StringBuilder and StringBuffer Classes | 414 | ||
Chapter 11 Inheritance and Polymorphism | 431 | ||
11.1 Introduction | 432 | ||
11.2 Superclasses and Subclasses | 432 | ||
11.3 Using the super Keyword | 438 | ||
11.4 Overriding Methods | 441 | ||
11.5 Overriding vs. Overloading | 442 | ||
11.6 The Object Class and Its toString() Method | 444 | ||
11.7 Polymorphism | 445 | ||
11.8 Dynamic Binding | 446 | ||
11.9 Casting Objects and the instanceof Operator | 449 | ||
11.10 The Object’s equals Method | 453 | ||
11.11 The ArrayList Class | 454 | ||
11.12 Useful Methods for Lists | 460 | ||
11.13 Case Study: A Custom Stack Class | 461 | ||
11.14 The protected Data and Methods | 462 | ||
11.15 Preventing Extending and Overriding | 464 | ||
Chapter 12 Exception Handling and Text I/O | 471 | ||
12.1 Introduction | 472 | ||
12.2 Exception-Handling Overview | 472 | ||
12.3 Exception Types | 477 | ||
12.4 More on Exception Handling | 480 | ||
12.5 The finally Clause | 488 | ||
12.6 When to Use Exceptions | 489 | ||
12.7 Rethrowing Exceptions | 490 | ||
12.8 Chained Exceptions | 491 | ||
12.9 Defining Custom Exception Classes | 492 | ||
12.10 The File Class | 495 | ||
12.11 File Input and Output | 498 | ||
12.12 Reading Data from the Web | 504 | ||
12.13 Case Study: Web Crawler | 506 | ||
Chapter 13 Abstract Classes and Interfaces | 517 | ||
13.1 Introduction | 518 | ||
13.2 Abstract Classes | 518 | ||
13.3 Case Study: the Abstract Number Class | 523 | ||
13.4 Case Study: Calendar and GregorianCalendar | 525 | ||
13.5 Interfaces | 528 | ||
13.6 The Comparable Interface | 531 | ||
13.7 The Cloneable Interface | 535 | ||
13.8 Interfaces vs. Abstract Classes | 539 | ||
13.9 Case Study: The Rational Class | 542 | ||
13.10 Class Design Guidelines | 547 | ||
Chapter 14 JavaFX Basics | 557 | ||
14.1 Introduction | 558 | ||
14.2 JavaFX vs Swing and AWT | 558 | ||
14.3 The Basic Structure of a JavaFX Program | 558 | ||
14.4 Panes, UI Controls, and Shapes | 561 | ||
14.5 Property Binding | 564 | ||
14.6 Common Properties and Methods for Nodes | 567 | ||
14.7 The Color Class | 568 | ||
14.8 The Font Class | 569 | ||
14.9 The Image and ImageView Classes | 571 | ||
14.10 Layout Panes | 574 | ||
14.11 Shapes | 582 | ||
14.12 Case Study: The ClockPane Class | 594 | ||
Chapter 15 Event-Driven Programming and Animations | 607 | ||
15.1 Introduction | 608 | ||
15.2 Events and Event Sources | 610 | ||
15.3 Registering Handlers and Handling Events | 611 | ||
15.4 Inner Classes | 615 | ||
15.5 Anonymous Inner Class Handlers | 616 | ||
15.6 Simplifying Event Handling Using Lambda Expressions | 619 | ||
15.7 Case Study: Loan Calculator | 622 | ||
15.8 Mouse Events | 624 | ||
15.9 Key Events | 625 | ||
15.10 Listeners for Observable Objects | 628 | ||
15.11 Animation | 630 | ||
15.12 Case Study: Bouncing Ball | 638 | ||
Chapter 16 JavaFX UI Controls and Multimedia | 651 | ||
16.1 Introduction | 652 | ||
16.2 Labeled and Label | 652 | ||
16.3 Button | 654 | ||
16.4 CheckBox | 656 | ||
16.5 RadioButton | 659 | ||
16.6 TextField | 661 | ||
16.7 TextArea | 663 | ||
16.8 ComboBox | 666 | ||
16.9 ListView | 669 | ||
16.10 ScrollBar | 673 | ||
16.11 Slider | 676 | ||
16.12 Case Study: Developing a Tic-Tac-Toe Game | 679 | ||
16.13 Video and Audio | 684 | ||
16.14 Case Study: National Flags and Anthems | 687 | ||
Chapter 17 Binary I/O | 699 | ||
17.1 Introduction | 700 | ||
17.2 How Is Text I/O Handled in Java? | 700 | ||
17.3 Text I/O vs. Binary I/O | 701 | ||
17.4 Binary I/O Classes | 702 | ||
17.5 Case Study: Copying Files | 713 | ||
17.6 Object I/O | 714 | ||
17.7 Random-Access Files | 719 | ||
Chapter 18 Recursion | 727 | ||
18.1 Introduction | 728 | ||
18.2 Case Study: Computing Factorials | 728 | ||
18.3 Case Study: Computing Fibonacci Numbers | 731 | ||
18.4 Problem Solving Using Recursion | 734 | ||
18.5 Recursive Helper Methods | 736 | ||
18.6 Case Study: Finding the Directory Size | 739 | ||
18.7 Case Study: Tower of Hanoi | 741 | ||
18.8 Case Study: Fractals | 744 | ||
18.9 Recursion vs. Iteration | 748 | ||
18.10 Tail Recursion | 749 | ||
Chapter 19 Generics | 759 | ||
19.1 Introduction | 760 | ||
19.2 Motivations and Benefits | 760 | ||
19.3 Defining Generic Classes and Interfaces | 762 | ||
19.4 Generic Methods | 764 | ||
19.5 Case Study: Sorting an Array of Objects | 766 | ||
19.6 Raw Types and Backward Compatibility | 768 | ||
19.7 Wildcard Generic Types | 769 | ||
19.8 Erasure and Restrictions on Generics | 772 | ||
19.9 Case Study: Generic Matrix Class | 774 | ||
Chapter 20 Lists, Stacks, Queues, and Priority Queues | 783 | ||
20.1 Introduction | 784 | ||
20.2 Collections | 784 | ||
20.3 Iterators | 788 | ||
20.4 Lists | 789 | ||
20.5 The Comparator Interface | 794 | ||
20.6 Static Methods for Lists and Collections | 795 | ||
20.7 Case Study: Bouncing Balls | 799 | ||
20.8 Vector and Stack Classes | 803 | ||
20.9 Queues and Priority Queues | 805 | ||
20.10 Case Study: Evaluating Expressions | 808 | ||
Chapter 21 Sets and Maps | 819 | ||
21.1 Introduction | 820 | ||
21.2 Sets | 820 | ||
21.3 Comparing the Performance of Sets and Lists | 828 | ||
21.4 Case Study: Counting Keywords | 831 | ||
21.5 Maps | 832 | ||
21.6 Case Study: Occurrences of Words | 837 | ||
21.7 Singleton and Unmodifiable Collections and Maps | 838 | ||
Chapter 22 Developing Efficient Algorithms | 843 | ||
22.1 Introduction | 844 | ||
22.2 Measuring Algorithm Efficiency Using Big O Notation | 844 | ||
22.3 Examples: Determining Big O | 846 | ||
22.4 Analyzing Algorithm Time Complexity | 850 | ||
22.5 Finding Fibonacci Numbers Using Dynamic Programming | 853 | ||
22.6 Finding Greatest Common Divisors Using Euclid’s Algorithm | 855 | ||
22.7 Efficient Algorithms for Finding Prime Numbers | 859 | ||
22.8 Finding the Closest Pair of Points Using Divide-and-Conquer | 865 | ||
22.9 Solving the Eight Queens Problem Using Backtracking | 868 | ||
22.10 Computational Geometry: Finding a Convex Hull | 871 | ||
Chapter 23 Sorting | 883 | ||
23.1 Introduction | 884 | ||
23.2 Insertion Sort | 884 | ||
23.3 Bubble Sort | 886 | ||
23.4 Merge Sort | 889 | ||
23.5 Quick Sort | 892 | ||
23.6 Heap Sort | 896 | ||
23.7 Bucket Sort and Radix Sort | 903 | ||
23.8 External Sort | 905 | ||
Chapter 24 Implementing Lists, Stacks, Queues,and Priority Queues | 917 | ||
24.1 Introduction | 918 | ||
24.2 Common Features for Lists | 918 | ||
24.3 Array Lists | 922 | ||
24.4 Linked Lists | 928 | ||
24.5 Stacks and Queues | 942 | ||
24.6 Priority Queues | 946 | ||
Chapter 25 Binary Search Trees | 951 | ||
25.1 Introduction | 952 | ||
25.2 Binary Search Trees | 952 | ||
25.3 Deleting Elements from a BST | 965 | ||
25.4 Tree Visualization and MVC | 971 | ||
25.5 Iterators | 974 | ||
25.6 Case Study: Data Compression | 976 | ||
Chapter 26 AVL Trees | 987 | ||
26.1 Introduction | 988 | ||
26.2 Rebalancing Trees | 988 | ||
26.3 Designing Classes for AVL Trees | 991 | ||
26.4 Overriding the insert Method | 992 | ||
26.5 Implementing Rotations | 993 | ||
26.6 Implementing the delete Method | 994 | ||
26.7 The AVLTree Class | 994 | ||
26.8 Testing the AVLTree Class | 1000 | ||
26.9 AVL Tree Time Complexity Analysis | 1003 | ||
Chapter 27 Hashing | 1007 | ||
27.1 Introduction | 1008 | ||
27.2 What Is Hashing? | 1008 | ||
27.3 Hash Functions and Hash Codes | 1009 | ||
27.4 Handling Collisions Using Open Addressing | 1011 | ||
27.5 Handling Collisions Using Separate Chaining | 1015 | ||
27.6 Load Factor and Rehashing | 1015 | ||
27.7 Implementing a Map Using Hashing | 1017 | ||
27.8 Implementing Set Using Hashing | 1026 | ||
Chapter 28 Graphs and Applications | 1037 | ||
28.1 Introduction | 1038 | ||
28.2 Basic Graph Terminologies | 1039 | ||
28.3 Representing Graphs | 1041 | ||
28.4 Modeling Graphs | 1046 | ||
28.5 Graph Visualization | 1056 | ||
28.6 Graph Traversals | 1059 | ||
28.7 Depth-First Search (DFS) | 1060 | ||
28.8 Case Study: The Connected Circles Problem | 1064 | ||
28.9 Breadth-First Search (BFS) | 1067 | ||
28.10 Case Study: The Nine Tails Problem | 1070 | ||
Chapter 29 Weighted Graphs and Applications | 1083 | ||
29.1 Introduction | 1084 | ||
29.2 Representing Weighted Graphs | 1085 | ||
29.3 The WeightedGraph Class | 1087 | ||
29.4 Minimum Spanning Trees | 1094 | ||
29.5 Finding Shortest Paths | 1100 | ||
29.6 Case Study: The Weighted Nine Tails Problem | 1108 | ||
Chapter 30 Multithreading and Parallel Programming | 1119 | ||
30.1 Introduction | 1120 | ||
30.2 Thread Concepts | 1120 | ||
30.3 Creating Tasks and Threads | 1120 | ||
30.4 The Thread Class | 1124 | ||
30.5 Case Study: Flashing Text | 1127 | ||
30.6 Thread Pools | 1128 | ||
30.7 Thread Synchronization | 1130 | ||
30.8 Synchronization Using Locks | 1134 | ||
30.9 Cooperation among Threads | 1136 | ||
30.10 Case Study: Producer/Consumer | 1141 | ||
30.11 Blocking Queues | 1144 | ||
30.12 Semaphores | 1146 | ||
30.13 Avoiding Deadlocks | 1148 | ||
30.14 Thread States | 1148 | ||
30.15 Synchronized Collections | 1149 | ||
30.16 Parallel Programming | 1150 | ||
Chapter 31 Networking | 1161 | ||
31.1 Introduction | 1162 | ||
31.2 Client/Server Computing | 1162 | ||
31.3 The InetAddress Class | 1169 | ||
31.4 Serving Multiple Clients | 1170 | ||
31.5 Sending and Receiving Objects | 1173 | ||
31.6 Case Study: Distributed Tic-Tac-Toe Games | 1178 | ||
Chapter 32 Java Database Programming | 1195 | ||
32.1 Introduction | 1196 | ||
32.2 Relational Database Systems | 1196 | ||
32.3 SQL | 1200 | ||
32.4 JDBC | 1211 | ||
32.5 PreparedStatement | 1219 | ||
32.6 CallableStatement | 1221 | ||
32.7 Retrieving Metadata | 1224 | ||
Chapter 33 JavaServer Faces | 1235 | ||
33.1 Introduction | 1236 | ||
33.2 Getting Started with JSF | 1236 | ||
33.3 JSF GUI Components | 1244 | ||
33.4 Processing the Form | 1248 | ||
33.5 Case Study: Calculator | 1252 | ||
33.6 Session Tracking | 1255 | ||
33.7 Validating Input | 1257 | ||
33.8 Binding Database with Facelets | 1261 | ||
33.9 Opening New JSF Pages | 1267 | ||
Appendixes | 1285 | ||
Appendix A\tJava Keywords | 1287 | ||
Appendix B The ASCII Character Set | 1288 | ||
Appendix C Operator Precedence Chart | 1290 | ||
Appendix D Java Modifiers | 1292 | ||
Appendix E Special Floating-Point Values | 1294 | ||
Appendix F Number Systems | 1295 | ||
Appendix G Bitwise Operations | 1299 | ||
Appendix H Regular Expressions | 1300 | ||
Appendix I Enumerated Types | 1305 | ||
Index | 1311 | ||
Symbols | 1311 | ||
Numbers | 1311 | ||
A | 1311 | ||
B | 1313 | ||
C | 1315 | ||
D | 1318 | ||
E | 1320 | ||
F | 1321 | ||
G | 1322 | ||
H | 1323 | ||
I | 1324 | ||
J | 1326 | ||
K | 1327 | ||
L | 1328 | ||
M | 1329 | ||
N | 1331 | ||
O | 1332 | ||
P | 1333 | ||
Q | 1335 | ||
R | 1335 | ||
S | 1336 | ||
T | 1339 | ||
U | 1341 | ||
V | 1341 | ||
W | 1342 | ||
X | 1342 |