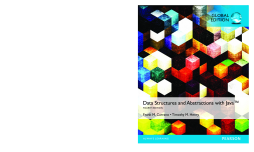
BOOK
Data Structures and Abstractions with Java, Global Edition
Timothy M. Henry | Frank M. Carrano
(2015)
Additional Information
Book Details
Abstract
Data Structures and Abstractions with Java is suitable for one- or two-semester courses in data structures (CS-2) in the departments of Computer Science, Computer Engineering, Business, and Management Information Systems.
This is the most student-friendly data structures text available that introduces ADTs in individual, brief chapters – each with pedagogical tools to help students master each concept. Using the latest features of Java, this unique object-oriented presentation makes a clear distinction between specification and implementation to simplify learning, while providing maximum classroom flexibility.
Teaching and Learning Experience
This book will provide a better teaching and learning experience–for you and your students. It will help:
- Aid comprehension and facilitate teaching with an approachable format and content organization: Material is organized into small segments that focus a reader’s attention and provide greater instructional flexibility.
- Keep your course current with updated material: Content is refreshed throughout the book to reflect the latest advancements and to refine the pedagogy. All of the Java code is Java 8 compatible.
- Support learning with student-friendly pedagogy: In-text and online features help students master the material.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Title Page | Title Page | ||
Copyright | Copyright | ||
Contents | 15 | ||
Introduction: Organizing Data | 31 | ||
Prelude: Designing Classes | 35 | ||
Encapsulation | 36 | ||
Specifying Methods | 38 | ||
Comments | 38 | ||
Preconditions and Postconditions | 39 | ||
Assertions | 40 | ||
Java Interfaces | 41 | ||
Writing an Interface | 42 | ||
Implementing an Interface | 43 | ||
An Interface as a Data Type | 45 | ||
Extending an Interface | 46 | ||
Named Constants Within an Interface | 47 | ||
Choosing Classes | 49 | ||
Identifying Classes | 50 | ||
CRC Cards | 51 | ||
The Unified Modeling Language | 51 | ||
Reusing Classes | 54 | ||
Chapter 1: Bags\r | 61 | ||
The Bag | 62 | ||
A Bag’s Behaviors | 62 | ||
Specifying a Bag | 63 | ||
An Interface | 69 | ||
Using the ADT Bag | 71 | ||
Using an ADT Is Like Using a Vending Machine | 75 | ||
The ADT Set | 77 | ||
Java Class Library: The Interface set | 77 | ||
Java Interlude 1: Generics\r | 83 | ||
Generic Data Types | 83 | ||
Generic Types Within an Interface | 84 | ||
Generic Classes | 85 | ||
Chapter 2: Bag Implementations That Use Arrays\r | 89 | ||
Using a Fixed-Size Array to Implement the ADT Bag | 90 | ||
An Analogy | 90 | ||
A Group of Core Methods | 91 | ||
Implementing the Core Methods | 92 | ||
Making the Implementation Secure | 99 | ||
Testing the Core Methods | 101 | ||
Implementing More Methods | 103 | ||
Methods That Remove Entries | 106 | ||
Using Array Resizing to Implement the ADT Bag | 114 | ||
Resizing an Array | 114 | ||
A New Implementation of a Bag | 117 | ||
The Pros and Cons of Using an Array to Implement the ADT Bag | 120 | ||
Java Interlude 2: Exceptions\r | 125 | ||
The Basics | 126 | ||
Handling an Exception | 128 | ||
Postpone Handling: The throws Clause | 128 | ||
Handle It Now: The try-catch Blocks | 129 | ||
Multiple catch Blocks | 130 | ||
Throwing an Exception | 131 | ||
Chapter 3: A Bag Implementation That Links Data\r | 133 | ||
Linked Data | 134 | ||
Forming a Chain by Adding to Its Beginning | 135 | ||
A Linked Implementation of the ADT Bag | 137 | ||
The Private Class Node | 137 | ||
An Outline of the Class LinkedBag | 138 | ||
Defining Some Core Methods | 139 | ||
Testing the Core Methods | 143 | ||
The Method getFrequencyOf | 144 | ||
The Method contains | 145 | ||
Removing an Item from a Linked Chain | 146 | ||
The Methods remove and clear | 147 | ||
A Class Node That Has Set and Get Methods | 151 | ||
The Pros and Cons of Using a Chain to Implement the ADT Bag | 154 | ||
Chapter 4: The Efficiency of Algorithms\r | 159 | ||
Motivation | 160 | ||
Measuring an Algorithm’s Efficiency | 161 | ||
Counting Basic Operations | 163 | ||
Best, Worst, and Average Cases | 165 | ||
Big Oh Notation | 166 | ||
The Complexities of Program Constructs | 168 | ||
Picturing Efficiency | 170 | ||
The Efficiency of Implementations of the ADT Bag | 173 | ||
An Array-Based Implementation | 173 | ||
A Linked Implementation | 175 | ||
Comparing the Implementations | 176 | ||
Chapter 5: Stacks\r | 183 | ||
Specifications of the ADT Stack | 184 | ||
Using a Stack to Process Algebraic Expressions | 188 | ||
A Problem Solved: Checking for Balanced Delimiters in an Infix Algebraic Expression | 189 | ||
A Problem Solved: Transforming an Infix Expression to a Postfix Expression | 194 | ||
A Problem Solved: Evaluating Postfix Expressions | 199 | ||
A Problem Solved: Evaluating Infix Expressions | 201 | ||
The Program Stack | 203 | ||
Java Class Library: The Class Stack | 204 | ||
Chapter 6: Stack Implementations\r | 211 | ||
A Linked Implementation | 211 | ||
An Array-Based Implementation | 215 | ||
A Vector-Based Implementation | 219 | ||
Java Class Library: The Class Vector | 220 | ||
Using a Vector to Implement the ADT Stack | 220 | ||
Chapter 7: Recursion\r | 227 | ||
What Is Recursion? | 228 | ||
Tracing a Recursive Method | 232 | ||
Recursive Methods That Return a Value | 235 | ||
Recursively Processing an Array | 237 | ||
Recursively Processing a Linked Chain | 240 | ||
The Time Efficiency of Recursive Methods | 241 | ||
The Time Efficiency of countDown | 242 | ||
The Time Efficiency of Computing xn | 243 | ||
A Simple Solution to a Difficult Problem | 244 | ||
A Poor Solution to a Simple Problem | 249 | ||
Tail Recursion | 251 | ||
Indirect Recursion | 253 | ||
Using a Stack Instead of Recursion | 254 | ||
Java Interlude 3: More About Generics\r | 265 | ||
The Interface Comparable | 265 | ||
Generic Methods | 267 | ||
Bounded Type Parameters | 268 | ||
Wildcards | 270 | ||
Bounded Wildcards | 271 | ||
Chapter 8: An Introduction to Sorting\r | 275 | ||
Organizing Java Methods That Sort an Array | 276 | ||
Selection Sort | 277 | ||
Iterative Selection Sort | 278 | ||
Recursive Selection Sort | 280 | ||
The Efficiency of Selection Sort | 281 | ||
Insertion Sort | 281 | ||
Iterative Insertion Sort | 283 | ||
Recursive Insertion Sort | 285 | ||
The Efficiency of Insertion Sort | 287 | ||
Insertion Sort of a Chain of Linked Nodes | 287 | ||
Shell Sort | 290 | ||
The Algorithm | 292 | ||
The Efficiency of Shell Sort | 293 | ||
Comparing the Algorithms | 293 | ||
Chapter 9: Faster Sorting Methods\x0B | 301 | ||
Merge Sort | 302 | ||
Merging Arrays | 302 | ||
Recursive Merge Sort | 303 | ||
The Efficiency of Merge Sort | 305 | ||
Iterative Merge Sort | 307 | ||
Merge Sort in the Java Class Library | 307 | ||
Quick Sort | 308 | ||
The Efficiency of Quick Sort | 308 | ||
Creating the Partition | 309 | ||
Implementing Quick Sort | 312 | ||
Quick Sort in the Java Class Library | 314 | ||
Radix Sort | 314 | ||
Pseudocode for Radix Sort | 315 | ||
The Efficiency of Radix Sort | 316 | ||
Comparing the Algorithms | 316 | ||
Java Interlude 4: More About Exceptions\r | 323 | ||
Programmer-Defined Exception Classes | 323 | ||
Inheritance and Exceptions | 327 | ||
The finally Block | 328 | ||
Chapter 10: Queues, Deques, and Priority Queues\r | 331 | ||
The ADT Queue | 332 | ||
A Problem Solved: Simulating a Waiting Line | 336 | ||
A Problem Solved: Computing the Capital Gain in a Sale of Stock | 342 | ||
Java Class Library: The Interface Queue | 345 | ||
The ADT Deque | 346 | ||
A Problem Solved: Computing the Capital Gain in a Sale of Stock | 349 | ||
Java Class Library: The Interface Deque | 350 | ||
Java Class Library: The Class ArrayDeque | 351 | ||
The ADT Priority Queue | 351 | ||
A Problem Solved: Tracking Your Assignments | 353 | ||
Java Class Library: The Class PriorityQueue | 355 | ||
Chapter 11: Queue, Deque, and Priority Queue Implementations\r | 361 | ||
A Linked Implementation of a Queue | 362 | ||
An Array-Based Implementation of a Queue | 366 | ||
A Circular Array | 366 | ||
A Circular Array with One Unused Location | 369 | ||
Circular Linked Implementations of a Queue | 374 | ||
A Two-Part Circular Linked Chain | 375 | ||
Java Class Library: The Class AbstractQueue | 380 | ||
A Doubly Linked Implementation of a Deque | 381 | ||
Possible Implementations of a Priority Queue | 385 | ||
Chapter 12: Lists\r | 391 | ||
Specifications for the ADT List | 392 | ||
Using the ADT List | 399 | ||
Java Class Library: The Interface List | 403 | ||
Java Class Library: The Class ArrayList | 403 | ||
Chapter 13: A List Implementation That Uses an Array\r | 409 | ||
Using an Array to Implement the ADT List | 410 | ||
An Analogy | 410 | ||
The Java Implementation | 412 | ||
The Efficiency of Using an Array to Implement the ADT List | 420 | ||
Chapter 14: A List Implementation That Links Data\r | 427 | ||
Operations on a Chain of Linked Nodes | 428 | ||
Adding a Node at Various Positions | 428 | ||
Removing a Node from Various Positions | 432 | ||
The Private Method getNodeAt | 433 | ||
Beginning the Implementation | 434 | ||
The Data Fields and Constructor | 435 | ||
Adding to the End of the List | 437 | ||
Adding at a Given Position Within the List | 438 | ||
The Methods isEmpty and toArray | 439 | ||
Testing the Core Methods | 441 | ||
Continuing the Implementation | 442 | ||
A Refined Implementation | 445 | ||
The Tail Reference | 445 | ||
The Efficiency of Using a Chain to Implement the ADT List | 448 | ||
Java Class Library: The Class LinkedList | 450 | ||
Java Interlude 5: Iterators\r | 457 | ||
What Is an Iterator? | 457 | ||
The Interface Iterator | 459 | ||
The Interface Iterable | 461 | ||
Using the Interface Iterator | 461 | ||
Iterable and for-each Loops | 465 | ||
The Interface ListIterator | 466 | ||
The Interface List Revisited | 469 | ||
Using the Interface ListIterator | 470 | ||
Chapter 15: Iterators for the ADT List\r | 473 | ||
Ways to Implement an Iterator | 474 | ||
A Separate Class Iterator | 474 | ||
An Inner Class Iterator | 477 | ||
A Linked Implementation | 478 | ||
An Array-Based Implementation | 481 | ||
Why Are Iterator Methods in Their Own Class? | 484 | ||
An Array-Based Implementation of the Interface ListIterator | 486 | ||
The Inner Class | 487 | ||
Java Interlude 6: Mutable and Immutable Objects\r | 499 | ||
Mutable Objects | 500 | ||
Immutable Objects | 502 | ||
Creating a Read-Only Class | 502 | ||
Companion Classes | 504 | ||
Chapter 16: Sorted Lists\r | 507 | ||
Specifications for the ADT Sorted List | 508 | ||
Using the ADT Sorted List | 511 | ||
A Linked Implementation | 512 | ||
The Method add | 513 | ||
The Efficiency of the Linked Implementation | 520 | ||
An Implementation That Uses the ADT List | 520 | ||
Efficiency Issues | 523 | ||
Java Interlude 7: Inheritance and Polymorphism\r | 529 | ||
Further Aspects of Inheritance | 529 | ||
When to Use Inheritance | 529 | ||
Protected Access | 530 | ||
Abstract Classes and Methods | 531 | ||
Interfaces Versus Abstract Classes | 533 | ||
Polymorphism | 534 | ||
Chapter 17: Inheritance and Lists\r | 541 | ||
Using Inheritance to Implement a Sorted List | 542 | ||
Designing a Base Class | 544 | ||
Creating an Abstract Base Class | 549 | ||
An Efficient Implementation of a Sorted List | 551 | ||
The Method add | 551 | ||
Chapter 18: Searching\r | 557 | ||
The Problem | 558 | ||
Searching an Unsorted Array | 558 | ||
An Iterative Sequential Search of an Unsorted Array | 559 | ||
A Recursive Sequential Search of an Unsorted Array | 560 | ||
The Efficiency of a Sequential Search of an Array | 562 | ||
Searching a Sorted Array | 562 | ||
A Sequential Search of a Sorted Array | 562 | ||
A Binary Search of a Sorted Array | 563 | ||
Java Class Library: The Method binarySearch | 568 | ||
The Efficiency of a Binary Search of an Array | 568 | ||
Searching an Unsorted Chain | 569 | ||
An Iterative Sequential Search of an Unsorted Chain | 570 | ||
A Recursive Sequential Search of an Unsorted Chain | 570 | ||
The Efficiency of a Sequential Search of a Chain | 571 | ||
Searching a Sorted Chain | 571 | ||
A Sequential Search of a Sorted Chain | 571 | ||
A Binary Search of a Sorted Chain | 572 | ||
Choosing a Search Method | 572 | ||
Java Interlude 8: Generics Once Again\r | 579 | ||
More Than One Generic Type | 579 | ||
Chapter 19: Dictionaries\r | 581 | ||
Specifications for the ADT Dictionary | 582 | ||
A Java Interface | 586 | ||
Iterators | 587 | ||
Using the ADT Dictionary | 588 | ||
A Problem Solved: A Directory of Telephone Numbers | 589 | ||
A Problem Solved: The Frequency of Words | 594 | ||
A Problem Solved: A Concordance of Words | 597 | ||
Java Class Library: The Interface Map | 600 | ||
Chapter 20: Dictionary Implementations\r | 605 | ||
Array-Based Implementations | 606 | ||
An Unsorted Array-Based Dictionary | 606 | ||
A Sorted Array-Based Dictionary | 611 | ||
Linked Implementations | 616 | ||
An Unsorted Linked Dictionary | 617 | ||
A Sorted Linked Dictionary | 618 | ||
Chapter 21: Introducing Hashing\r | 625 | ||
What Is Hashing? | 626 | ||
Hash Functions | 629 | ||
Computing Hash Codes | 629 | ||
Compressing a Hash Code into an Index for the Hash Table | 632 | ||
Resolving Collisions | 633 | ||
Open Addressing with Linear Probing | 633 | ||
Open Addressing with Quadratic Probing | 638 | ||
Open Addressing with Double Hashing | 639 | ||
A Potential Problem with Open Addressing | 641 | ||
Separate Chaining | 642 | ||
Chapter 22: Hashing as a Dictionary Implementation\r | 649 | ||
The Efficiency of Hashing | 650 | ||
The Load Factor | 650 | ||
The Cost of Open Addressing | 651 | ||
The Cost of Separate Chaining | 653 | ||
Rehashing | 654 | ||
Comparing Schemes for Collision Resolution | 655 | ||
A Dictionary Implementation That Uses Hashing | 656 | ||
Entries in the Hash Table | 656 | ||
Data Fields and Constructors | 657 | ||
The Methods getValue, remove, and add | 659 | ||
Iterators | 664 | ||
Java Class Library: The Class HashMap | 665 | ||
Jave Class Library: The Class HashSet | 666 | ||
Chapter 23: Trees\r | 669 | ||
Tree Concepts | 670 | ||
Hierarchical Organizations | 670 | ||
Tree Terminology | 672 | ||
Traversals of a Tree | 676 | ||
Traversals of a Binary Tree | 677 | ||
Traversals of a General Tree | 679 | ||
Java Interfaces for Trees | 680 | ||
Interfaces for All Trees | 680 | ||
An Interface for Binary Trees | 681 | ||
Examples of Binary Trees | 682 | ||
Expression Trees | 683 | ||
Decision Trees | 684 | ||
Binary Search Trees | 688 | ||
Heaps | 690 | ||
Examples of General Trees | 693 | ||
Parse Trees | 693 | ||
Game Trees | 693 | ||
Chapter 24: Tree Implementations\r | 703 | ||
The Nodes in a Binary Tree | 704 | ||
A Class of Binary Nodes | 705 | ||
An Implementation of the ADT Binary Tree | 706 | ||
Creating a Basic Binary Tree | 707 | ||
The Method privateSetTree | 708 | ||
Accessor and Mutator Methods | 711 | ||
Computing the Height and Counting Nodes | 711 | ||
Traversals | 712 | ||
An Implementation of an Expression Tree | 717 | ||
General Trees | 718 | ||
A Node for a General Tree | 718 | ||
Using a Binary Tree to Represent a General Tree | 719 | ||
Java Interlude 9: Cloning\r | 727 | ||
Cloneable Objects | 727 | ||
Cloning an Array | 733 | ||
Cloning a Chain | 736 | ||
A Sorted List of Clones | 739 | ||
Cloning a Binary Node | 741 | ||
Chapter 25: A Binary Search Tree Implementation\r | 743 | ||
Getting Started | 744 | ||
An Interface for the Binary Search Tree | 745 | ||
Duplicate Entries | 747 | ||
Beginning the Class Definition | 748 | ||
Searching and Retrieving | 749 | ||
Traversing | 750 | ||
Adding an Entry | 751 | ||
A Recursive Implementation | 752 | ||
An Iterative Implementation | 755 | ||
Removing an Entry | 756 | ||
Removing an Entry Whose Node Is a Leaf | 757 | ||
Removing an Entry Whose Node Has One Child | 757 | ||
Removing an Entry Whose Node Has Two Children | 758 | ||
Removing an Entry in the Root | 761 | ||
A Recursive Implementation | 762 | ||
An Iterative Implementation | 765 | ||
The Efficiency of Operations | 769 | ||
The Importance of Balance | 770 | ||
The Order in Which Nodes Are Added | 770 | ||
An Implementation of the ADT Dictionary | 770 | ||
Chapter 26: A Heap Implementation\r | 783 | ||
Reprise: The ADT Heap | 784 | ||
Using an Array to Represent a Heap | 784 | ||
Adding an Entry | 787 | ||
Removing the Root | 790 | ||
Creating a Heap | 793 | ||
Heap Sort | 796 | ||
Chapter 27: Balanced Search Trees\r | 805 | ||
AVL Trees | 806 | ||
Single Rotations | 806 | ||
Double Rotations | 809 | ||
Implementation Details | 813 | ||
2-3 Trees | 817 | ||
Searching a 2-3 Tree | 818 | ||
Adding Entries to a 2-3 Tree | 819 | ||
Splitting Nodes During Addition | 821 | ||
2-4 Trees | 822 | ||
Adding Entries to a 2-4 Tree | 823 | ||
Comparing AVL, 2-3, and 2-4 Trees | 825 | ||
Red-Black Trees | 826 | ||
Properties of a Red-Black Tree | 827 | ||
Adding Entries to a Red-Black Tree | 828 | ||
Java Class Library: The Class TreeMap | 834 | ||
B-Trees | 834 | ||
Chapter 28: Graphs\r | 841 | ||
Some Examples and Terminology | 842 | ||
Road Maps | 842 | ||
Airline Routes | 845 | ||
Mazes | 845 | ||
Course Prerequisites | 846 | ||
Trees | 846 | ||
Traversals | 847 | ||
Breadth-First Traversal | 848 | ||
Depth-First Traversal | 849 | ||
Topological Order | 851 | ||
Paths | 854 | ||
Finding a Path | 854 | ||
The Shortest Path in an Unweighted Graph | 854 | ||
The Shortest Path in a Weighted Graph | 857 | ||
Java Interfaces for the ADT Graph | 860 | ||
Chapter 29: Graph Implementations\r | 871 | ||
An Overview of Two Implementations | 872 | ||
The Adjacency Matrix | 872 | ||
The Adjacency List | 873 | ||
Vertices and Edges | 874 | ||
Specifying the Class Vertex | 875 | ||
The Inner Class Edge | 877 | ||
Implementing the Class Vertex | 878 | ||
An Implementation of the ADT Graph | 881 | ||
Basic Operations | 881 | ||
Graph Algorithms | 884 | ||
Appendix A: Documentation and Programming Style\r | 891 | ||
Naming Variables and Classes | 891 | ||
Indenting | 892 | ||
Comments | 892 | ||
Single-Line Comments | 893 | ||
Comment Blocks | 893 | ||
When to Write Comments | 893 | ||
Java Documentation Comments | 893 | ||
Appendix B: Java Basics (online)\r | 897 | ||
Introduction | 897 | ||
Applications and Applets | 897 | ||
Objects and Classes | 897 | ||
A First Java Application Program | 897 | ||
Elements of Java | 897 | ||
Identifiers | 897 | ||
Reserved Words | 897 | ||
Variables | 897 | ||
Primitive Types | 897 | ||
Constants | 897 | ||
Assignment Statements | 897 | ||
Assignment Compatibilities | 897 | ||
Type Casting | 897 | ||
Arithmetic Operators and Expressions | 897 | ||
Parentheses and Precedence Rules | 897 | ||
Increment and Decrement Operators | 897 | ||
Special Assignment Operators | 897 | ||
Named Constants | 897 | ||
The Class Math | 897 | ||
Simple Input and Output Using the Keyboard and Screen | 897 | ||
Screen Output | 897 | ||
Keyboard Input Using the Class Scanner | 897 | ||
The if-else Statement | 897 | ||
Boolean Expressions | 897 | ||
Nested Statements | 897 | ||
Multiway if-else Statements | 897 | ||
The Conditional Operator (Optional) | 897 | ||
The switch Statement | 897 | ||
Enumerations | 897 | ||
Scope | 897 | ||
Loops | 897 | ||
The while Statement | 897 | ||
The for Statement | 897 | ||
The do-while Statement | 897 | ||
Additional Loop Information | 897 | ||
The Class String | 897 | ||
Characters Within Strings | 897 | ||
Concatenation of Strings | 897 | ||
String Methods | 897 | ||
The Class StringBuilder | 897 | ||
Using Scanner to Extract Pieces of a String | 897 | ||
Arrays | 897 | ||
Array Parameters and Returned Values | 897 | ||
Initializing Arrays | 897 | ||
Array Index Out of Bounds | 897 | ||
Use of = and == with Arrays | 897 | ||
Arrays and the For-Each Loop | 897 | ||
Multidimensional Arrays | 897 | ||
Wrapper Classes | 897 | ||
Appendix C: Java Classes (online)\r | 898 | ||
Objects and Classes | 898 | ||
Using the Methods in a Java Class | 898 | ||
References and Aliases | 898 | ||
Defining a Java Class | 898 | ||
Method Definitions | 898 | ||
Arguments and Parameters | 898 | ||
Passing Arguments | 898 | ||
A Definition of the Class Name | 898 | ||
Constructors | 898 | ||
The Method toString | 898 | ||
Methods That Call Other Methods | 898 | ||
Methods That Return an Instance of Their Class | 898 | ||
Static Fields and Methods | 898 | ||
Overloading Methods | 898 | ||
Enumeration as a Class | 898 | ||
Packages | 898 | ||
The Java Class Library | 898 | ||
Appendix D: Creating Classes from Other Classes\r | 899 | ||
Composition | 899 | ||
Adapters | 899 | ||
Inheritance | 899 | ||
Invoking Constructors from Within Constructors | 899 | ||
Private Fields and Methods of the Superclass | 899 | ||
Overriding and Overloading Methods | 899 | ||
Multiple Inheritance | 899 | ||
Type Compatibility and Superclasses | 899 | ||
The Class Object | 899 | ||
Appendix E: File Input and Output (online)\r | 917 | ||
Preliminaries | 917 | ||
Why Files? | 917 | ||
Streams | 917 | ||
The Kinds of Files | 917 | ||
File Names | 917 | ||
Text Files | 917 | ||
Creating a Text File | 917 | ||
Reading a Text File | 917 | ||
Changing Existing Data in a Text File | 917 | ||
Defining a Method to Open a Stream | 917 | ||
Binary Files | 917 | ||
Creating a Binary File of Primitive Data | 917 | ||
Reading a Binary File of Primitive Data | 917 | ||
Strings in a Binary File | 917 | ||
Object Serialization | 917 | ||
Glossary (online) | 918 | ||
Index | 919 | ||
A | 919 | ||
B | 920 | ||
C | 920 | ||
D | 921 | ||
E | 921 | ||
F | 922 | ||
G | 922 | ||
H | 922 | ||
I | 923 | ||
J | 923 | ||
K | 923 | ||
L | 924 | ||
M | 924 | ||
N | 924 | ||
O | 924 | ||
P | 925 | ||
Q | 925 | ||
R | 925 | ||
S | 926 | ||
T | 926 | ||
U | 927 | ||
V | 927 | ||
W | 927 |