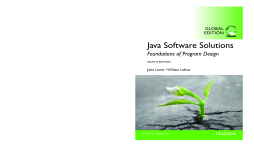
Additional Information
Book Details
Abstract
Intended for use in the Java programming course
Java Software Solutions teaches a foundation of programming techniques to foster well-designed object-oriented software. Heralded for its integration of small and large realistic examples, this worldwide best-selling text emphasizes building solid problem-solving and design skills to write high-quality programs.
Teaching and Learning Experience
To provide a better teaching and learning experience, for both instructors and students, this program will:
- Personalize Learning: Through the power of practice and immediate personalized feedback, MyProgrammingLab helps students fully grasp the logic, semantics, and syntax of programming.
- Help Students Build Sound Program-Development Skills: A software methodology is introduced early and revisited throughout the text to ensure that students build sound program-development skills.
- Enhance Learning with In-text Features: A variety of features in each chapter help motivate learning.
- Provide Opportunities to Practice Design Skills and Implement Java Programs: A wealth of end-of-chapter programming projects and chapter review features help reinforce key concepts.
- Support Instructors and Students: Resources to support learning are available on the Companion website and Instructor Resource Center.
Table of Contents
Section Title | Page | Action | Price |
---|---|---|---|
Cover | Cover | ||
Dedication | 3 | ||
Preface | 5 | ||
Contents | 13 | ||
Credits | 25 | ||
Chapter 1 : Introduction | 27 | ||
1.1 Computer Processing | 28 | ||
Software Categories | 29 | ||
Digital Computers | 31 | ||
Binary Numbers | 33 | ||
1.2 Hardware Components | 36 | ||
Computer Architecture | 37 | ||
Input/Output Devices | 38 | ||
Main Memory and Secondary Memory | 39 | ||
The Central Processing Unit | 43 | ||
1.3 Networks | 46 | ||
Network Connections | 46 | ||
Local-Area Networks and Wide-Area Networks | 48 | ||
The Internet | 49 | ||
The World Wide Web | 50 | ||
Uniform Resource Locators | 51 | ||
1.4 The Java Programming Language | 52 | ||
A Java Program | 54 | ||
Comments | 56 | ||
Identifiers and Reserved Words | 57 | ||
White Space | 60 | ||
1.5 Program Development | 62 | ||
Programming Language Levels | 62 | ||
Editors, Compilers, and Interpreters | 65 | ||
Development Environments | 66 | ||
Syntax and Semantics | 67 | ||
Errors | 68 | ||
1.6 Object-Oriented Programming | 70 | ||
Problem Solving | 71 | ||
Object-Oriented Software Principles | 72 | ||
Summary of Key Concepts | 76 | ||
Exercises | 78 | ||
Programming Projects | 80 | ||
Chapter 2 : Data and Expressions | 83 | ||
2.1 Character Strings | 84 | ||
The print and println Methods | 84 | ||
String Concatenation | 86 | ||
Escape Sequences | 89 | ||
2.2 Variables and Assignment | 91 | ||
Variables | 91 | ||
The Assignment Statement | 93 | ||
Constants | 95 | ||
2.3 Primitive Data Types | 97 | ||
Integers and Floating Points | 97 | ||
Characters | 99 | ||
Booleans | 100 | ||
2.4 Expressions | 101 | ||
Arithmetic Operators | 101 | ||
Operator Precedence | 102 | ||
Increment and Decrement Operators | 106 | ||
Assignment Operators | 107 | ||
2.5 Data Conversion | 109 | ||
Conversion Techniques | 111 | ||
2.6 Interactive Programs | 113 | ||
The Scanner Class | 113 | ||
2.7 Graphics | 118 | ||
Coordinate Systems | 118 | ||
Representing Color | 120 | ||
2.8 Applets | 121 | ||
Executing Applets Using the Web | 124 | ||
2.9 Drawing Shapes | 125 | ||
The Graphics Class | 125 | ||
Summary of Key Concepts | 131 | ||
Exercises | 132 | ||
Programming Projects | 134 | ||
Software Failure : NASA Mars Climate Orbiter and Polar Lander | 137 | ||
What Happened? | 137 | ||
What Caused It? | 137 | ||
Lessons Learned | 138 | ||
Chapter 3 : Using Classes and Objects | 139 | ||
3.1 Creating Objects | 140 | ||
Aliases | 142 | ||
3.2 The String Class | 144 | ||
3.3 Packages | 148 | ||
The import Declaration | 150 | ||
3.4 The Random Class | 152 | ||
3.5 The Math Class | 155 | ||
3.6 Formatting Output | 158 | ||
The NumberFormat Class | 158 | ||
The DecimalFormat Class | 160 | ||
The printf Method | 161 | ||
3.7 Enumerated Types | 164 | ||
3.8 Wrapper Classes | 167 | ||
Autoboxing | 169 | ||
3.9 Components and Containers | 169 | ||
Frames and Panels | 170 | ||
3.10 Nested Panels | 174 | ||
3.11 Images | 177 | ||
Summary of Key Concepts | 181 | ||
Exercises | 181 | ||
Programming Projects | 183 | ||
Chapter 4 : Writing Classes | 185 | ||
4.1 Classes and Objects Revisited | 186 | ||
4.2 Anatomy of a Class | 188 | ||
Instance Data | 193 | ||
UMl Class Diagrams | 193 | ||
4.3 Encapsulation | 195 | ||
Visibility Modifiers | 196 | ||
Accessors and Mutators | 197 | ||
4.4 Anatomy of a Method | 198 | ||
The return Statement | 200 | ||
Parameters | 201 | ||
Local Data | 201 | ||
Bank Account Example | 202 | ||
4.5 Constructors Revisited | 207 | ||
4.6 Graphical Objects | 208 | ||
4.7 Graphical User Interfaces | 217 | ||
4.8 Buttons | 218 | ||
4.9 Text Fields | 222 | ||
Summary of Key Concepts | 226 | ||
Exercises | 226 | ||
Programming Projects | 228 | ||
Software Failure : Denver Airport Baggage Handling System | 231 | ||
What Happened? | 231 | ||
What Caused It? | 232 | ||
Lessons Learned | 232 | ||
Chapter 5 : Conditionals and Loops | 233 | ||
5.1 Boolean Expressions | 234 | ||
Equality and Relational Operators | 235 | ||
Logical Operators | 236 | ||
5.2 The if Statement | 239 | ||
The if-else Statement | 242 | ||
Using Block Statements | 245 | ||
Nested if Statements | 249 | ||
5.3 Comparing Data | 252 | ||
Comparing Floats | 252 | ||
Comparing Characters | 253 | ||
Comparing Objects | 254 | ||
5.4 The while Statement | 256 | ||
Infinite Loops | 260 | ||
Nested Loops | 262 | ||
The break and Continue Statements | 265 | ||
5.5 Iterators | 267 | ||
Reading Text Files | 268 | ||
5.6 The ArrayList Class | 271 | ||
5.7 Determining Event Sources | 274 | ||
5.8 Check Boxes and Radio Buttons | 277 | ||
Check Boxes | 277 | ||
Radio Buttons | 281 | ||
Summary of Key Concepts | 286 | ||
Exercises | 286 | ||
Programming Projects | 289 | ||
Software Failure : Therac-25 | 293 | ||
What Happened? | 293 | ||
What Caused It? | 293 | ||
Lessons Learned | 294 | ||
Chapter 6 : More Conditionals and Loops | 295 | ||
6.1 The switch Statement | 296 | ||
6.2 The Conditional Operator | 300 | ||
6.3 The do Statement | 301 | ||
6.4 The for Statement | 305 | ||
The for-each Loop | 308 | ||
Comparing Loops | 310 | ||
6.5 Drawing with Loops and Conditionals | 311 | ||
6.6 Dialog Boxes | 317 | ||
Summary of Key Concepts | 320 | ||
Exercises | 320 | ||
Programming Projects | 322 | ||
Chapter 7 : Object-Oriented Design | 327 | ||
7.1 Software Development Activities | 328 | ||
7.2 Identifying Classes and Objects | 329 | ||
Assigning Responsibilities | 331 | ||
7.3 Static Class Members | 331 | ||
Static Variables | 332 | ||
Static Methods | 332 | ||
7.4 Class Relationships | 336 | ||
Dependency | 336 | ||
Dependencies Among Objects of the Same Class | 336 | ||
Aggregation | 342 | ||
The this Reference | 346 | ||
7.5 Interfaces | 348 | ||
The Comparable Interface | 353 | ||
The Iterator Interface | 354 | ||
7.6 Enumerated Types Revisited | 355 | ||
7.7 Method Design | 358 | ||
Method Decomposition | 359 | ||
Method Parameters Revisited | 364 | ||
7.8 Method Overloading | 369 | ||
7.9 Testing | 371 | ||
Reviews | 372 | ||
Defect Testing | 372 | ||
7.10 GUI Design | 375 | ||
7.11 Layout Managers | 376 | ||
Flow Layout | 378 | ||
Border Layout | 382 | ||
Grid Layout | 385 | ||
Box Layout | 387 | ||
7.12 Borders | 391 | ||
7.13 Containment Hierarchies | 395 | ||
Summary of Key Concepts | 398 | ||
Exercises | 399 | ||
Programming Projects | 400 | ||
Software Failure : 2003 Northeast Blackout | 403 | ||
What Happened? | 403 | ||
What Caused It? | 403 | ||
Lessons Learned | 404 | ||
Chapter 8 : Arrays | 405 | ||
8.1 Array Elements | 406 | ||
8.2 Declaring and Using Arrays | 407 | ||
Bounds Checking | 410 | ||
Alternate Array Syntax | 415 | ||
Initializer Lists | 415 | ||
Arrays as Parameters | 416 | ||
8.3 Arrays of Objects | 418 | ||
8.4 Command-Line Arguments | 428 | ||
8.5 Variable Length Parameter Lists | 430 | ||
8.6 Two-Dimensional Arrays | 434 | ||
Multidimensional Arrays | 438 | ||
8.7 Polygons and Polylines | 439 | ||
The Polygon Class | 442 | ||
8.8 Mouse Events | 444 | ||
8.9 Key Events | 453 | ||
Summary of Key Concepts | 459 | ||
Exercises | 459 | ||
Programming Projects | 461 | ||
Software Failure : LA Air Traffic Control | 467 | ||
What Happened? | 467 | ||
What Caused It? | 467 | ||
Lessons Learned | 468 | ||
Chapter 9 : Inheritance | 469 | ||
9.1 Creating Subclasses | 470 | ||
The protected Modifier | 473 | ||
The super Reference | 476 | ||
Multiple Inheritance | 479 | ||
9.2 Overriding Methods | 481 | ||
Shadowing Variables | 483 | ||
9.3 Class Hierarchies | 484 | ||
The Object Class | 486 | ||
Abstract Classes | 487 | ||
Interface Hierarchies | 489 | ||
9.4 Visibility | 489 | ||
9.5 Designing for Inheritance | 492 | ||
Restricting Inheritance | 493 | ||
9.6 The Component Class Hierarchy | 494 | ||
9.7 Extending Adapter Classes | 497 | ||
9.8 The Timer Class | 501 | ||
Summary of Key Concepts | 506 | ||
Exercises | 507 | ||
Programming Projects | 508 | ||
Software Failure : Ariane 5 Flight 501 | 511 | ||
What Happened? | 511 | ||
What Caused It? | 511 | ||
Lessons Learned | 511 | ||
Chapter 10 : Polymorphism | 513 | ||
10.1 Late Binding | 514 | ||
10.2 Polymorphism via Inheritance | 515 | ||
10.3 Polymorphism via Interfaces | 528 | ||
10.4 Sorting | 530 | ||
Selection Sort | 531 | ||
Insertion Sort | 537 | ||
Comparing Sorts | 538 | ||
10.5 Searching | 539 | ||
Linear Search | 539 | ||
Binary Search | 541 | ||
Comparing Searches | 545 | ||
10.6 Designing for Polymorphism | 545 | ||
10.7 Event Processing | 547 | ||
10.8 File Choosers | 548 | ||
10.9 Color Choosers | 551 | ||
10.10 Sliders | 553 | ||
Summary of Key Concepts | 559 | ||
Exercises | 559 | ||
Programming Projects | 560 | ||
Chapter 11 : Exceptions | 563 | ||
11.1 Exception Handling | 564 | ||
11.2 Uncaught Exceptions | 565 | ||
11.3 The try-catch Statement | 566 | ||
The finally Clause | 570 | ||
11.4 Exception Propagation | 571 | ||
11.5 The Exception Class Hierarchy | 575 | ||
Checked and Unchecked Exceptions | 578 | ||
11.6 I/O Exceptions | 579 | ||
11.7 Tool Tips and Mnemonics | 583 | ||
11.8 Combo Boxes | 590 | ||
11.9 Scroll Panes | 595 | ||
11.10 Split Panes | 598 | ||
Summary of Key Concepts | 605 | ||
Exercises | 606 | ||
Programming Projects | 606 | ||
Chapter 12 : Recursion | 609 | ||
12.1 Recursive Thinking | 610 | ||
Infinite Recursion | 610 | ||
Recursion in Math | 611 | ||
12.2 Recursive Programming | 612 | ||
Recursion vs. Iteration | 615 | ||
Direct vs. Indirect Recursion | 615 | ||
12.3 Using Recursion | 616 | ||
Traversing a Maze | 617 | ||
The Towers of Hanoi | 622 | ||
12.4 Recursion in Graphics | 627 | ||
Tiled Pictures | 627 | ||
Fractals | 630 | ||
Summary of Key Concepts | 638 | ||
Exercises | 638 | ||
Programming Projects | 639 | ||
Chapter 13 : Collections | 643 | ||
13.1 Collections and Data Structures | 644 | ||
Separating Interface from Implementation | 644 | ||
13.2 Dynamic Representations | 645 | ||
Dynamic Structures | 645 | ||
A Dynamically Linked List | 646 | ||
Other Dynamic List Representations | 651 | ||
13.3 Linear Data Structures | 653 | ||
Queues | 653 | ||
Stacks | 654 | ||
13.4 Non-Linear Data Structures | 657 | ||
Trees | 657 | ||
Graphs | 658 | ||
13.5 The Java Collections API | 660 | ||
Generics | 660 | ||
Summary of Key Concepts | 662 | ||
Exercises | 662 | ||
Programming Projects | 664 | ||
Appendix | 667 | ||
Appendix A : Glossary | 667 | ||
Appendix B : Number Systems | 691 | ||
Place Value | 691 | ||
Bases Higher Than 10 | 693 | ||
Conversions | 695 | ||
Shortcut Conversions | 696 | ||
Appendix C : The Unicode Character Set | 699 | ||
Appendix D : Java Operators | 703 | ||
Java Bitwise Operators | 703 | ||
Appendix E : Java Modifiers | 709 | ||
Java Visibility Modifiers | 709 | ||
A Visibility Example | 710 | ||
Other Java Modifiers | 711 | ||
Appendix F : Java Coding Guidelines | 713 | ||
Design Guidelines | 714 | ||
Style Guidelines | 715 | ||
Documentation Guidelines | 716 | ||
Appendix G : Java Applets | 719 | ||
Appendix H : Regular Expressions | 721 | ||
Appendix I : Javadoc Documentation Generator | 723 | ||
Doc Comments | 724 | ||
Tags | 724 | ||
Files Generated | 726 | ||
Appendix J : The PaintBox Project | 729 | ||
PaintBox Requirements | 729 | ||
PaintBox Architectural Design | 731 | ||
PaintBox Refinements | 732 | ||
PaintBox Refinement | 732 | ||
PaintBox Refinement | 735 | ||
Remaining PaintBox Refinements | 737 | ||
Appendix K : GUI Events | 741 | ||
Appendix L : Java Syntax | 745 | ||
Appendix M : The Java Class Library | 759 | ||
Appendix N : Answers to Self-Review Questions | 761 | ||
Chapter 1 : Introduction | 761 | ||
1.1 Computer Processing | 761 | ||
1.2 Hardware Components | 761 | ||
1.3 Networks | 762 | ||
1.4 The Java Programming Language | 763 | ||
1.5 Program Development | 764 | ||
1.6 Object-Oriented Programming | 765 | ||
Chapter 2 : Data and Expressions | 765 | ||
2.1 Character Strings | 765 | ||
2.2 Variables and Assignment | 766 | ||
2.3 Primitive Data Types | 767 | ||
2.4 Expressions | 767 | ||
2.5 Data Conversion | 768 | ||
2.6 Interactive Programs | 769 | ||
2.7 Graphics | 769 | ||
2.8 Applets | 770 | ||
2.9 Drawing Shapes | 770 | ||
Chapter 3 : Using Classes and Objects | 771 | ||
3.1 Creating Objects | 771 | ||
3.2 The String Class | 771 | ||
3.3 Packages | 772 | ||
3.4 The Random Class | 772 | ||
3.5 The Math Class | 773 | ||
3.6 Formatting Output | 773 | ||
3.7 Enumerated Types | 774 | ||
3.8 Wrapper Classes | 775 | ||
3.9 Components and Containers | 775 | ||
3.10 Nested Panels | 776 | ||
3.11 Images | 776 | ||
Chapter 4 : Writing Classes | 777 | ||
4.1 Classes and Objects Revisited | 777 | ||
4.2 Anatomy of a Class | 778 | ||
4.3 Encapsulation | 778 | ||
4.4 Anatomy of a Method | 779 | ||
4.5 Constructors Revisited | 780 | ||
4.6 Graphical Objects | 780 | ||
4.7 Graphical User Interfaces | 781 | ||
4.8 Buttons | 781 | ||
4.9 Text Fields | 782 | ||
Chapter 5 : Conditionals and Loops | 782 | ||
5.1 Boolean Expressions | 782 | ||
5.2 The if Statement | 783 | ||
5.3 Comparing Data | 784 | ||
5.4 The while Statement | 785 | ||
5.5 Iterators | 786 | ||
5.6 The ArrayList Class | 786 | ||
Chapter 6 : More Conditionals and Loops | 787 | ||
6.1 The switch Statement | 787 | ||
6.2 The Conditional Operator | 787 | ||
6.3 The do Statement | 788 | ||
6.4 The for Statement | 788 | ||
Chapter 7 : Object-Oriented Design | 789 | ||
7.1 Software Development Activities | 789 | ||
7.2 Identifying Classes and Objects | 789 | ||
7.3 Static Class Members | 790 | ||
7.4 Class Relationships | 790 | ||
7.5 Interfaces | 790 | ||
7.6 Enumerated Types Revisited | 791 | ||
7.7 Method Design | 791 | ||
7.8 Method Overloading | 792 | ||
7.9 Testing | 792 | ||
7.10 GUI Design | 792 | ||
7.11 Layout Managers | 793 | ||
7.12 Borders | 793 | ||
7.13 Containment Hierarchies | 793 | ||
Chapter 8 : Arrays | 794 | ||
8.1 Array Elements | 794 | ||
8.2 Declaring and Using Arrays | 794 | ||
8.3 Arrays of Objects | 795 | ||
8.4 Command-Line Arguments | 796 | ||
8.5 Variable Length Parameter Lists | 796 | ||
8.6 Two-Dimensional Arrays | 797 | ||
8.7 Polygons and Polylines | 797 | ||
8.8 Mouse Events | 798 | ||
8.9 Key Events | 798 | ||
Chapter 9 : Inheritance | 799 | ||
9.1 Creating Subclasses | 799 | ||
9.2 Overriding Methods | 800 | ||
9.3 Class Hierarchies | 800 | ||
9.4 Visibility | 801 | ||
9.5 Designing for Inheritance | 801 | ||
9.6 The Component Class Hierarchy | 802 | ||
9.7 Extending Adapter Classes | 802 | ||
9.8 The Timer Class | 802 | ||
Chapter 10 : Polymorphism | 803 | ||
10.1 Late Binding | 803 | ||
10.2 Polymorphism via Inheritance | 803 | ||
10.3 Polymorphism via Interfaces | 804 | ||
10.4 Sorting | 804 | ||
10.5 Searching | 805 | ||
10.6 Designing for Polymorphism | 806 | ||
Chapter 11 : Exceptions | 806 | ||
11.1 Exception Handling | 806 | ||
11.2 Uncaught Exceptions | 806 | ||
11.3 The try-catch Statement | 806 | ||
11.4 Exception Propagation | 807 | ||
11.5 The Exception Class Hierarchy | 807 | ||
11.6 I/O Exceptions | 808 | ||
11.7 Tool Tips and Mnemonics | 808 | ||
11.8 Combo Boxes | 809 | ||
11.9 Scroll Panes | 809 | ||
11.10 Split Panes | 810 | ||
Chapter 12 : Recursion | 810 | ||
12.1 Recursive Thinking | 810 | ||
12.2 Recursive Programming | 810 | ||
12.3 Using Recursion | 811 | ||
12.4 Recursion in Graphics | 812 | ||
Chapter 13 : Collections | 812 | ||
13.1 Collections and Data Structures | 812 | ||
13.2 Dynamic Representations | 813 | ||
13.3 Linear Data Structures | 813 | ||
13.4 Non-Linear Data Structures | 814 | ||
13.5 The Java Collections API | 814 | ||
Index | 815 |